C++ RAII Resource Wizardry - C++ RAII Expertise
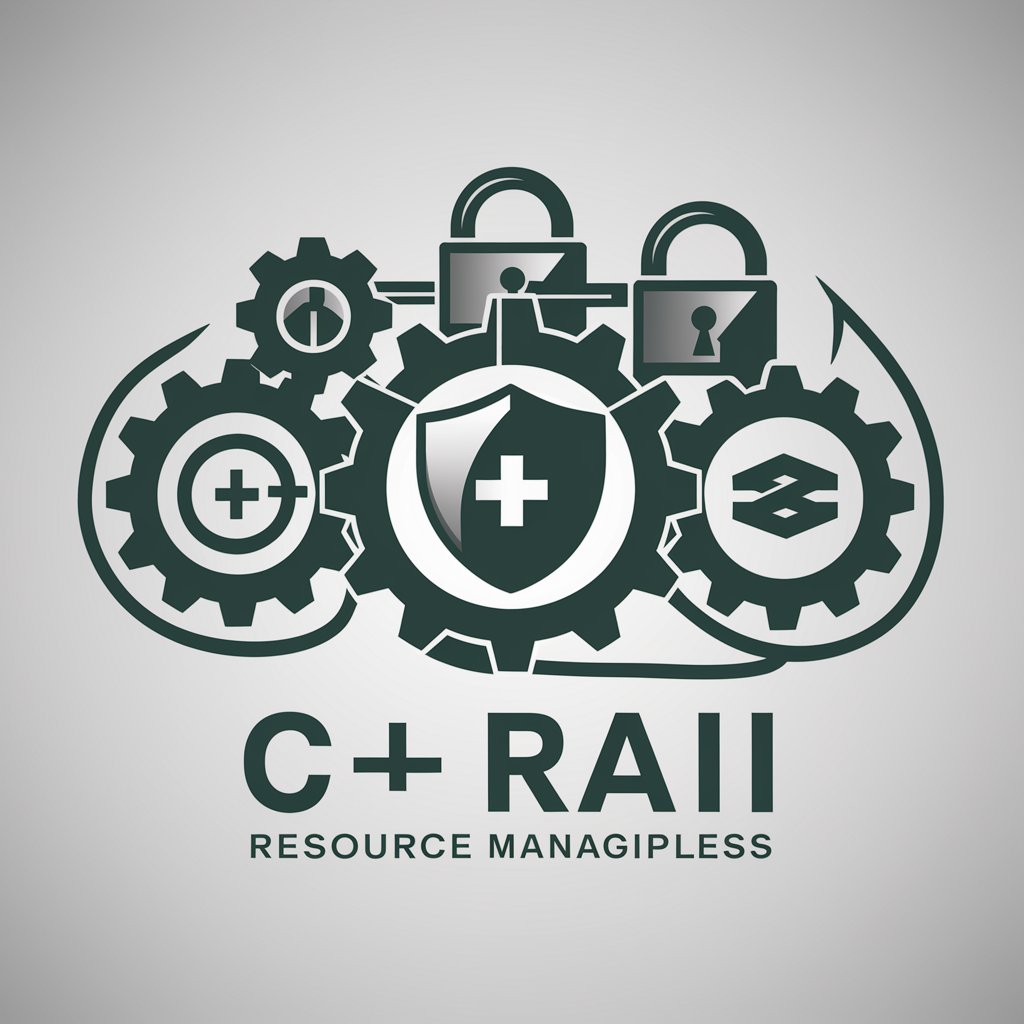
Hello! Let's optimize your C++ resource management with RAII.
Automate Resource Management with AI
How can I implement RAII for file handling in C++?
What are the best practices for writing a custom smart pointer in C++?
Can you demonstrate RAII with a network socket example in C++?
How do I manage dynamic memory in C++ using RAII principles?
Get Embed Code
Introduction to C++ RAII Resource Wizardry
C++ RAII (Resource Acquisition Is Initialization) Resource Wizardry is a specialized paradigm in C++ programming that emphasizes efficient resource management through the lifecycle of an object. The core principle involves acquiring resources during object creation and releasing them upon object destruction, encapsulating resource management within an object's lifetime. This approach mitigates resource leaks, ensures exception safety, and promotes cleaner, more maintainable code. For example, a database connection class using RAII would open the connection in its constructor and close it in its destructor, ensuring the connection is properly managed and released, regardless of how the object's lifecycle ends. Powered by ChatGPT-4o。
Main Functions of C++ RAII Resource Wizardry
Resource Management
Example
class FileWrapper { std::fstream file; public: FileWrapper(const std::string& filename) { file.open(filename); } ~FileWrapper() { if (file.is_open()) file.close(); }};
Scenario
Automatically managing file resources, opening upon object creation and closing upon destruction, preventing file descriptor leaks.
Memory Management
Example
class MemoryBlock { std::unique_ptr<int[]> data; public: MemoryBlock(size_t size) : data(std::make_unique<int[]>(size)) {} };
Scenario
Safely allocating and deallocating dynamic arrays, using smart pointers to prevent memory leaks and dangling pointers.
Concurrency Control
Example
class MutexLock { std::mutex& m; public: explicit MutexLock(std::mutex& mutex) : m(mutex) { m.lock(); } ~MutexLock() { m.unlock(); }};
Scenario
Ensuring thread safety by locking a mutex upon object creation and automatically unlocking it upon destruction.
Ideal Users of C++ RAII Resource Wizardry Services
Software Developers
Developers writing C++ applications, especially those dealing with low-level resource management (files, network connections, memory) or multi-threading, benefit from RAII for its ability to automate cleanup and prevent leaks, leading to robust, error-free code.
System Architects
System architects designing large-scale, high-reliability C++ systems can leverage RAII principles to enforce resource management policies, ensuring that resource acquisition and release are consistently handled across the system, reducing maintenance overhead.
How to Use C++ RAII Resource Wizardry
1
Start your journey by exploring yeschat.ai for a hassle-free trial, requiring no login or subscription to ChatGPT Plus.
2
Familiarize yourself with C++ fundamentals, especially smart pointers, constructors, destructors, and copy/move semantics, as these are crucial for implementing RAII effectively.
