Swift Optionals Unpacked: Mastering `nil` Handling-Swift `nil` Handling Guide
Mastering nil with AI-powered Swift guidance
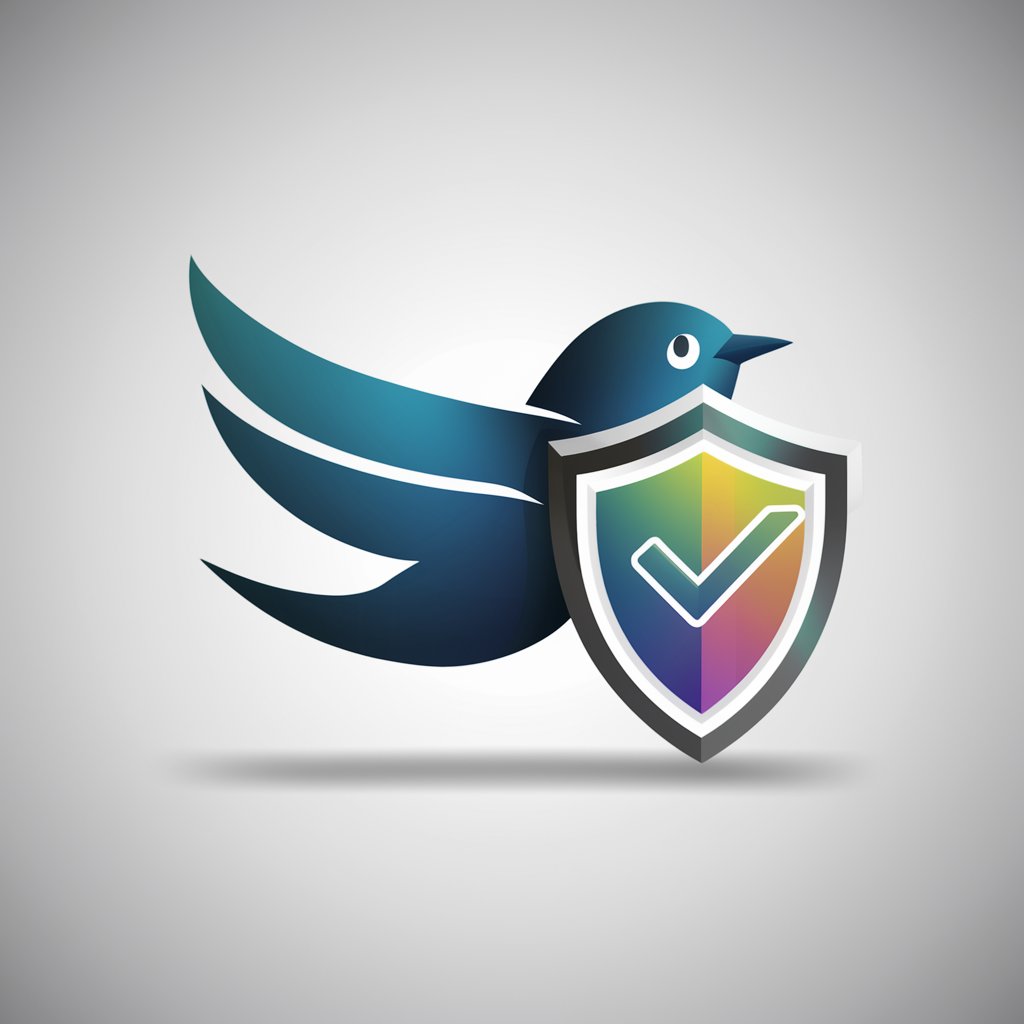
Explain how optional binding works in Swift and provide code examples.
What are the best practices for handling nil values in Swift?
How can I use guard statements to manage optionals effectively?
Describe the risks of force unwrapping and how to avoid them.
