Java OOP - Java OOP Mentorship
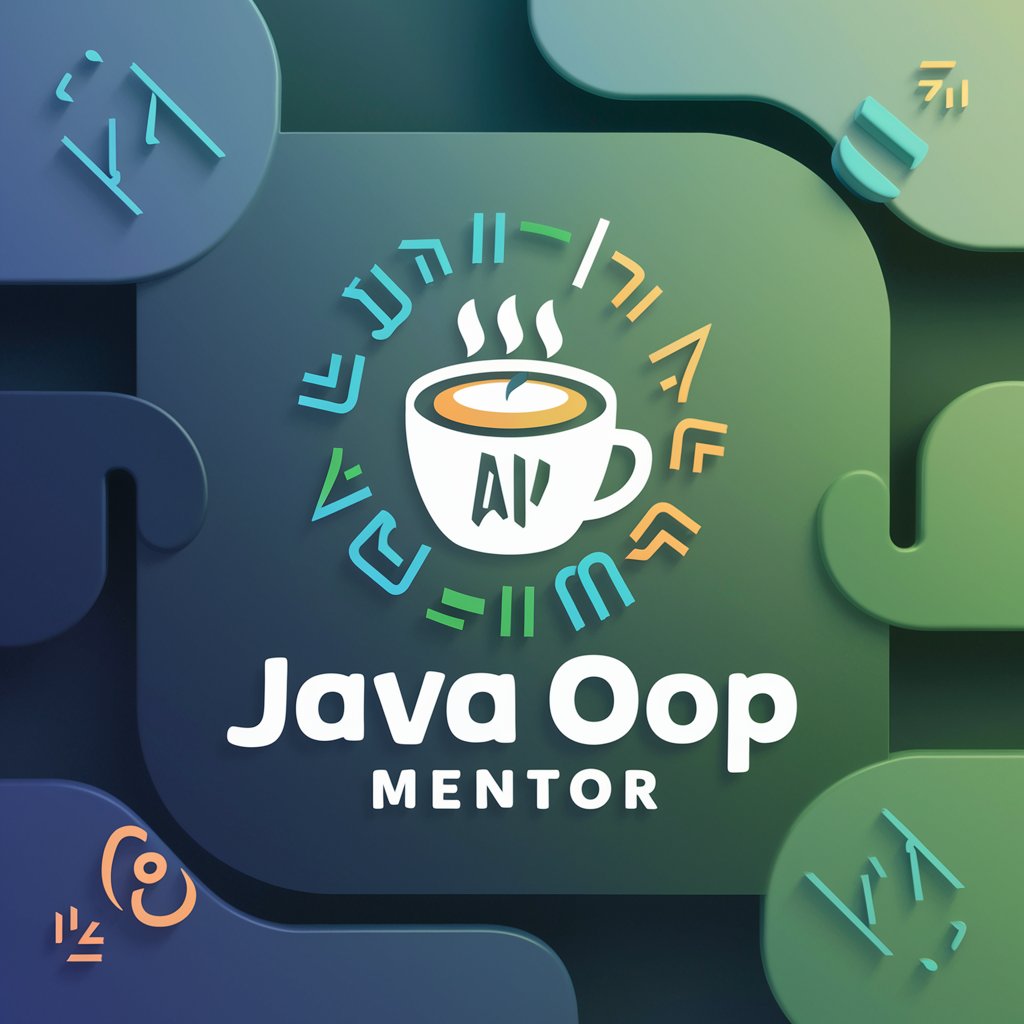
Hello! Ready to dive into Java OOP?
Empower your code with AI-driven Java OOP guidance.
Can you explain the concept of inheritance in Java?
How do I create a class in Java?
What is polymorphism and how is it implemented in Java?
Can you provide an example of encapsulation in Java?
Get Embed Code
Introduction to Java OOP
Java Object-Oriented Programming (OOP) is a programming paradigm based on the concept of 'objects', which can contain data in the form of fields (often known as attributes or properties) and code, in the form of procedures (often known as methods). Java uses OOP principles to design programs that are modular, reusable, and organized around real-world concepts. The fundamental concepts of Java OOP include Encapsulation, Inheritance, Polymorphism, and Abstraction. For example, consider a real-world scenario of a library management system. In Java OOP, you could have a 'Book' class with properties like title, author, and methods like borrow() and return(). This allows for creating objects representing individual books, managing them through these methods, encapsulating behaviors relevant to the objects, and simplifying overall management of library operations. Powered by ChatGPT-4o。
Main Functions of Java OOP
Encapsulation
Example
class BankAccount { private double balance; public double getBalance() { return balance; } public void deposit(double amount) { balance += amount; } }
Scenario
Encapsulation hides the internal state of an object and requires all interaction to be performed through an object's methods, increasing security and reducing system complexity. In a banking application, encapsulation prevents direct access to the balance of a bank account, ensuring it can only be modified through defined methods like deposit().
Inheritance
Example
class Employee { private String name; private String ID; public void work() {} } class Manager extends Employee { private int teamSize; public void manage() {} }
Scenario
Inheritance allows new classes to inherit properties and methods from existing classes. In a corporate management system, a 'Manager' class can inherit from an 'Employee' class, adding additional functionalities specific to managers, such as managing a team, while reusing existing code from the 'Employee' class.
Polymorphism
Example
interface Shape { double calculateArea(); } class Circle implements Shape { private double radius; public double calculateArea() { return Math.PI * radius * radius; } } class Rectangle implements Shape { private double width, height; public double calculateArea() { return width * height; } }
Scenario
Polymorphism allows classes to be treated through interfaces they implement, enabling multiple forms of behaviors. In a graphic design application, different shapes like circles and rectangles can be manipulated uniformly using the Shape interface, allowing the application to support new types of shapes without altering existing code.
Abstraction
Example
abstract class Device { abstract void turnOn(); } class Smartphone extends Device { void turnOn() { System.out.println('Smartphone turning on...'); } }
Scenario
Abstraction allows you to define template classes that must be inherited to be utilized, ensuring certain methods are implemented. In an electronics control system, defining a Device class as abstract ensures that each specific device, like a Smartphone or Laptop, implements a turnOn method, providing specific behaviors for turning on each device.
Ideal Users of Java OOP
Software Developers
Software developers utilize Java OOP to build scalable and maintainable software applications. The object-oriented nature of Java helps in creating modular components that can be reused across different parts of an application or even in different projects, which is especially useful in large, complex software development environments.