3
Identify the resources your application uses that require careful management (e.g., memory, file handles, database connections) to apply RAII patterns.
4
Implement classes that encapsulate these resources, ensuring that acquisition occurs in the constructor and release in the destructor, while also appropriately handling copy and move operations.
5
Regularly review and refactor your code to leverage modern C++ features (such as smart pointers) for safer and more efficient resource management.
Try other advanced and practical GPTs
AdCraft Pro Expert
Crafting Clicks with AI-Powered Ads
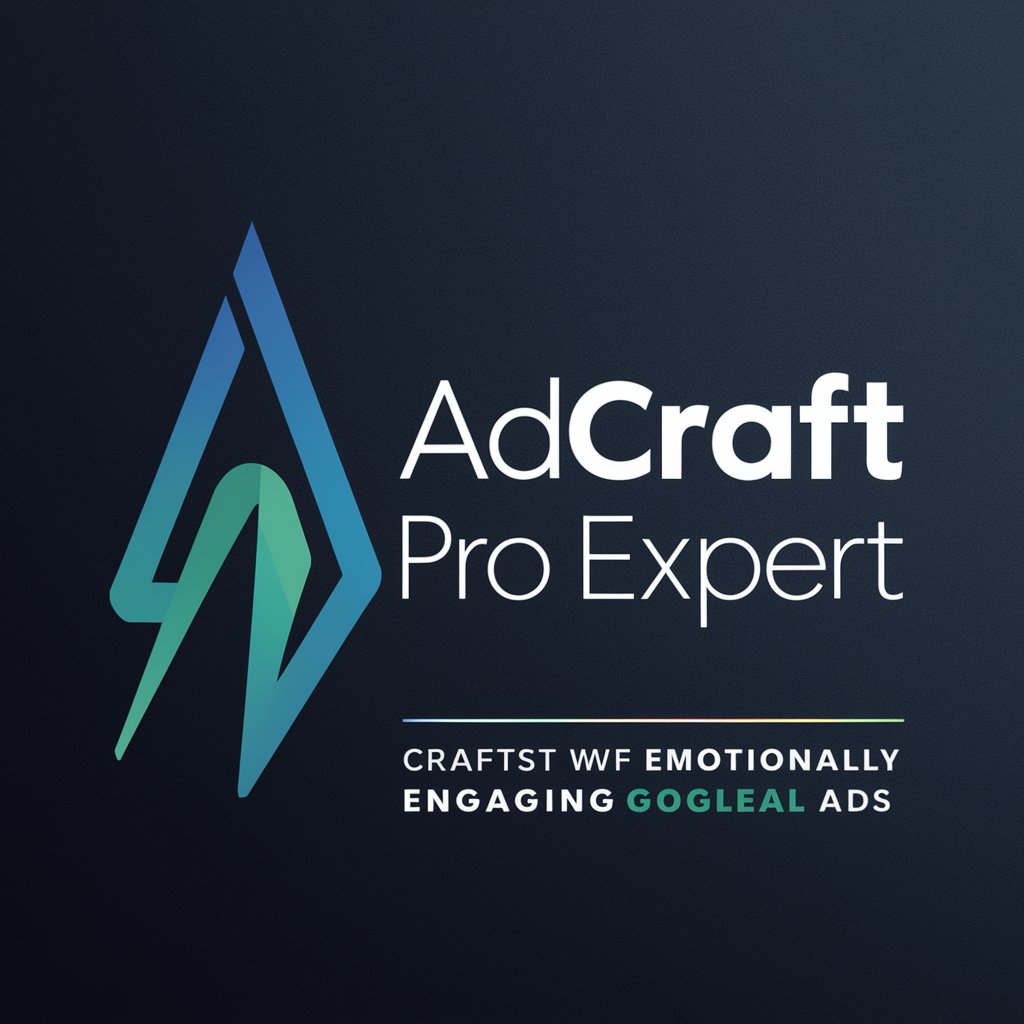