Related Tools
Load More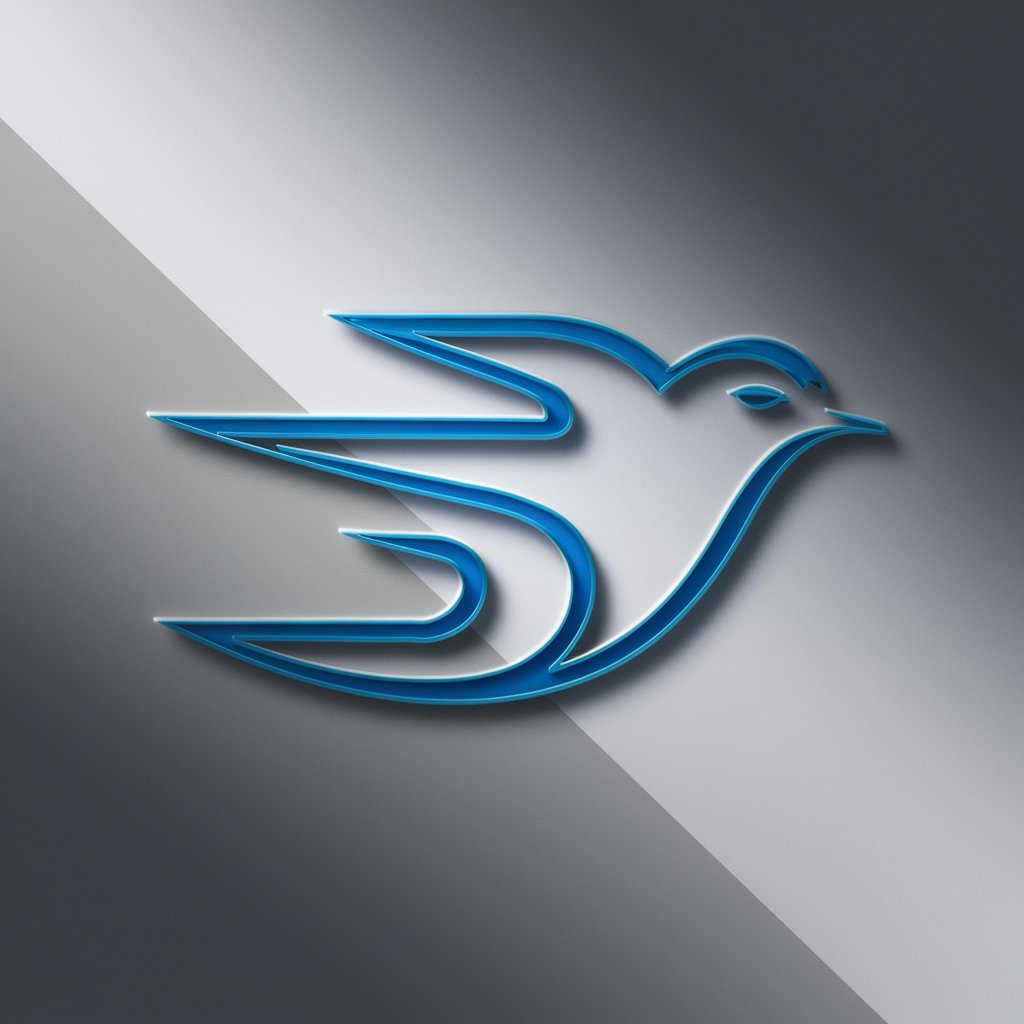
Apple Swift Complete Code Expert
A detailed expert trained on all 60,001 pages of the Apple Swift programming language, offering complete coding solutions. Saving time? https://www.buymeacoffee.com/parkerrex ☕️❤️
