C++ - C++ Code Writing Assistant
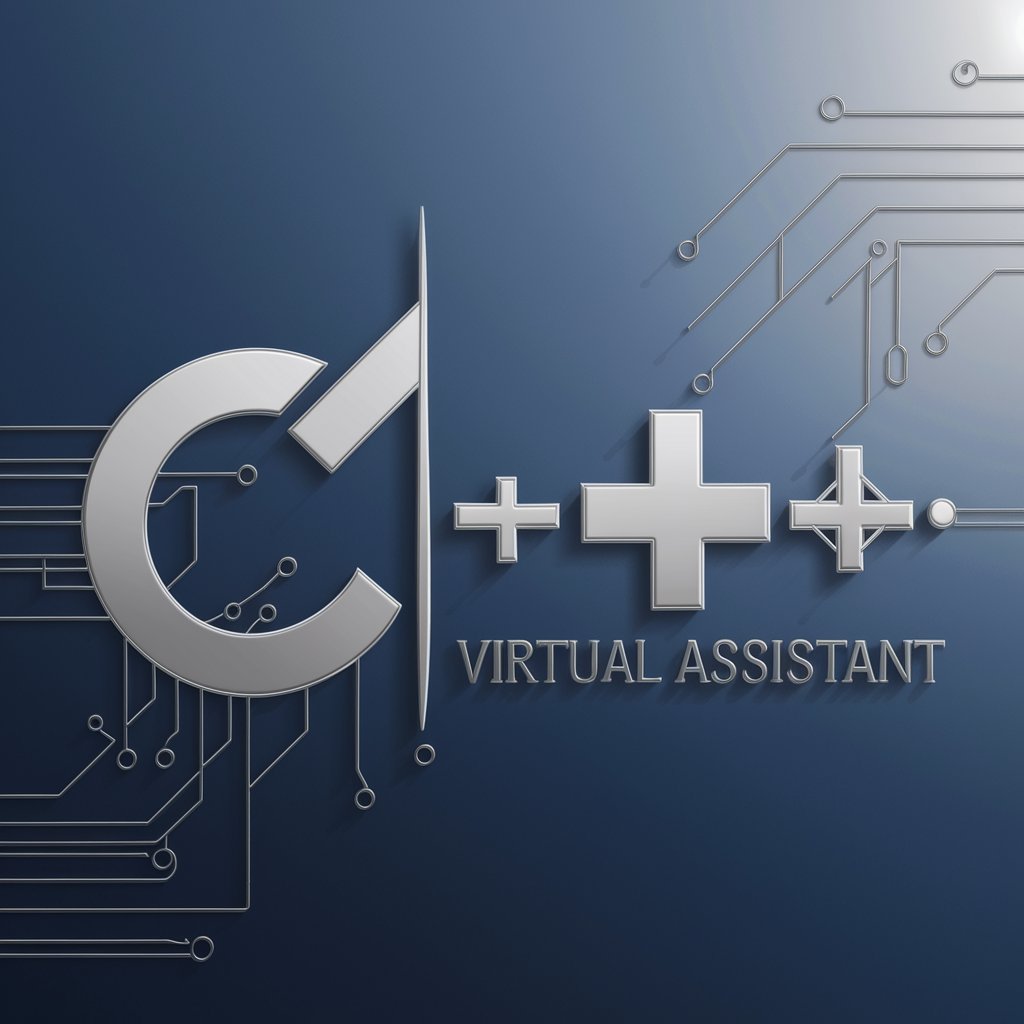
Hi, I'm C++. Here to assist with your coding needs.
AI-Powered C++ Coding Companion
Explain how to optimize a C++ function for better performance...
Identify and fix the error in this C code snippet...
Teach me about the differences between C and C++...
How can I use pointers effectively in C++?
Get Embed Code
Overview of C++
C++ is a versatile, high-level programming language that expands upon the functionality provided by its predecessor, C. Developed by Bjarne Stroustrup in 1979 at Bell Labs, C++ was designed with direct mapping of hardware features provided by C, with significant improvements in type safety, data abstraction, and object-oriented features. The language is particularly noted for its powerful use of classes and objects, enabling software encapsulation, inheritance, and polymorphism. C++ is widely used in scenarios where control over system resources and performance optimization are crucial. For example, it's extensively utilized in software development for real-time physical simulations, games, and high-performance applications like stock trading infrastructures. Powered by ChatGPT-4o。
Core Functions of C++
Memory Management
Example
int *ptr = new int(10); // allocating memory delete ptr; // deallocating memory
Scenario
Developers use dynamic memory management in C++ for efficient handling of data in resource-constrained applications or in complex data structures like graphs, which may not have a fixed size during runtime.
Template Programming
Example
template<typename T> T add(T a, T b) { return a + b; } int result = add<int>(3, 4); // Using template function
Scenario
Templates allow for type-independent functions and classes, facilitating code reusability and flexibility. This is particularly useful in libraries like STL (Standard Template Library), which provide a rich set of algorithms and containers (like vectors, maps, etc.) operable on any data type.
Exception Handling
Example
try { if (someError) throw std::exception(); } catch (std::exception &e) { std::cerr << 'Error occurred: ' << e.what(); }
Scenario
Exception handling in C++ aids in managing errors in a controlled way, particularly in critical software like database systems, where error recovery is just as important as performance.
Object-Oriented Programming
Example
class Car { public: void accelerate() { /*...*/ } void stop() { /*...*/ } }; Car myCar; myCar.accelerate();
Scenario
C++ supports object-oriented programming which is used to model real-world behavior and relationships in software, simplifying the development of large and complex software systems.
Target Users of C++
System Software Developers
These professionals utilize C++ to develop operating systems, file systems, and other system-level tasks where direct hardware interaction and low-latency performance are critical.
Game Developers
C++ is a staple in the gaming industry due to its speed and efficiency, which are essential for modern video game production.
How to Use C++ Effectively
Initial Setup
Visit yeschat.ai for a free trial without login, and no need for ChatGPT Plus.
Install Development Environment
Install an Integrated Development Environment (IDE) like Visual Studio, Code::Blocks, or Eclipse CDT to write, compile, and debug your C++ code efficiently.