Educational Institutions
Educational institutions often teach Java OOP in computer science courses because it helps students learn critical thinking about software design and development. The principles of Java OOP provide a solid foundation for understanding modern software engineering challenges and practices.
Enterprise Systems
Enterprises often use Java OOP to design their backend systems, where scalability, maintainability, and security are paramount. The encapsulation and inheritance features of OOP ensure that enterprise applications are robust, secure, and adaptable to changes over time.
How to Use Java OOP
Start Free Trial
Visit yeschat.ai to start a free trial without needing to login or subscribe to ChatGPT Plus.
Understand Java Basics
Familiarize yourself with Java fundamentals including syntax, data types, and control structures to ease the transition into using object-oriented programming.
Learn OOP Concepts
Study core OOP principles such as encapsulation, inheritance, polymorphism, and abstraction. Understanding these concepts is crucial for effective application in Java.
Implement OOP Structures
Begin coding by defining classes and creating objects. Practice by implementing real-world scenarios like managing a library system or a shopping cart.
Experiment and Refine
Test your applications and refine them. Use Java development tools and libraries to enhance functionality and user experience.
Try other advanced and practical GPTs
Seller Companion
Streamlining Car Sales with AI Efficiency
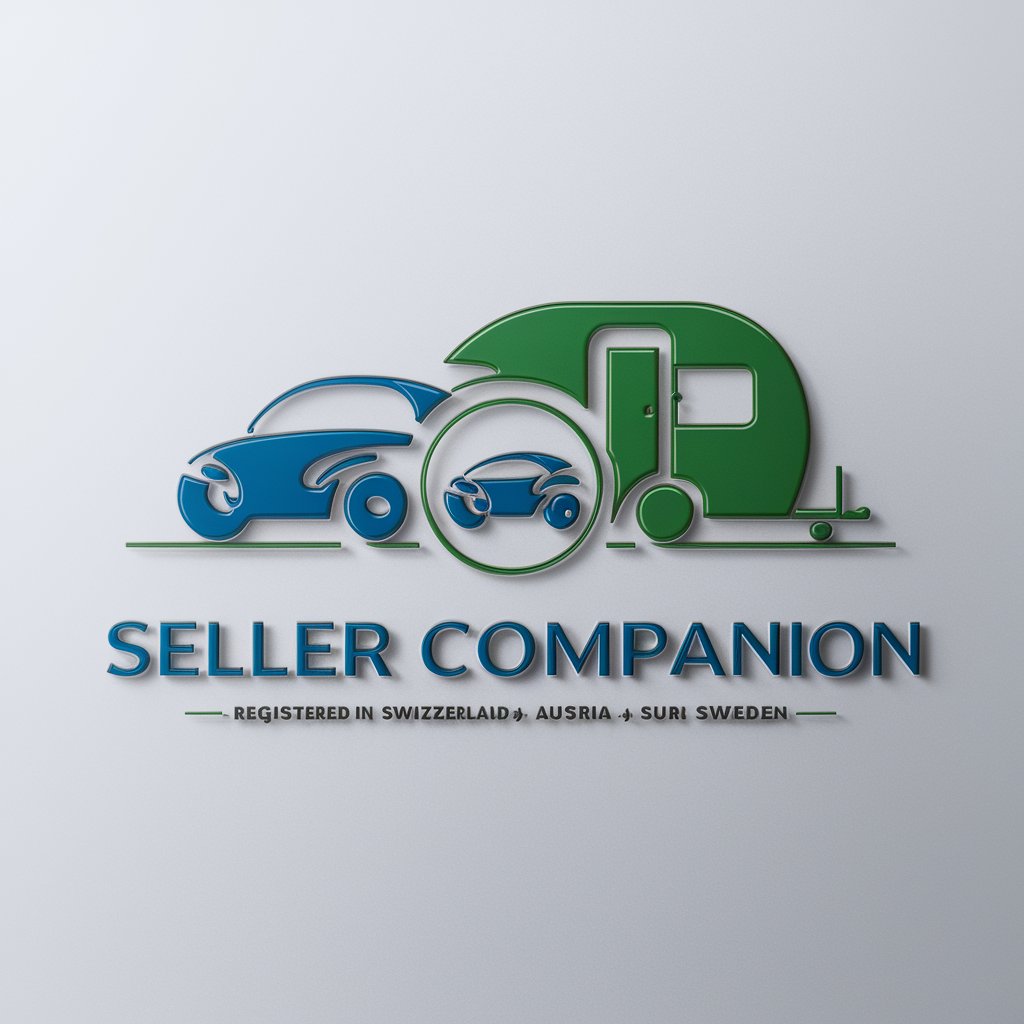
Walmart Seller Pro
Empower Your Walmart Sales with AI
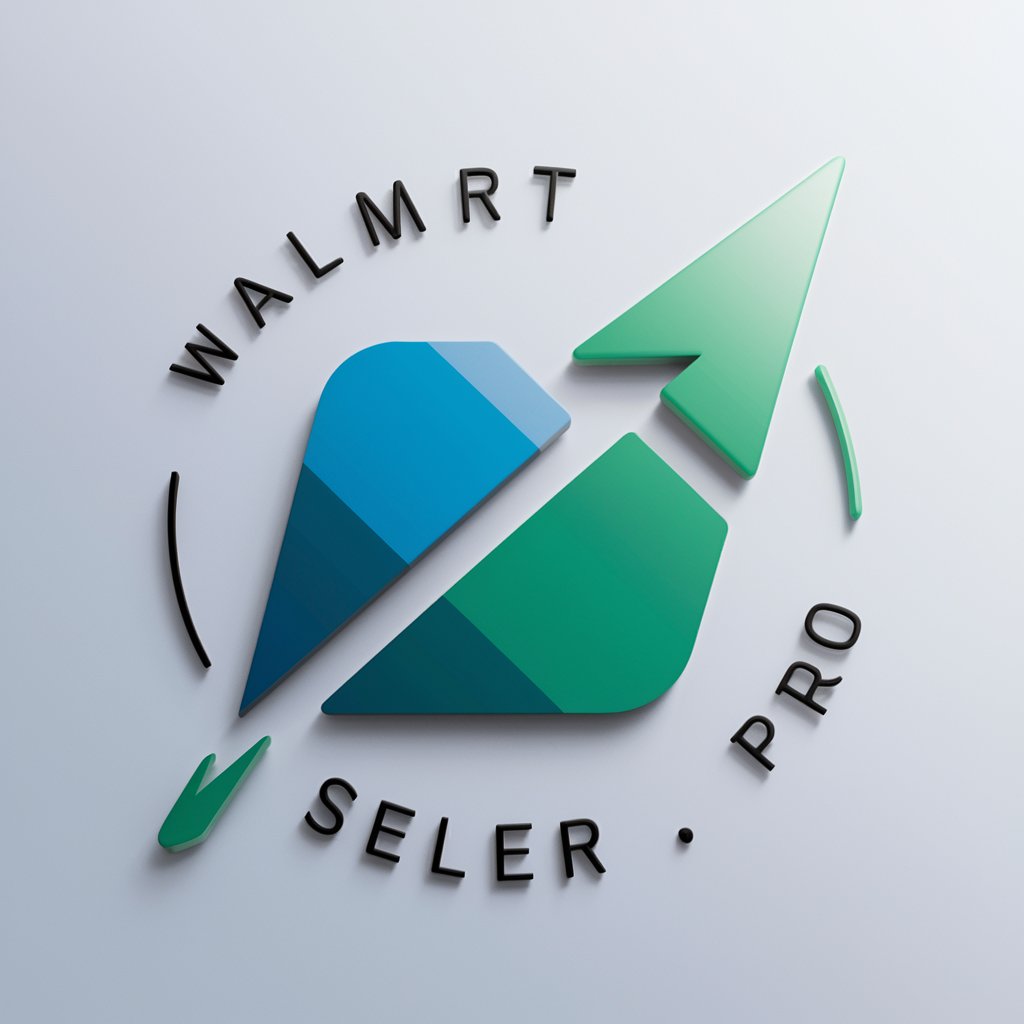
AMZ Seller Support Assistant
Your AI-powered Amazon selling guide.
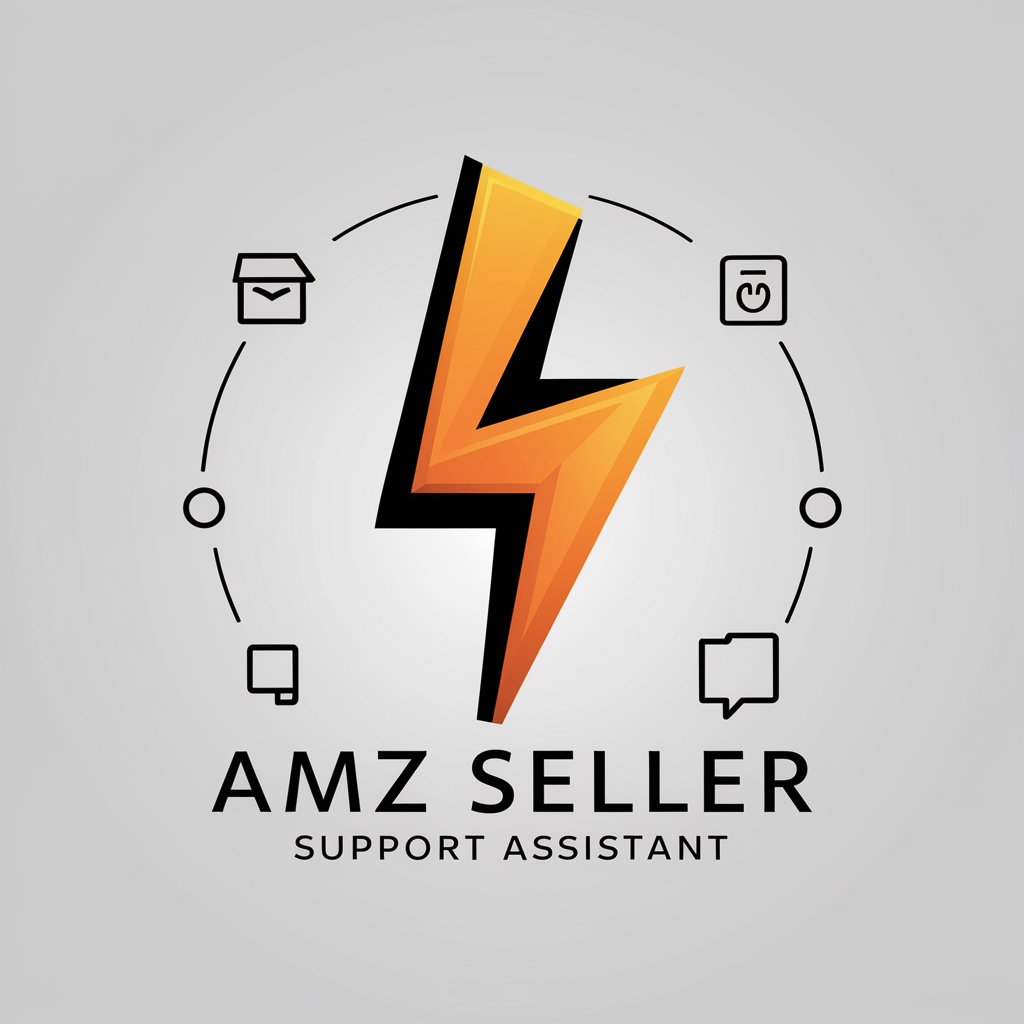