Jamie meaning?
Unlock detailed insights with AI
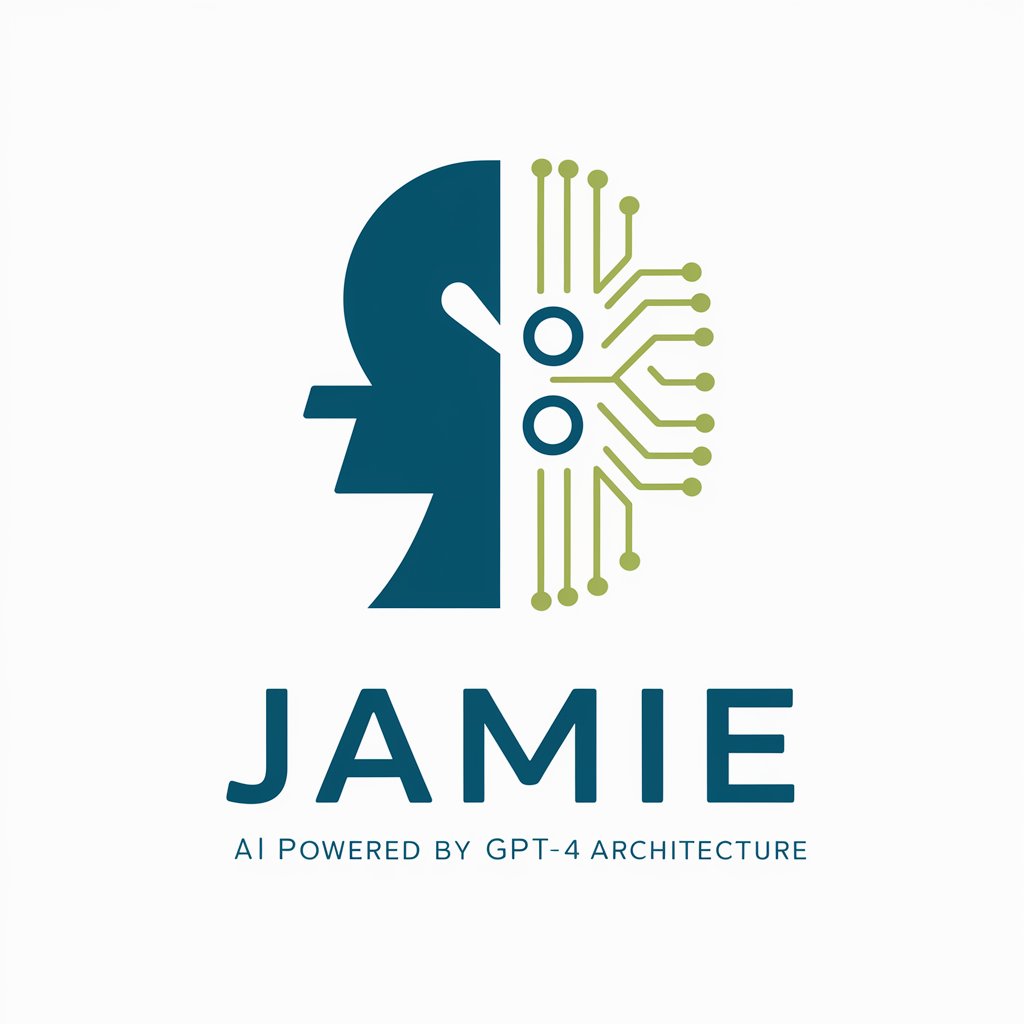
Game Edu Creator
Craft engaging educational games with AI
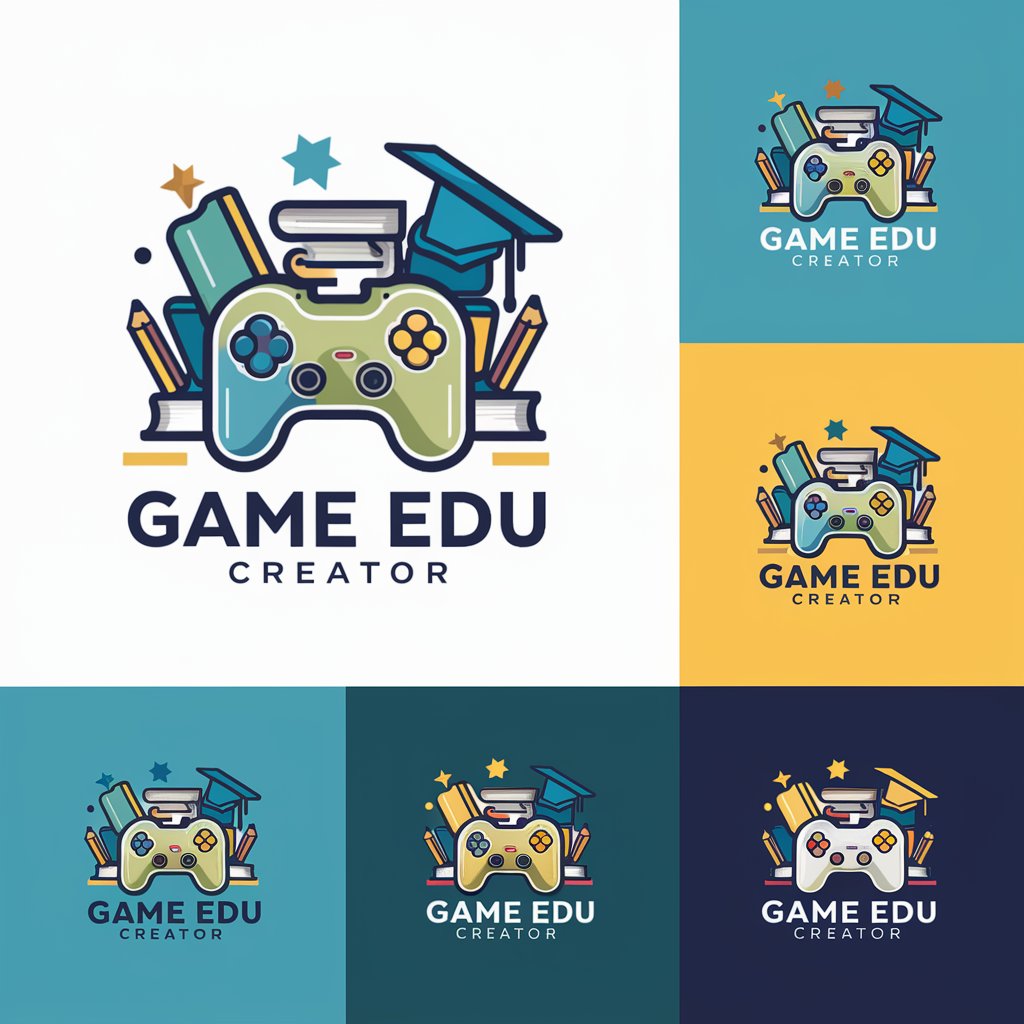
Stab Media SEO Writer
Empowering SEO with AI-driven writing
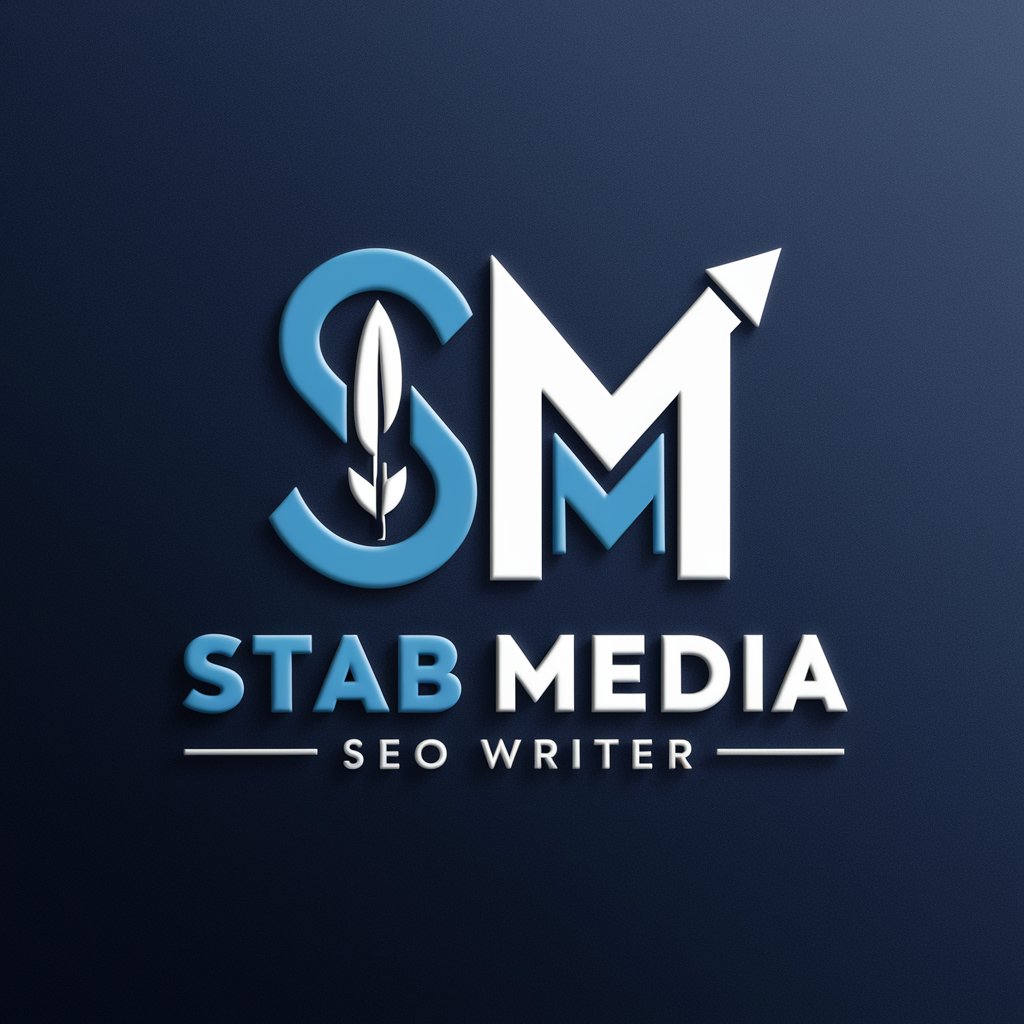
Eat Z Bugz
Revolutionizing Cooking with AI-Powered Bug Recipes