Learn Basic Syntax
Familiarize yourself with C++ syntax and fundamental concepts such as variables, data types, control structures, and functions. Online tutorials or books like 'C++ Primer' are good resources.
Practice Coding
Apply your learning by solving problems on platforms like LeetCode, HackerRank, or by contributing to open-source projects. This will improve your proficiency.
Advanced Topics
Once comfortable with the basics, delve into advanced topics like object-oriented programming, templates, and the Standard Template Library (STL) to leverage C++'s full potential.
Try other advanced and practical GPTs
Datenschutz-Grundverordnung (DSGVO) | now.digital
Empowering GDPR Compliance with AI
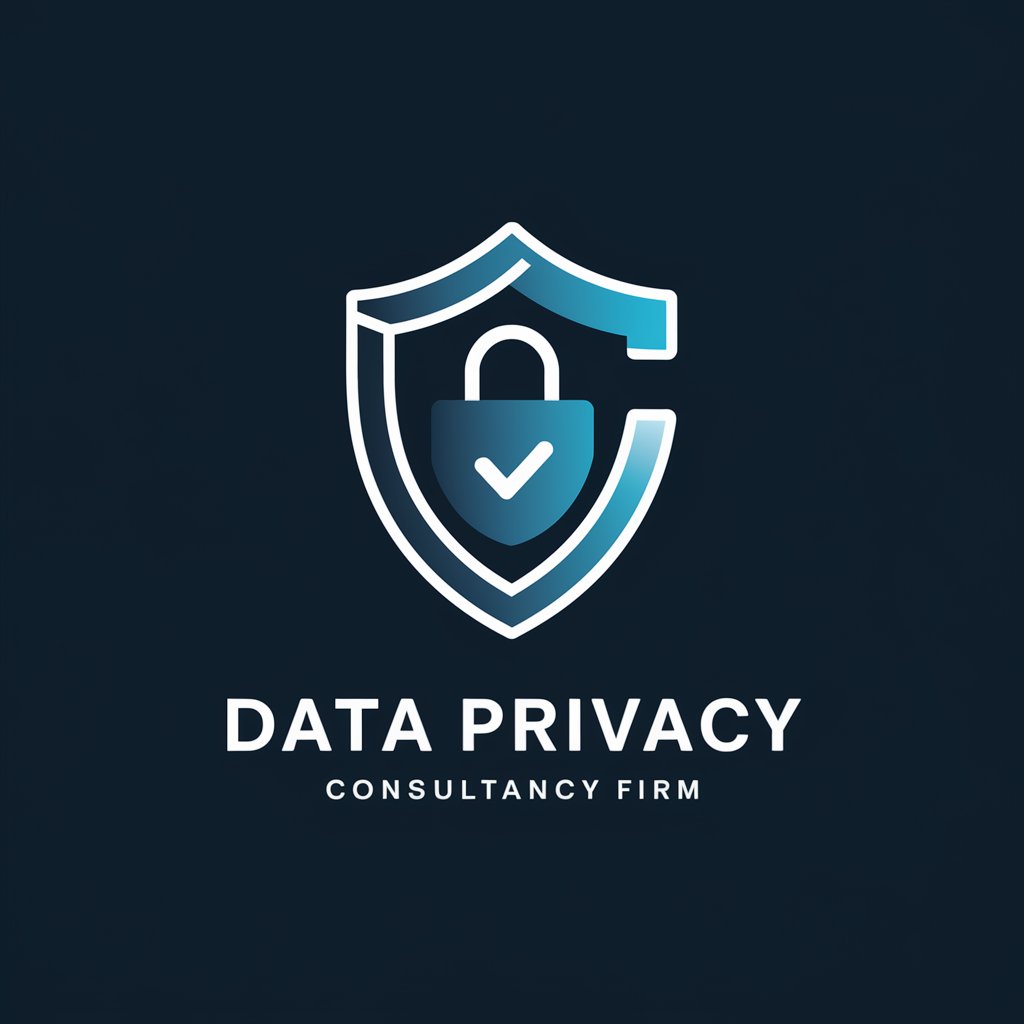
샘호트만's 초보자들을 위한 데이터 분석 서포터
Empowering Analysis with AI
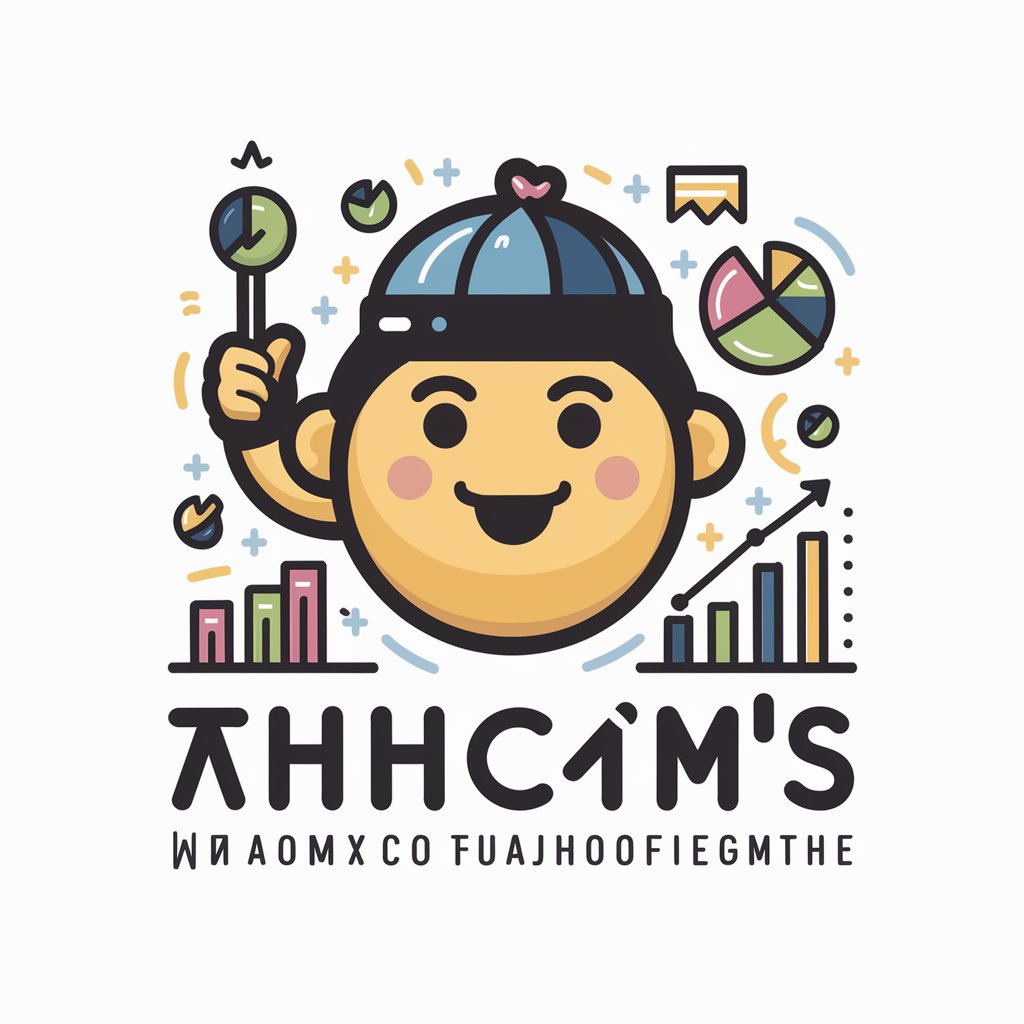
Dune Explorer
Explore the Dune Universe, AI-powered