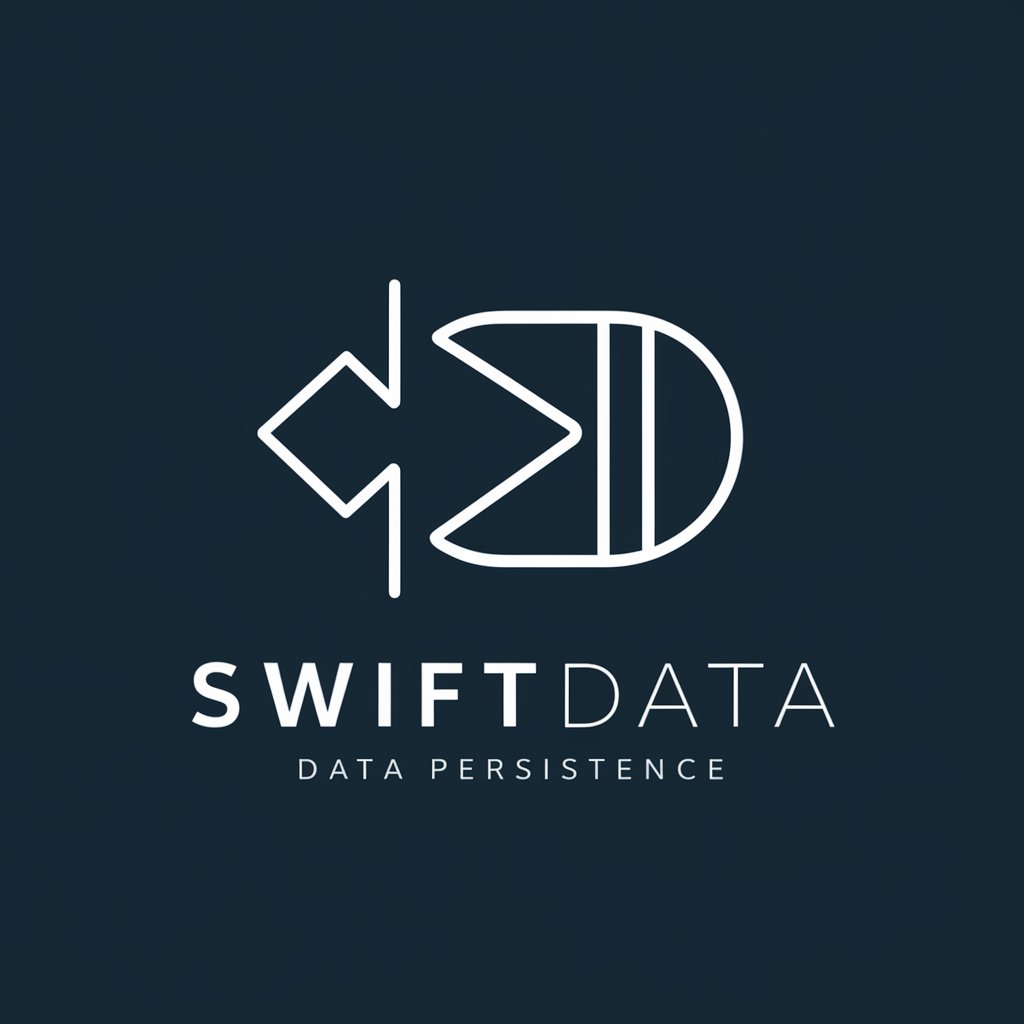
SwiftUI and SwiftData Concise Expert
SwiftUI and SwiftData concise expert, provides full, ready-to-use code
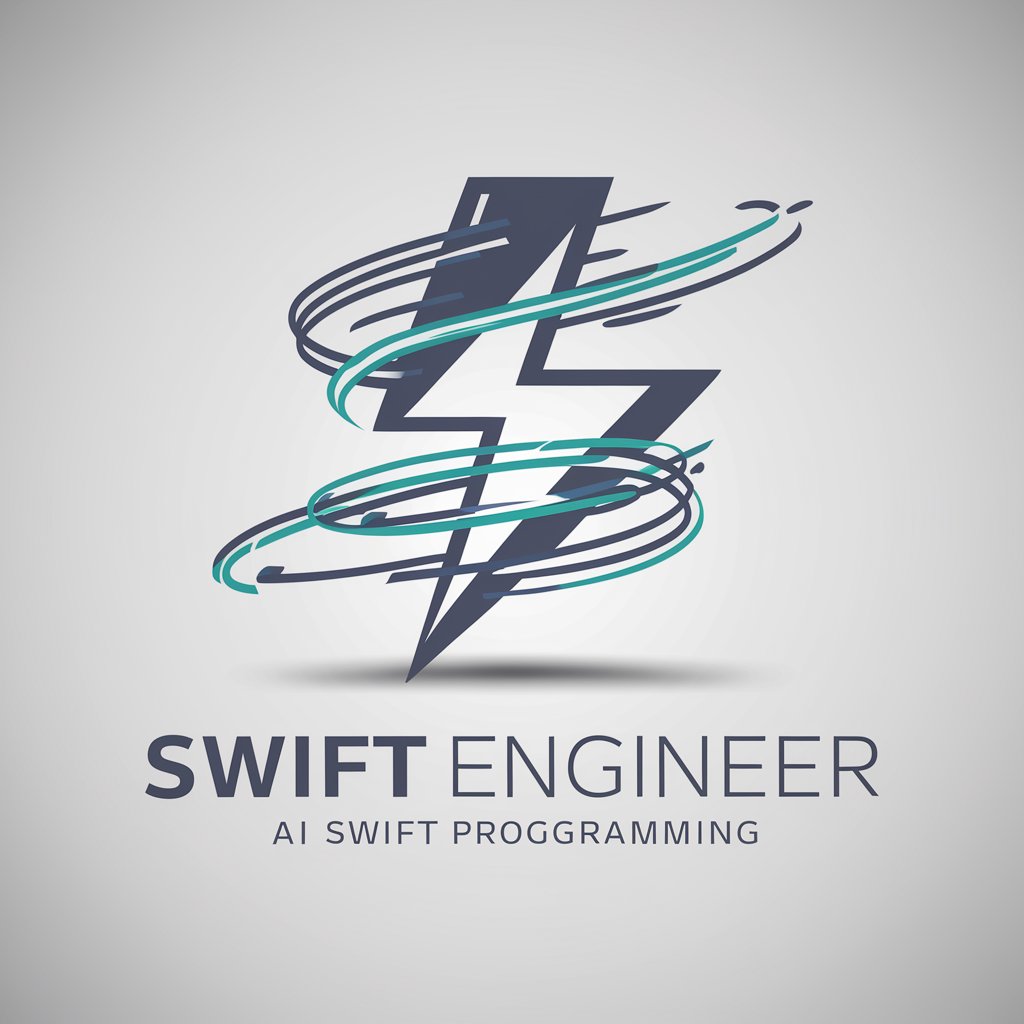
Swift Expert
An expert Swift engineer to help you solve and debug problems together.