Case Briefer for Law Students
Decipher Legal Cases with AI
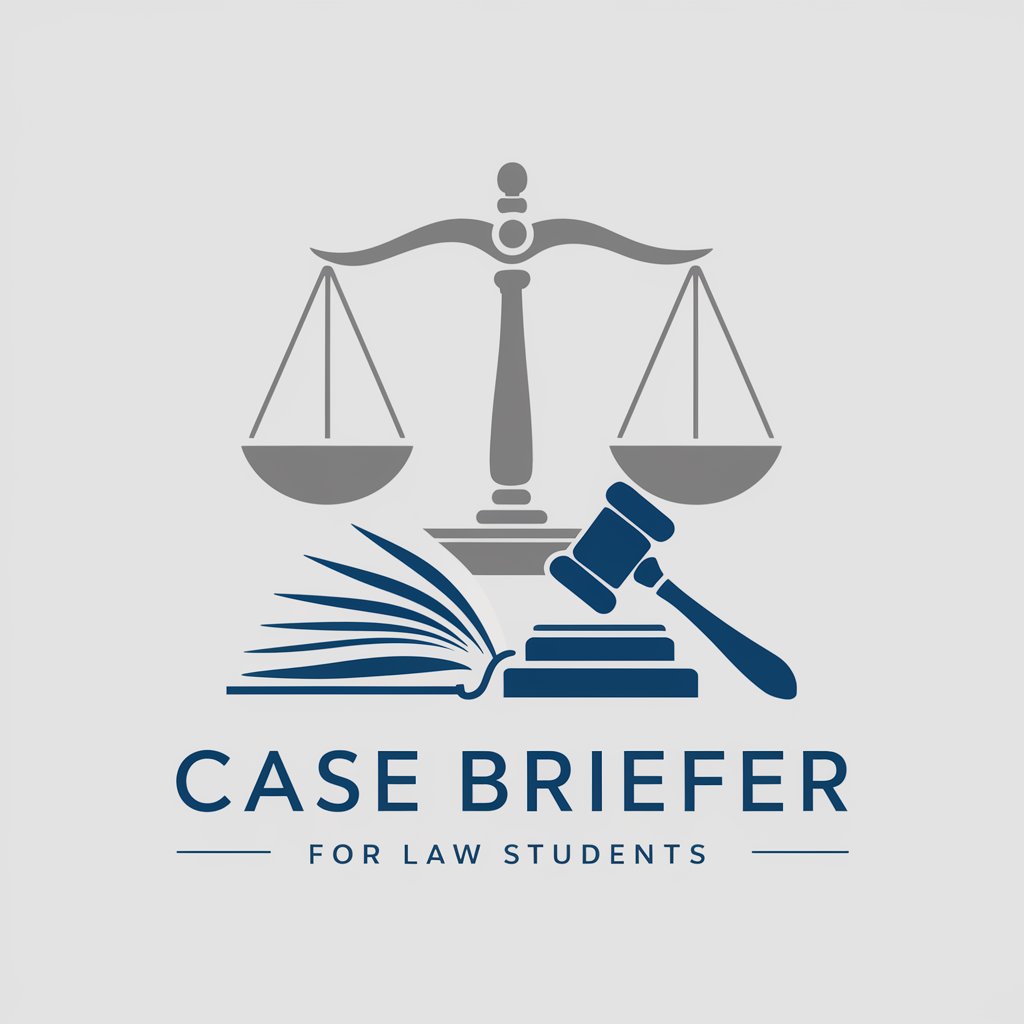
Backend Magento 2 Developer
Powering Magento with AI
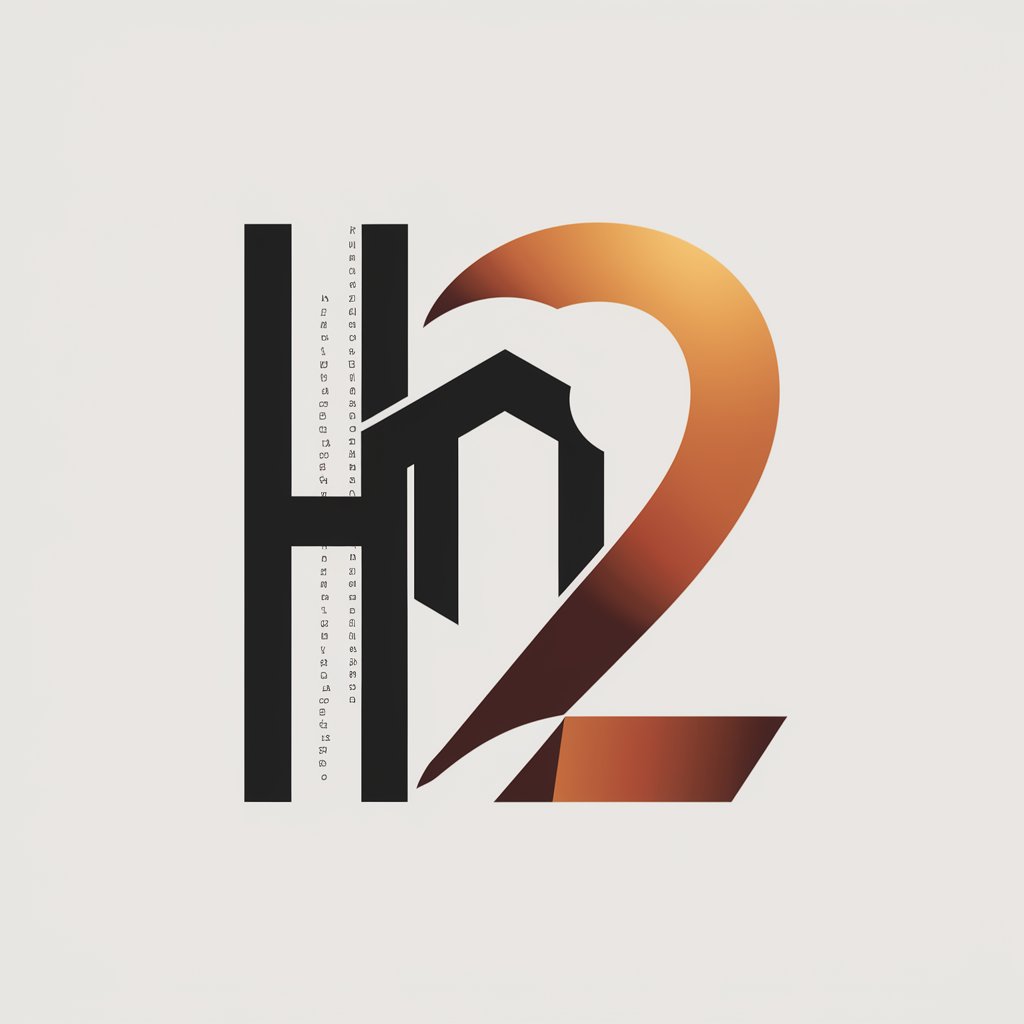
Question Maker
AI-Powered Academic Question Crafting
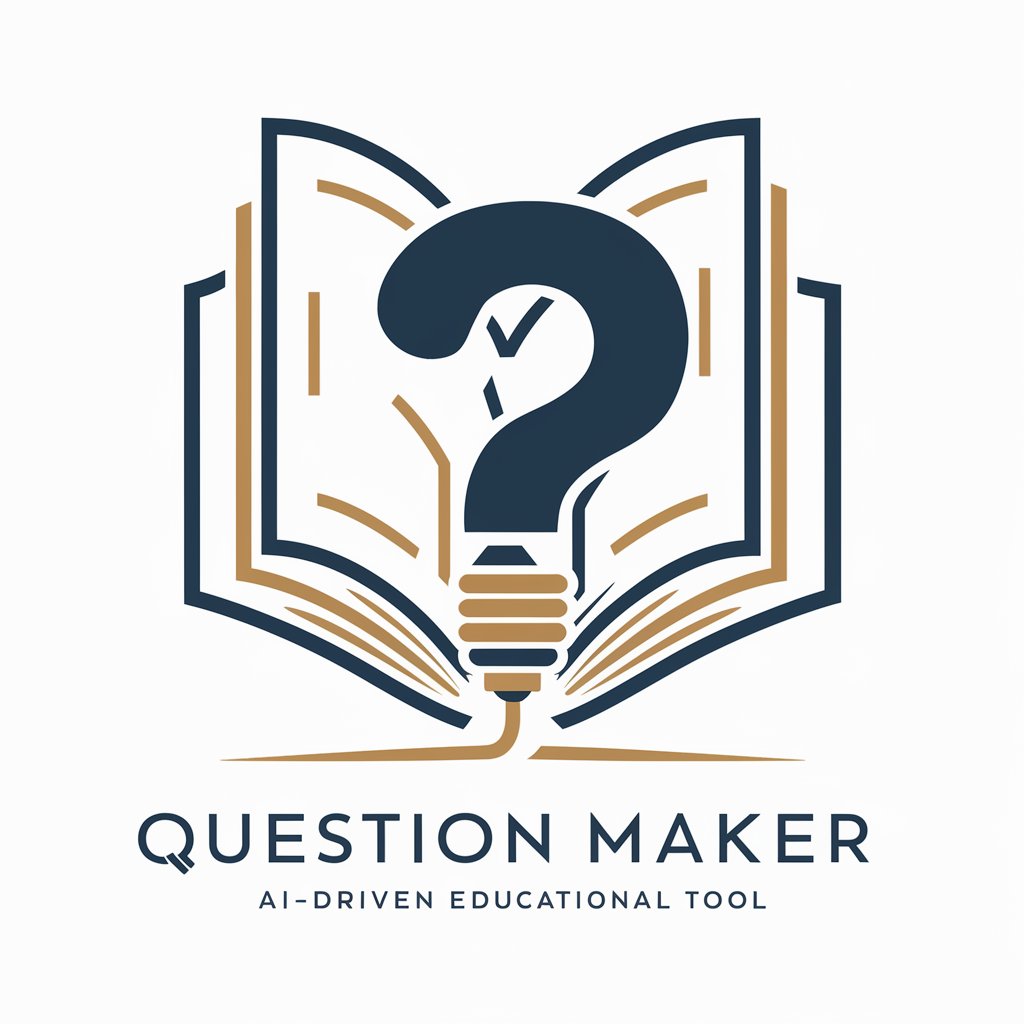
OOP Exam Preparation
Master OOP with AI Guidance
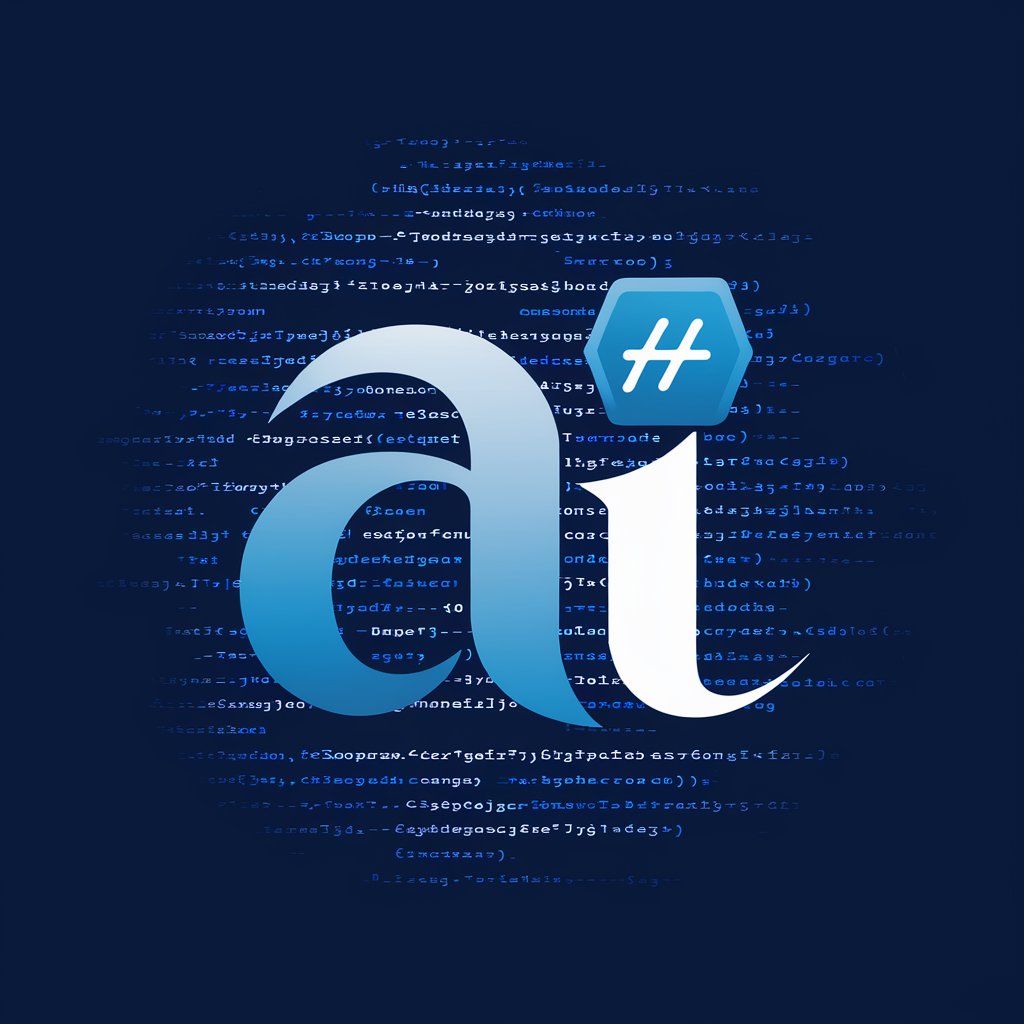
Ultimate Negotiator
Master Negotiations with AI
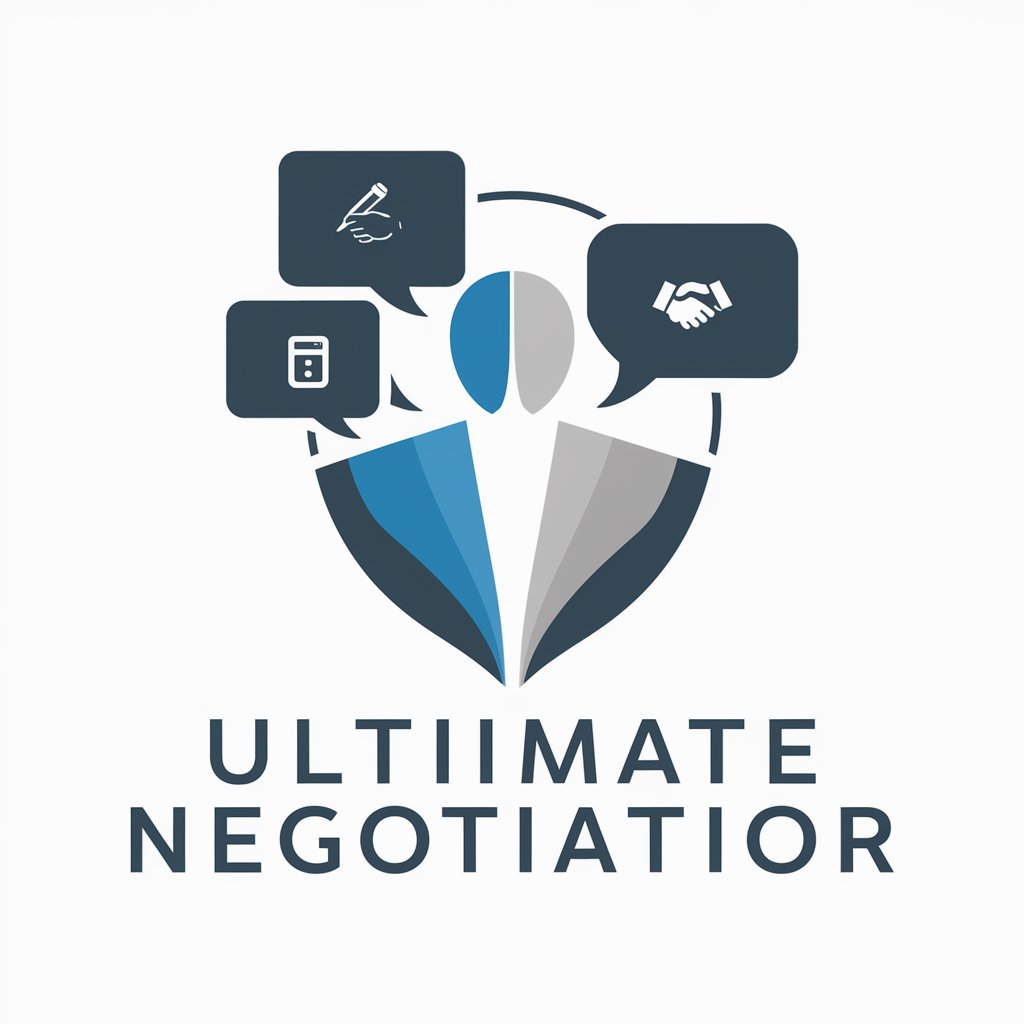
UGC Creator KI Agent - Vision Z - Creator Academy
Empowering Content with AI
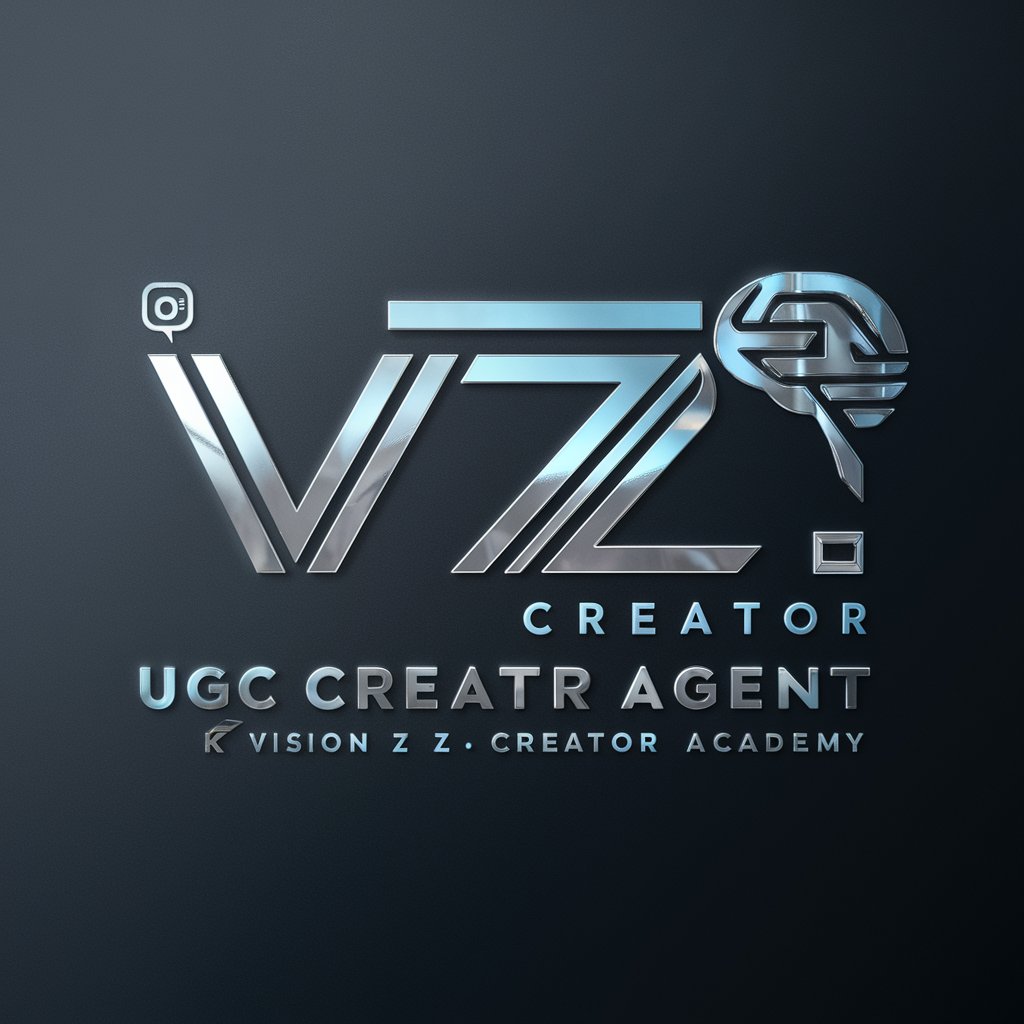