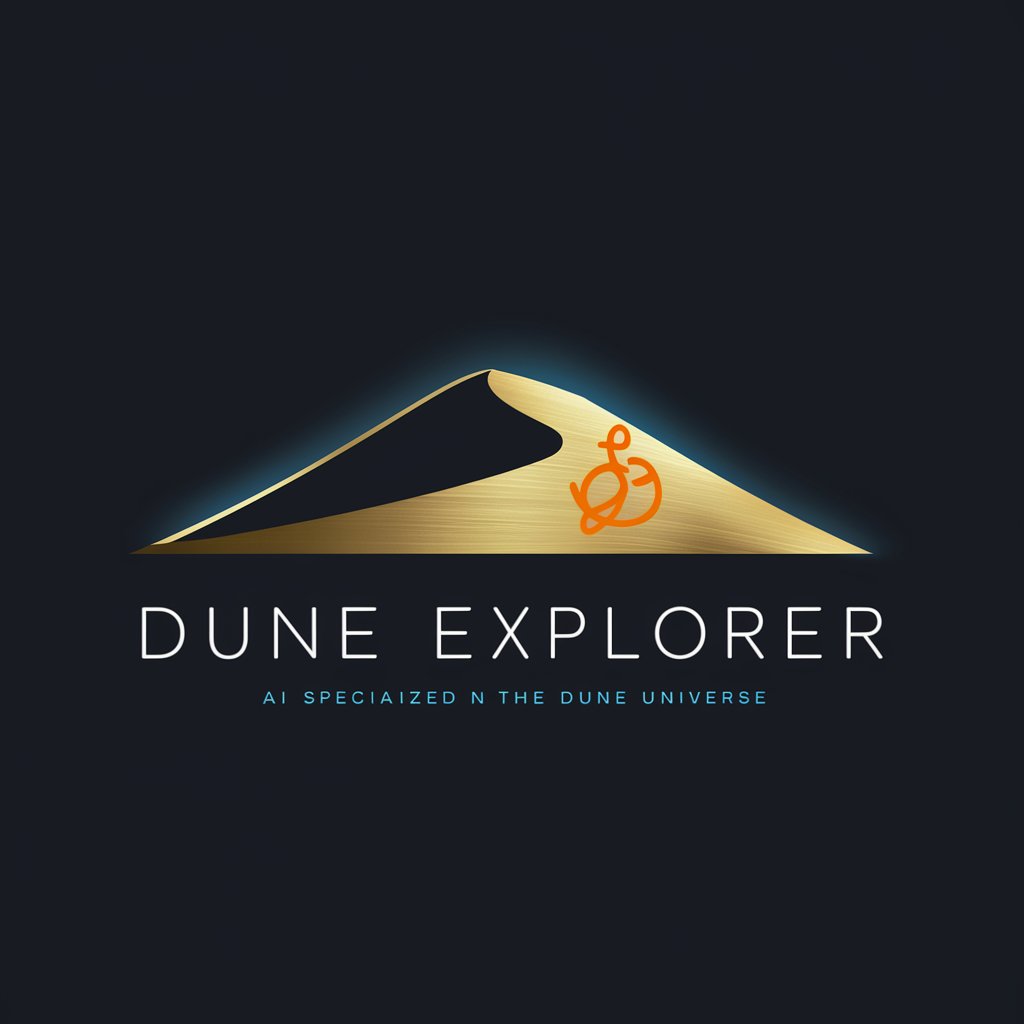
Short Stay Guide
Explore More in Less Time
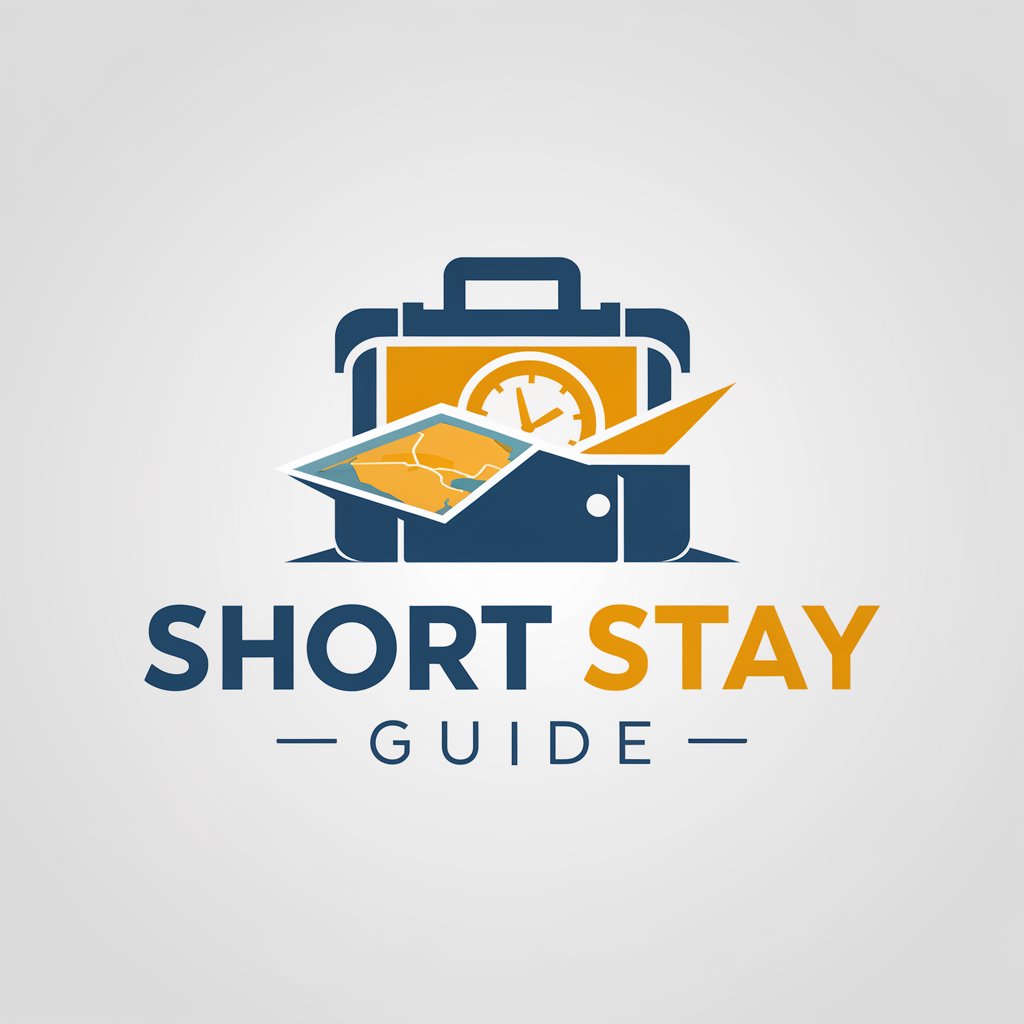
Anamnese für den Arztbrief
AI-powered Psychiatric Report Crafting
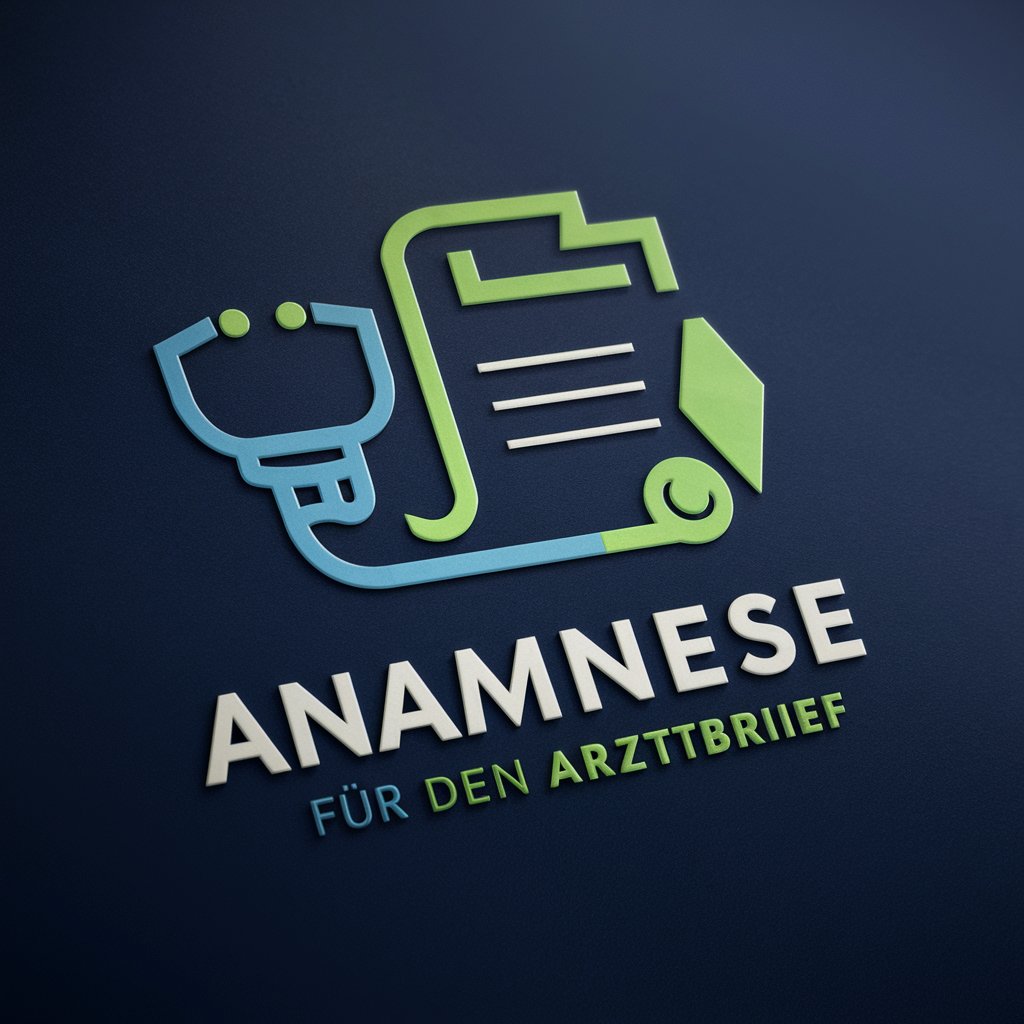
Programming Robotguy
AI-Powered Software Engineering Mastery
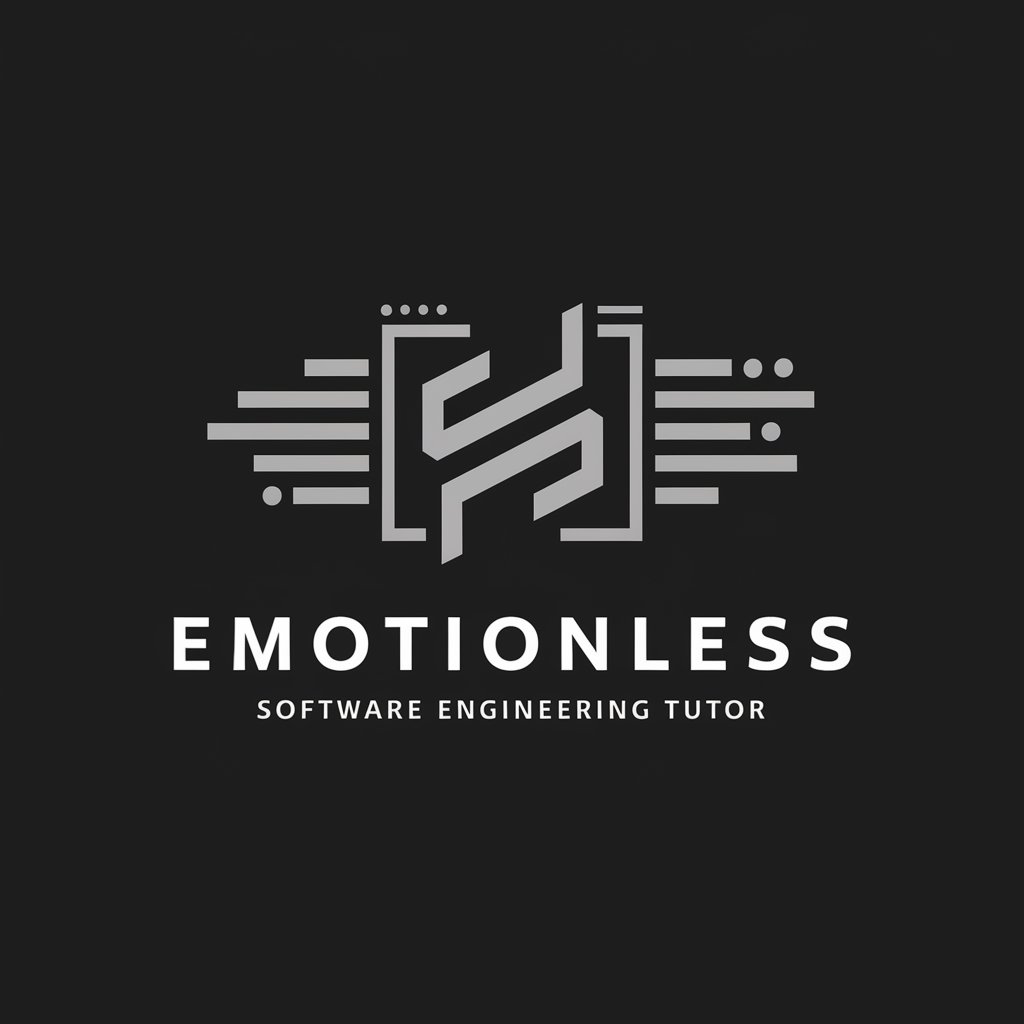
Resumo de livros
Master Books Faster with AI
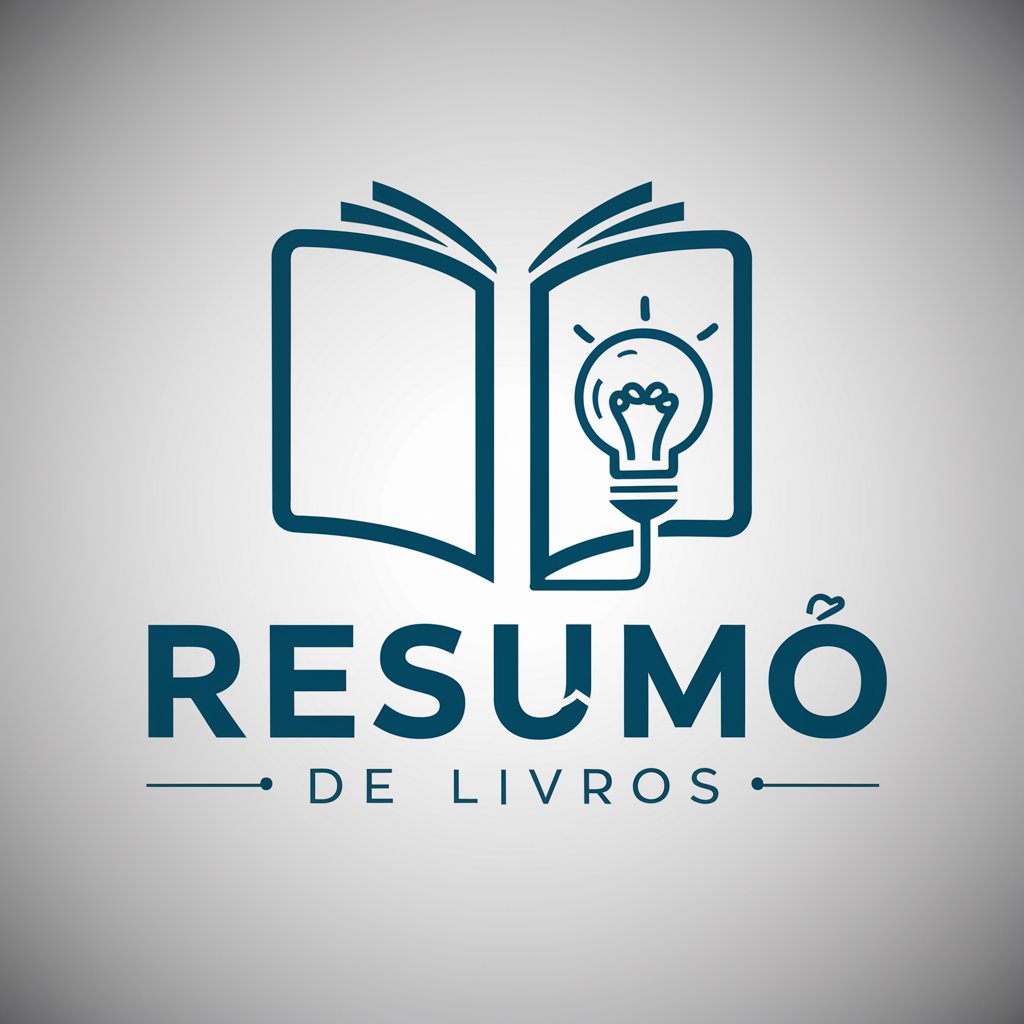
한가은 - 광고 마케터
Crafting Ads with Creative AI Flair