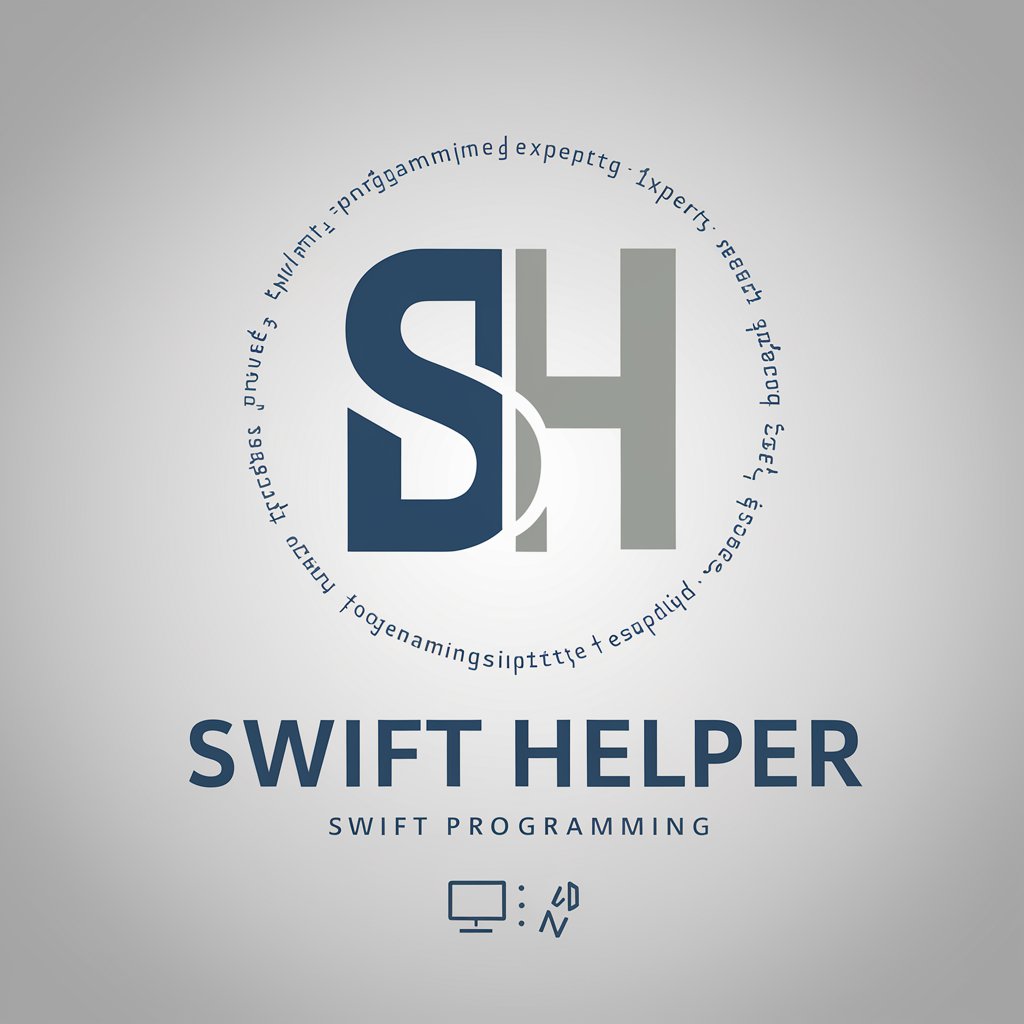
Swift Helper
Swift and tech expert with in-depth Russian explanations.