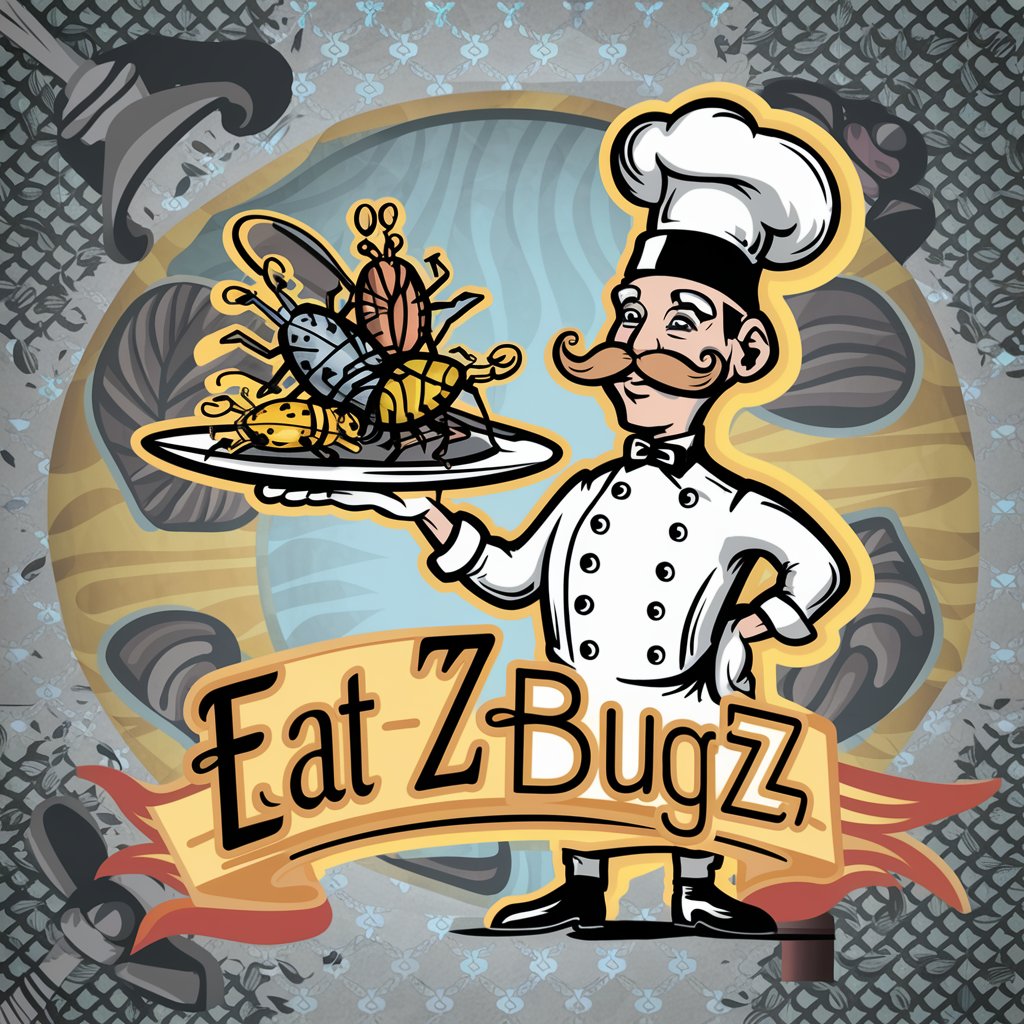
Vitest Generator
Automating Your Testing Workflow with AI
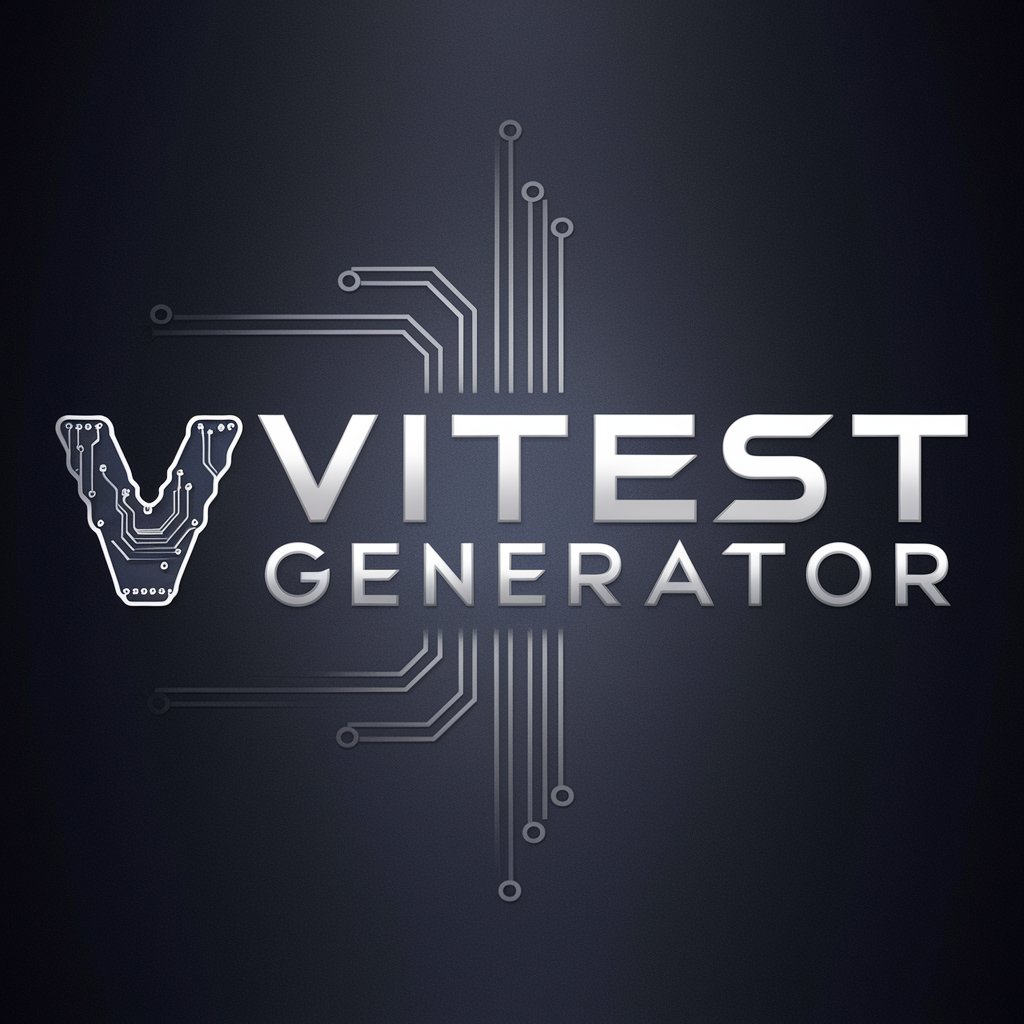
Listening Canvas
Transform audio into visual stories.
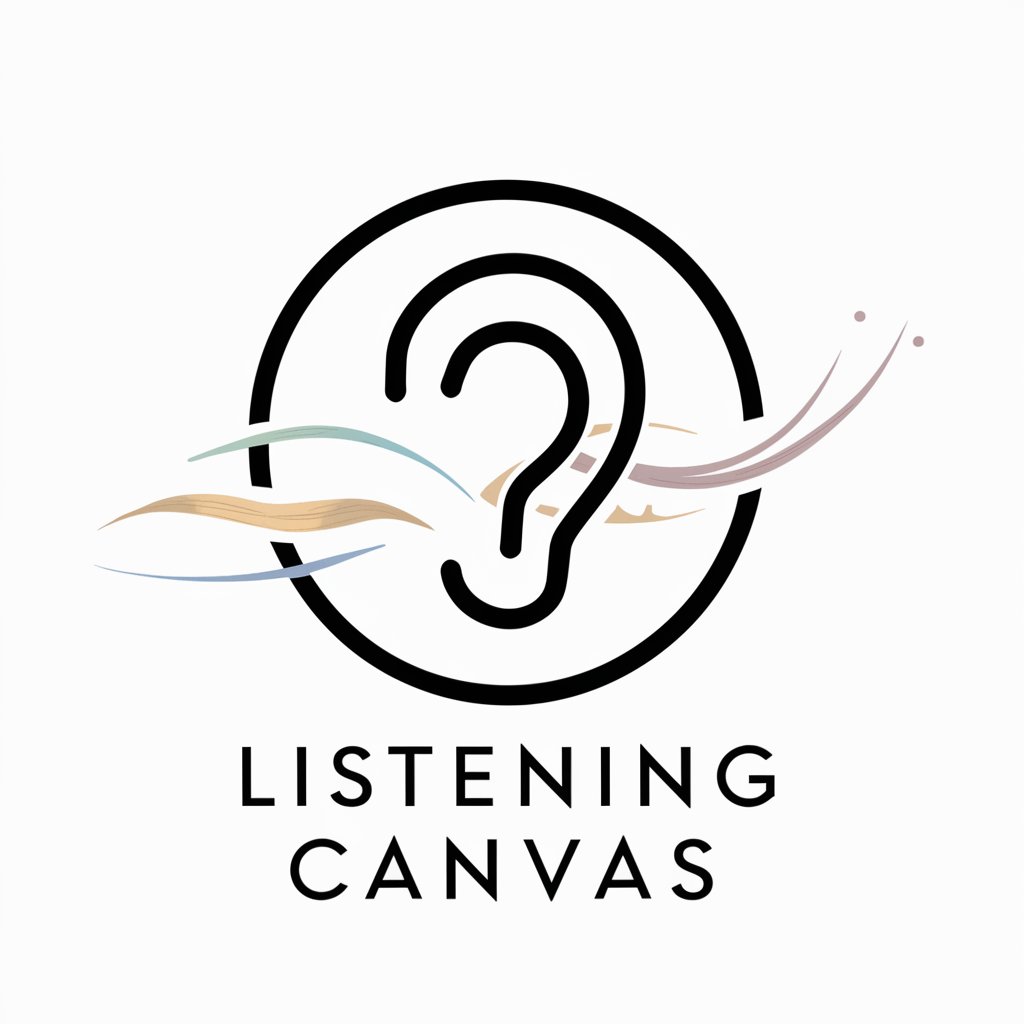
Mon ChefNutri
Eat smart, live well with AI-powered nutrition.