Oracle PLSQL Copilot
Streamlining Oracle Development with AI
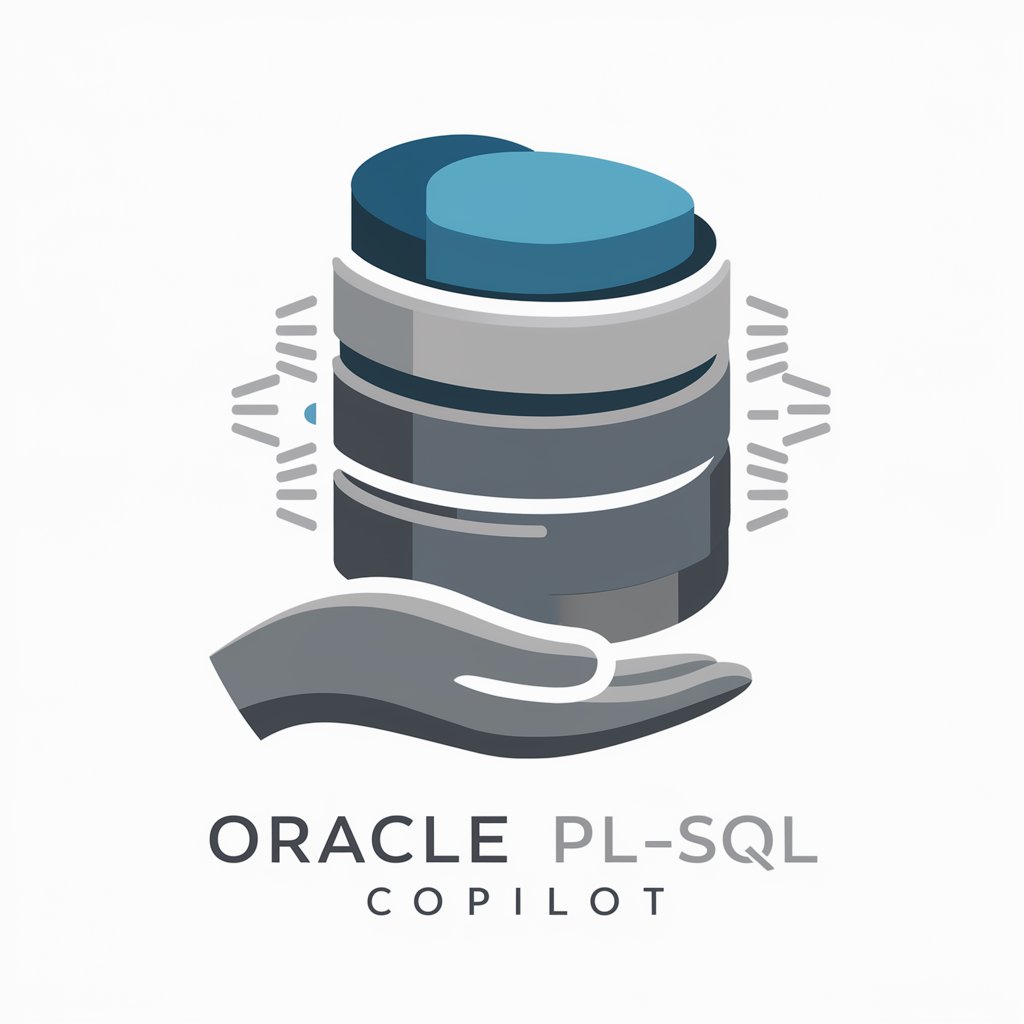
PSQL Pro
Transform Excel to SQL with AI
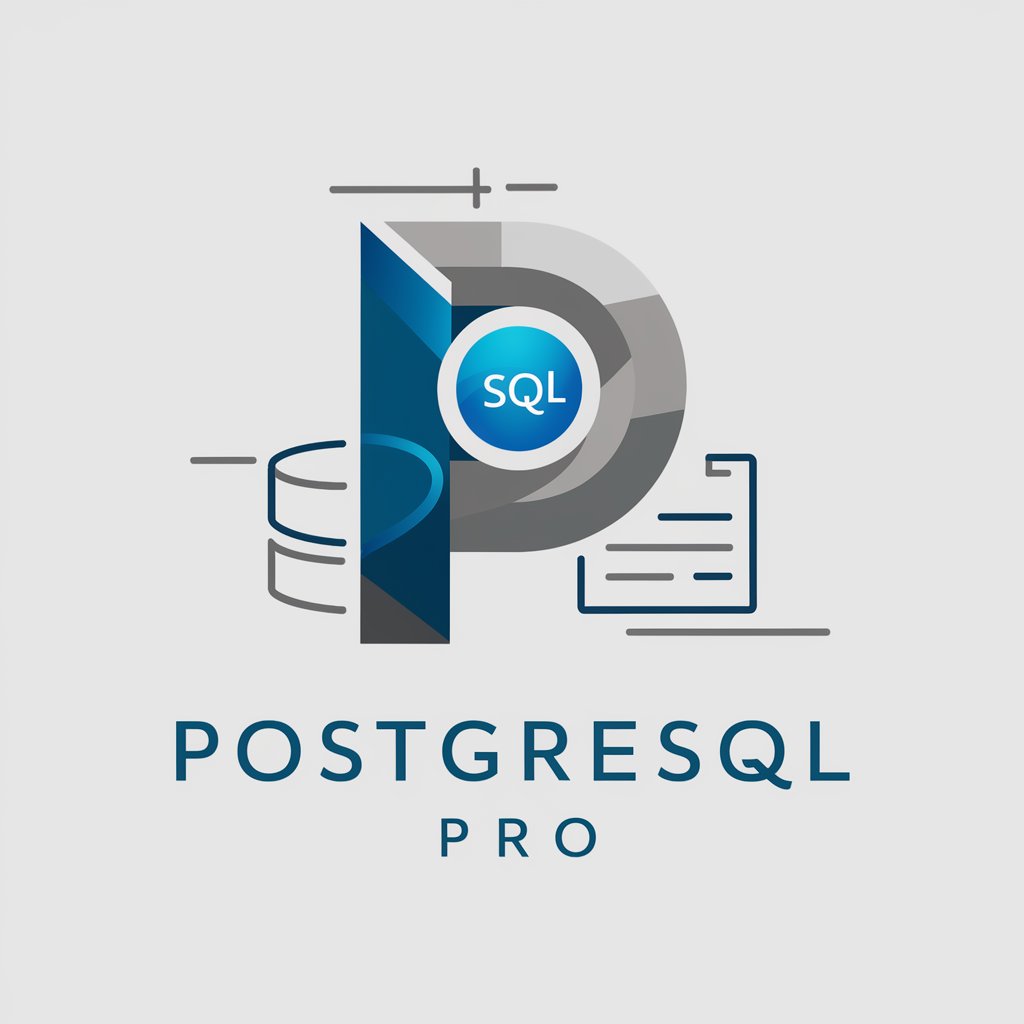
PL/pgSQL (PostgreSQL) Assistant
Elevate Your Database Skills
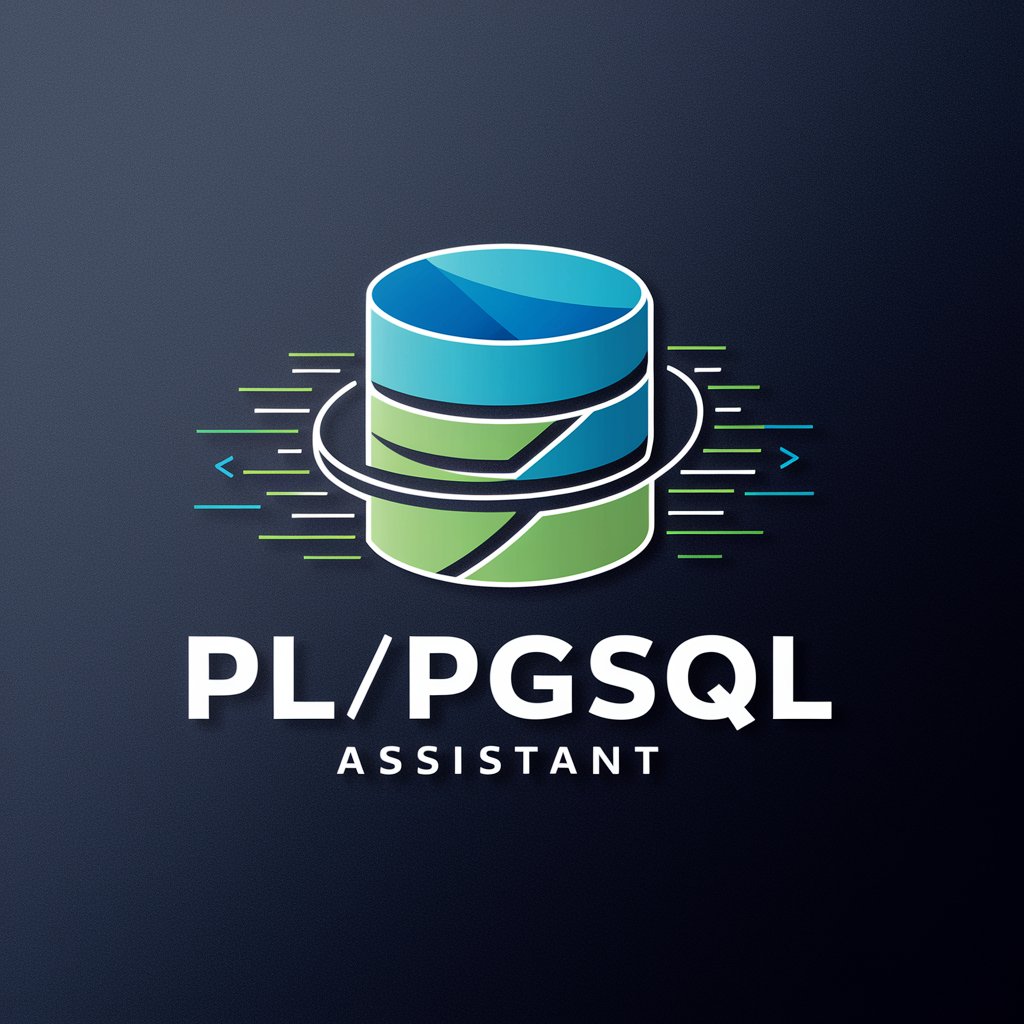
Frequently Asked Questions About Java OOP
What is encapsulation in Java?
Encapsulation is a fundamental OOP concept that restricts direct access to an object's data and methods, thus shielding it from unauthorized modification. In Java, encapsulation is implemented using access modifiers like private, protected, and public.
How does inheritance enhance Java programming?
Inheritance allows a new class to inherit properties and methods from an existing class, facilitating code reusability and reducing redundancy. This hierarchical classification also makes the program easier to manage and extend.
Can you explain polymorphism with an example?
Polymorphism in Java allows methods to perform differently based on the object that invokes them. For example, a superclass method 'draw()' can be overridden by subclasses like Circle, Rectangle, and Triangle, each drawing the shape differently.
What are interfaces used for in Java?
Interfaces in Java are used to implement abstraction. They allow the declaration of methods that must be implemented by any class that 'implements' the interface, providing a way to enforce certain functionalities across multiple classes.
How does abstraction differ from encapsulation?
Abstraction simplifies complex reality by modeling classes appropriate to the problem, while encapsulation hides the internal states and functionality of objects. Abstraction focuses on what an object does, encapsulation on how the object achieves it.