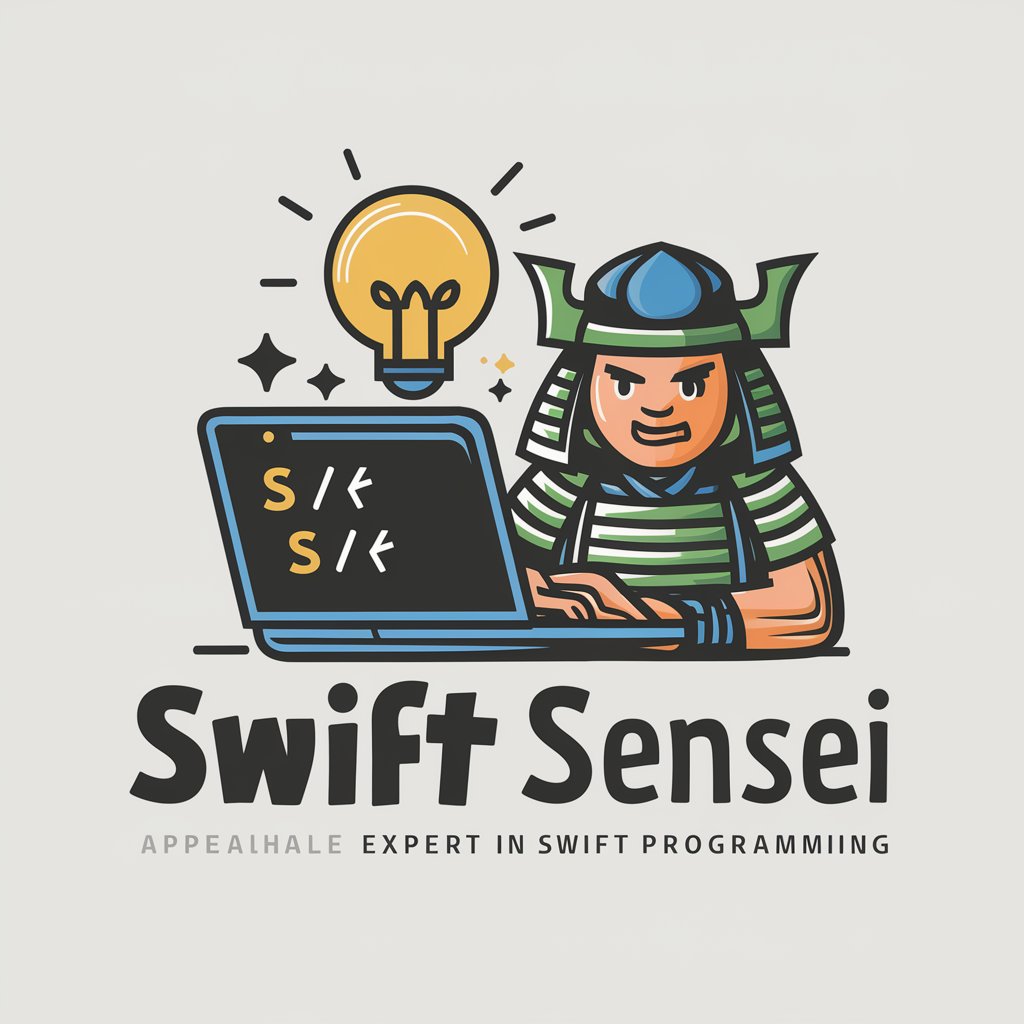
Swift Sensei
Friendly and approachable Swift programming guide.