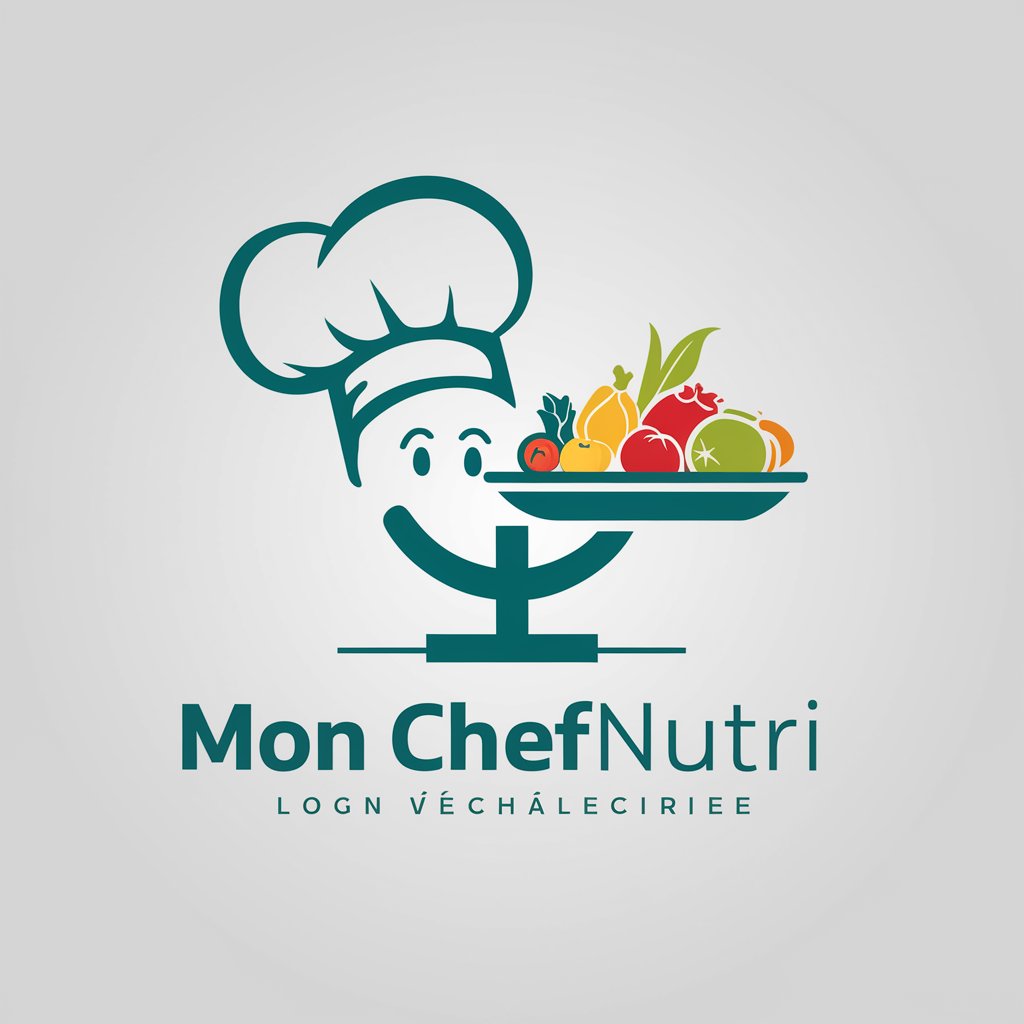
Spreuken en gezegden
Illuminate sayings with AI-driven insights and illustrations.
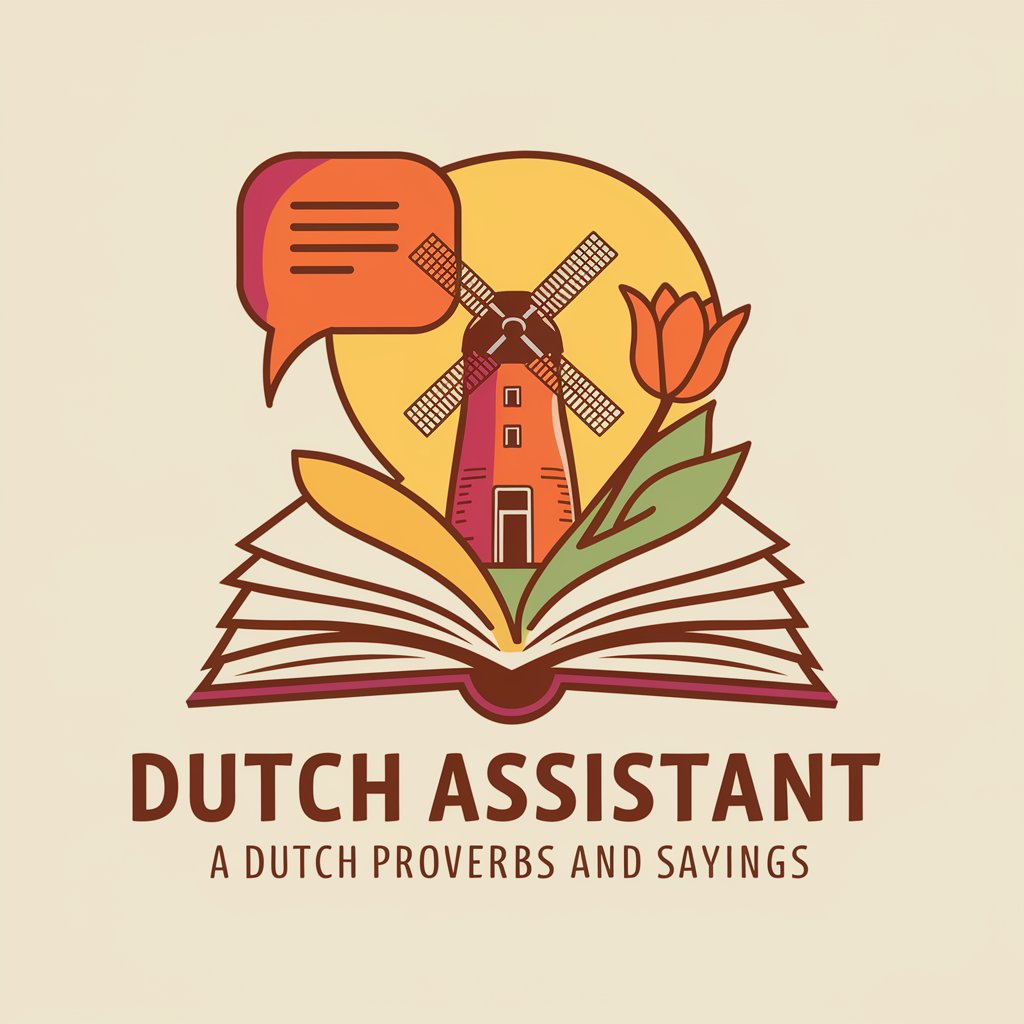
Multi-Asset-Economic GPT
AI-Powered Economic and Investment Insights
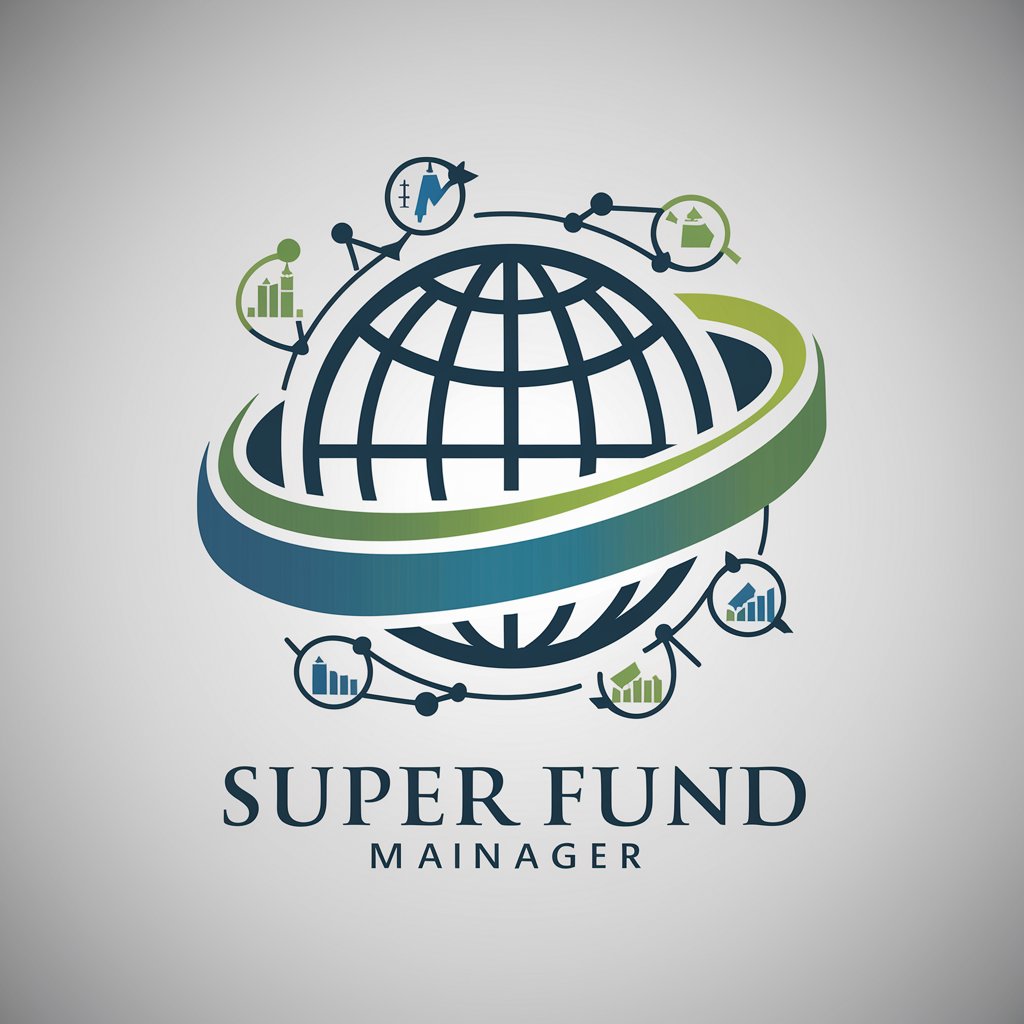
Curio
Unlock Your Curiosity with AI
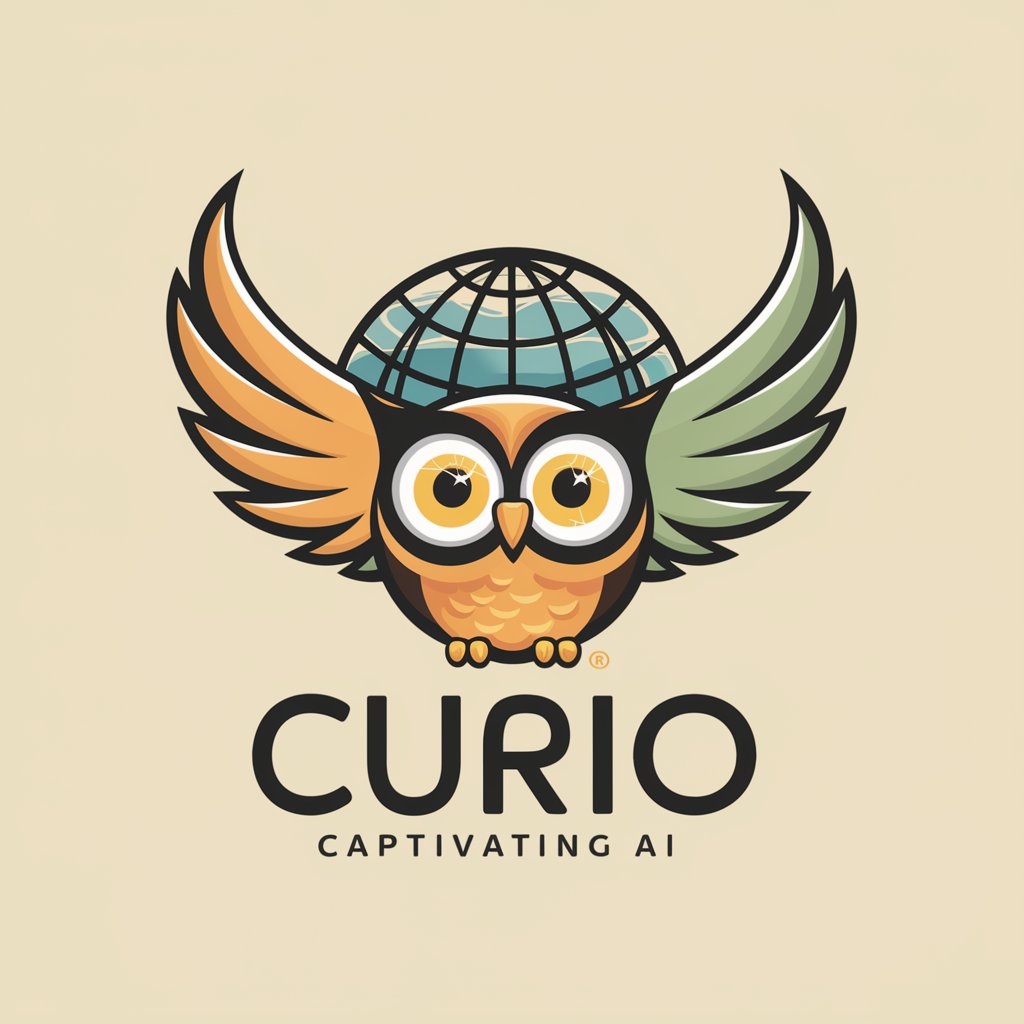
TU CONTABLE Digitalización Automática de Facturas
Streamline Your Invoices with AI
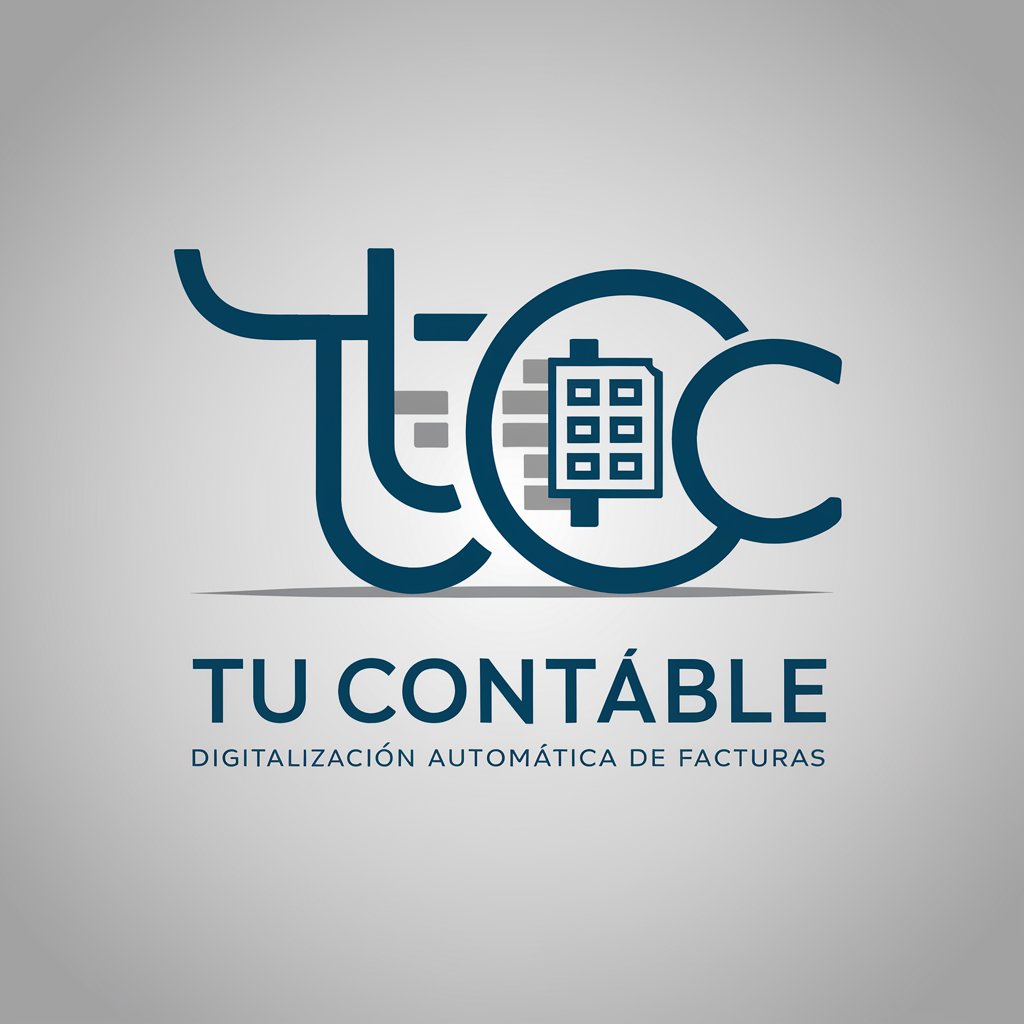
Frequently Asked Questions About C++ RAII Resource Wizardry
What exactly is C++ RAII Resource Wizardry?
C++ RAII Resource Wizardry refers to the application of the Resource Acquisition Is Initialization (RAII) principle in C++ programming, ensuring resources are managed safely and efficiently through object lifecycles.
Why is RAII important in C++?
RAII prevents resource leaks and undefined behavior by ensuring resources such as memory, file handles, and network connections are automatically allocated and released with object creation and destruction.
How does RAII handle exceptions?
RAII inherently provides exception safety by guaranteeing resource release even if an exception occurs, as destructors are called for stack-allocated objects when unwinding the stack during an exception.
Can RAII be used with dynamic memory?
Yes, RAII is particularly effective with dynamic memory when using smart pointers like std::unique_ptr and std::shared_ptr, which automatically deallocate memory when no longer needed.
How do copy and move semantics relate to RAII?
Proper implementation of copy and move constructors and assignment operators is crucial in RAII to manage resource ownership and avoid issues like double deletion or resource leaks.