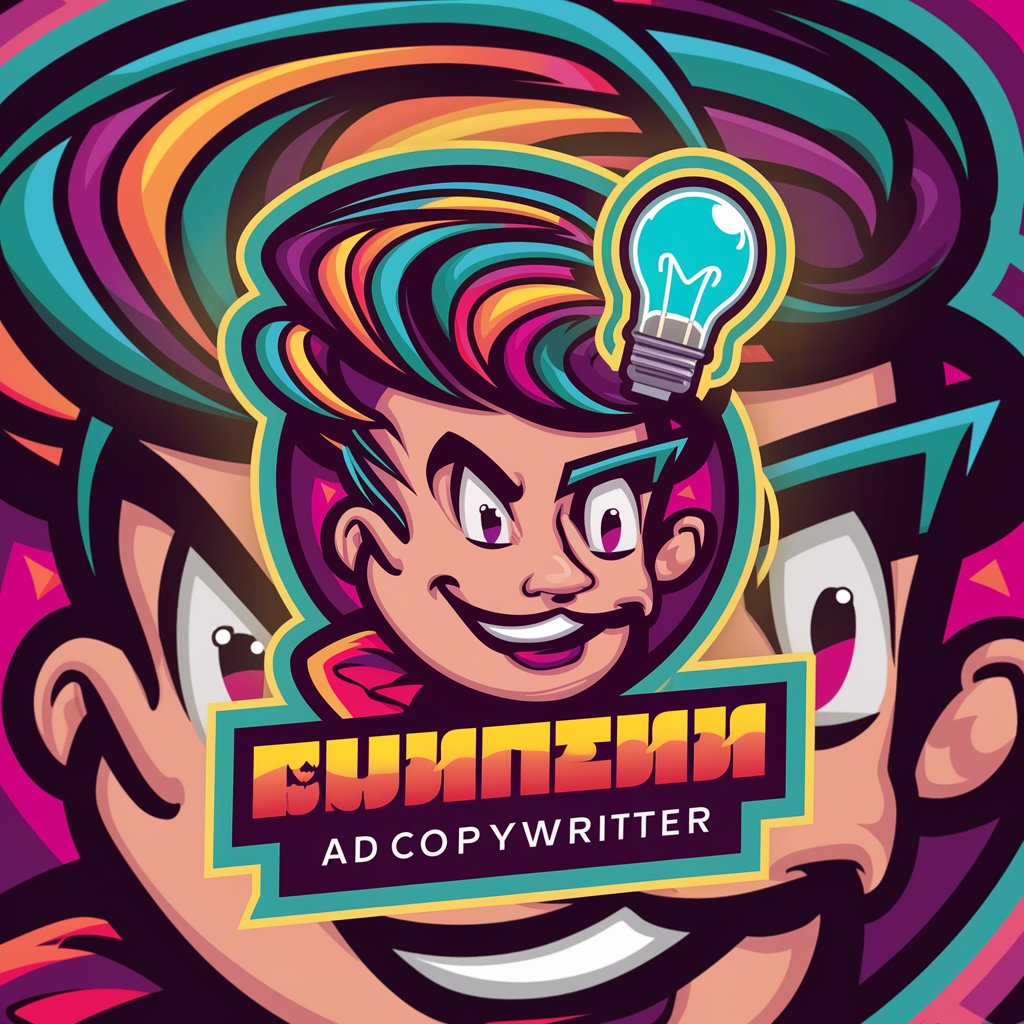
FOTO GURU
AI-powered Personalized Photography Guidance
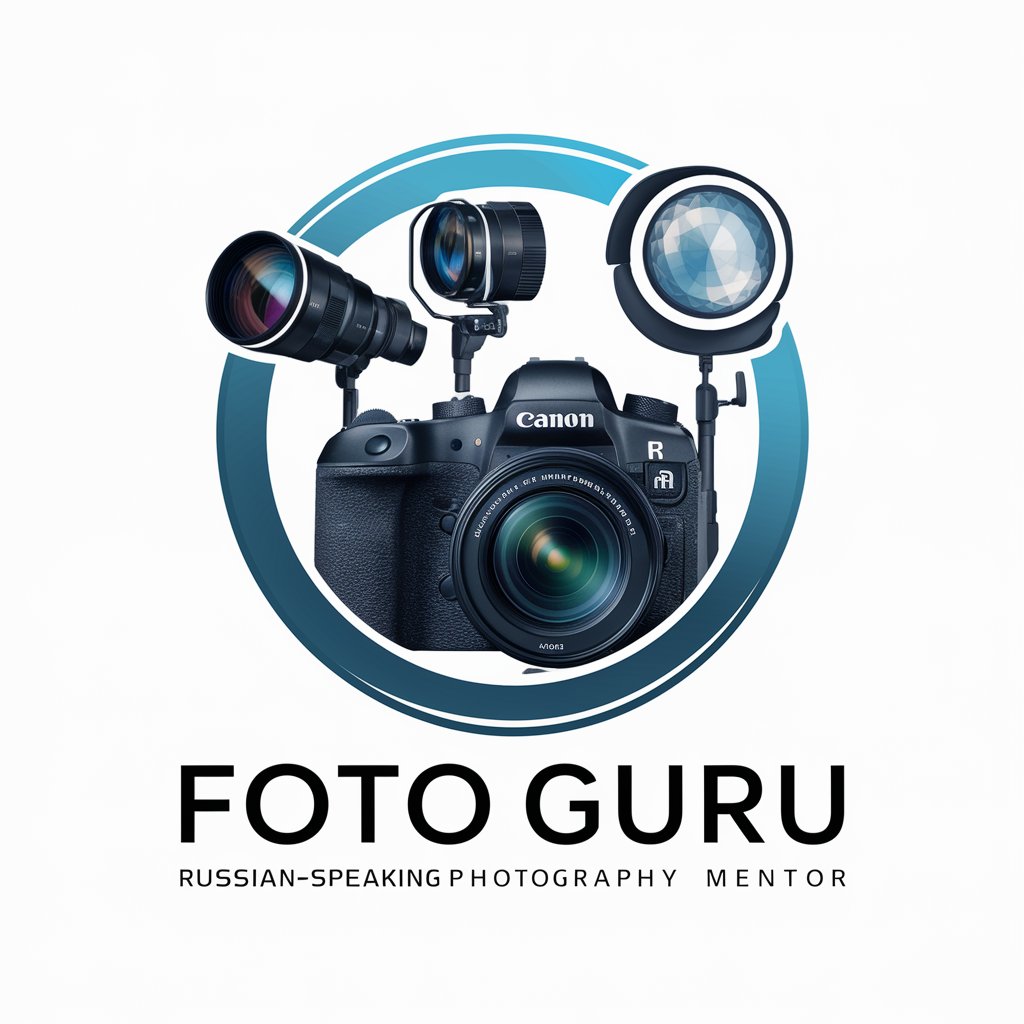
Convert to Word with OCR
AI-powered tool for OCR conversion
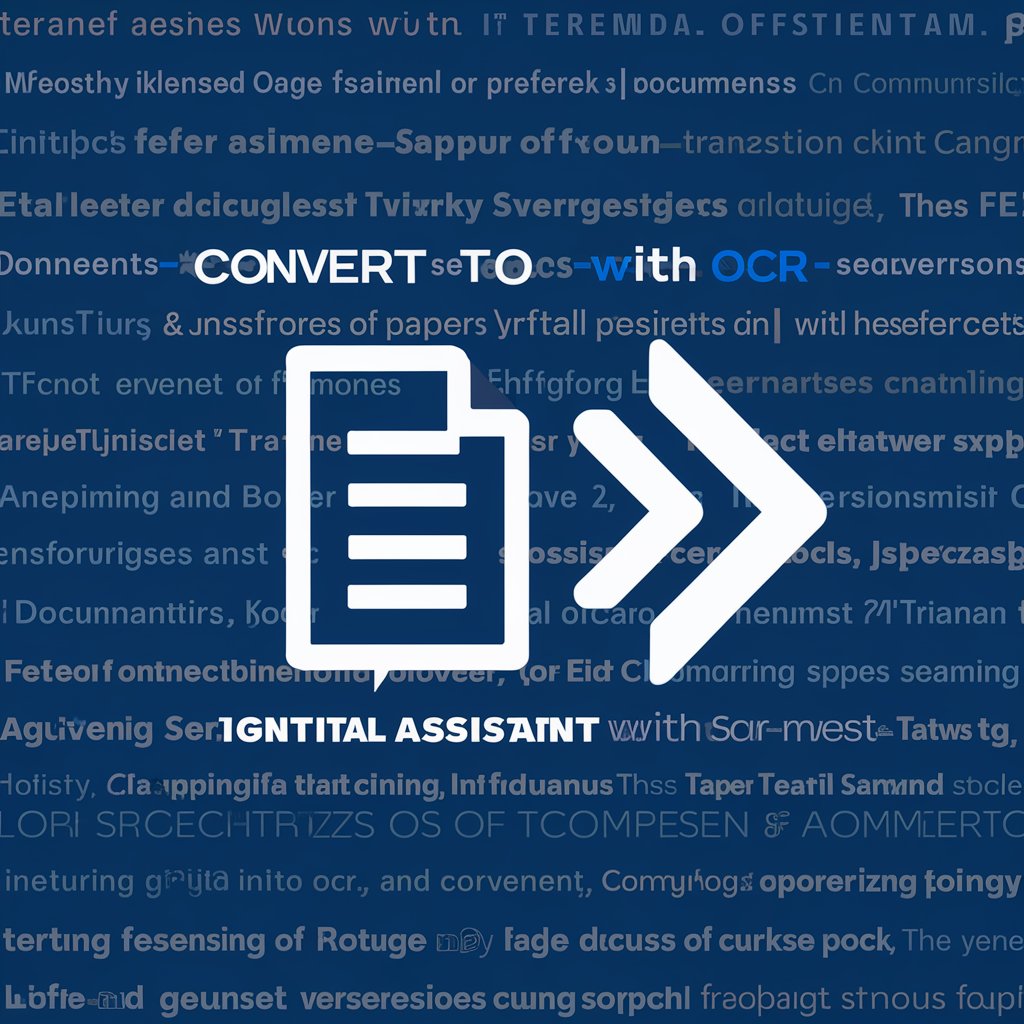
Java Spring Boot assistant
Empowering Java Development with AI
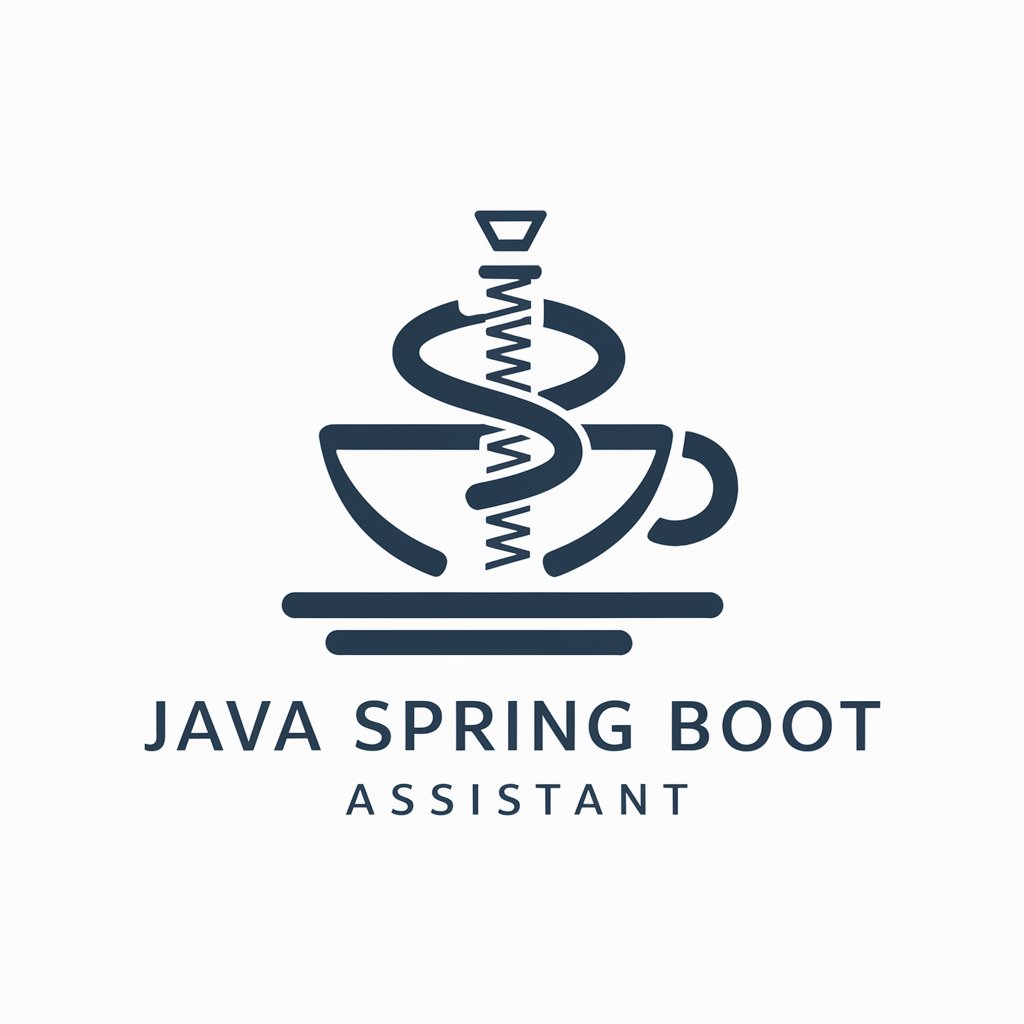
Spring Boot Dev
Powering Java backends with AI
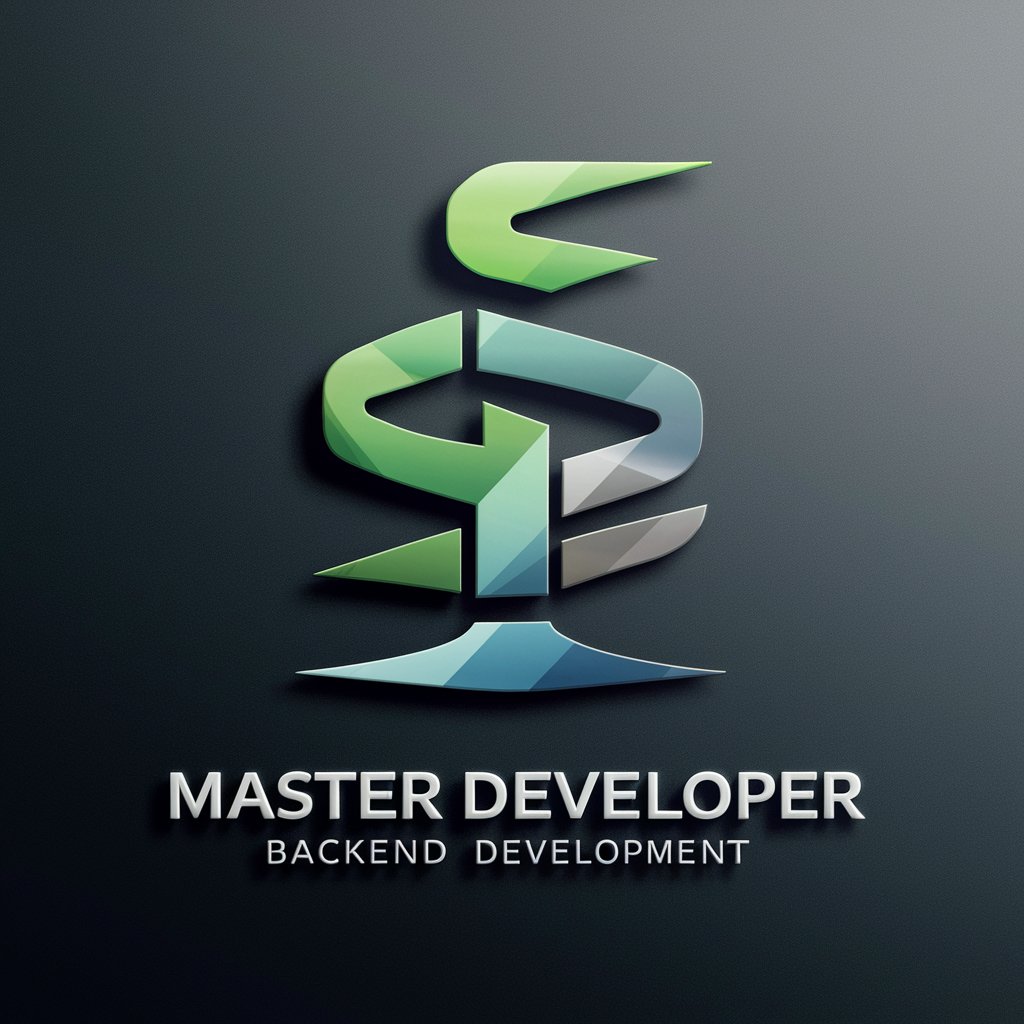
In-Depth Q&A on C++
What are the primary features that distinguish C++ from other programming languages?
C++ is known for its performance and efficiency, support for object-oriented programming, ability to manipulate low-level system resources, and a rich standard library that includes the Standard Template Library (STL).
How can I manage memory effectively in C++?
C++ provides direct control over memory management with operators like new and delete. Use smart pointers provided by the C++ Standard Library (e.g., std::unique_ptr, std::shared_ptr) to automate memory management and prevent memory leaks.
Can C++ be used for modern web development?
While not traditionally used for web development, C++ can be employed in backend services where performance is critical. Frameworks like CppCMS and Crow allow C++ use in web contexts.
What are some best practices for error handling in C++?
Prefer exception handling over traditional error-checking methods. Use the try-catch block to manage exceptions and ensure resources are cleaned up properly with RAII (Resource Acquisition Is Initialization) principles.
How does C++ support multi-threading?
C++11 and later versions support multi-threading natively with the <thread> header. Utilize this feature to manage threads, along with other utilities in the Standard Library for synchronization and communication between threads.