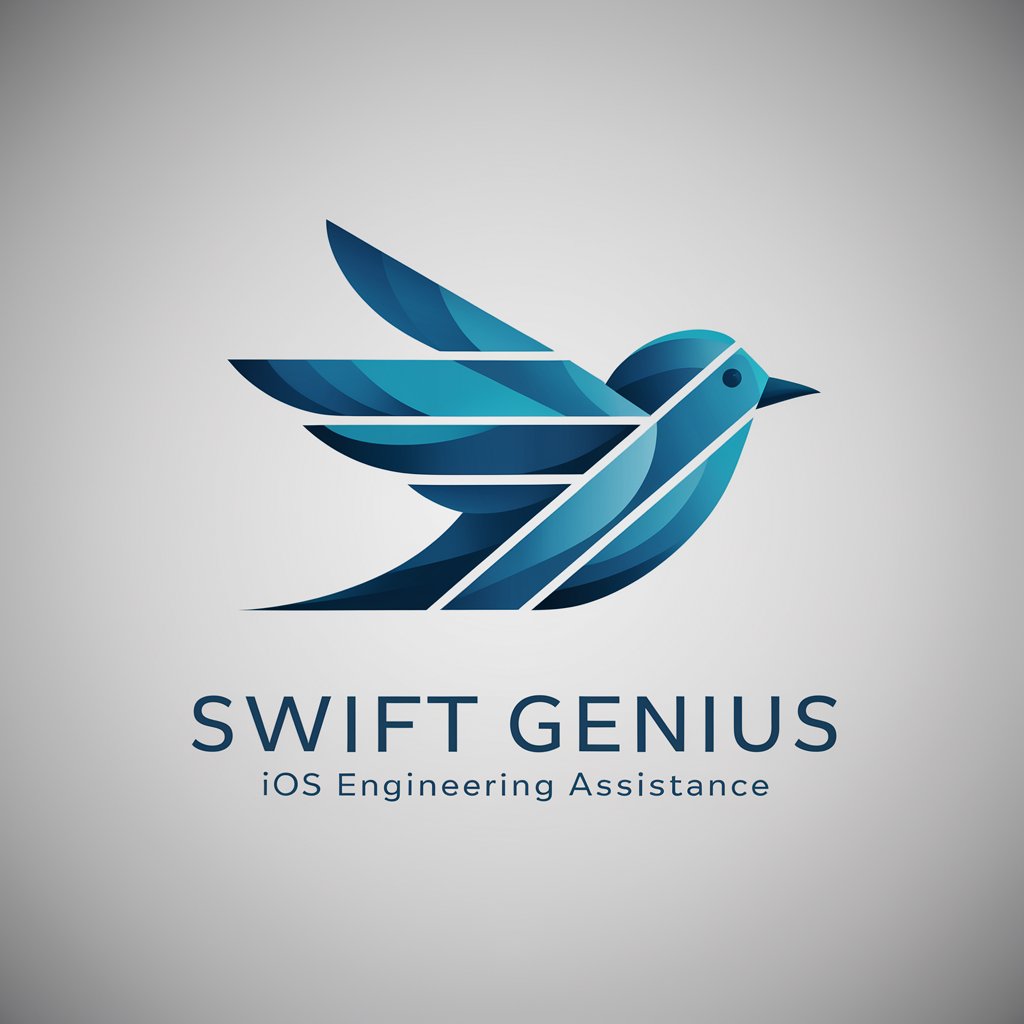
Swift Genius
Expert iOS Swift engineer for step-by-step app development.
20.0 / 5 (200 votes)
Understanding Swift Optionals: Mastering `nil` Handling
In Swift, optionals are a type that can hold either a value or no value (`nil`). This concept is crucial for managing the presence or absence of values safely within your code. Swift's design around optionals aims to improve code safety and clarity by forcing developers to explicitly deal with the possibility of `nil` values. This eliminates common errors in programming, such as null pointer exceptions. For example, when dealing with user input that might not provide a value for a user's age, an optional integer (`Int?`) can be used to represent this variable. This allows the program to compile only if it correctly handles cases where the age might be `nil`. Powered by ChatGPT-4o。
Core Functions of Swift Optionals
Optional Binding
Example
if let age = userAge { print(age) } else { print('Age is undefined.') }
Scenario
Used to safely unwrap an optional, executing the code block if the optional contains a value. Ideal for handling user input that may or may not be provided, ensuring the program can proceed safely with a guaranteed value.
Optional Chaining
Example
let roomCount = building?.apartments?.count
Scenario
Allows the program to attempt to access a property, method, or subscript on an optional that might currently be `nil`. This is particularly useful for querying nested optional properties without requiring multiple layers of unwrapping.
Guard Statement
Example
guard let age = userAge else { return }
Scenario
Enables early exit from a function or loop if an optional doesn’t contain a value. It’s often used at the beginning of a function to ensure all required data is available before proceeding, improving code readability and safety.
Nil Coalescing Operator
Example
let age = userAge ?? 18
Scenario
Provides a default value for an optional if it contains `nil`. This is useful for setting default states or values in the application, ensuring the program can operate with sensible defaults.
Target User Groups for Mastering Swift Optionals
Swift Beginners
Individuals new to Swift programming can benefit immensely from understanding optionals early on. Mastering `nil` handling is fundamental to writing safe, robust Swift code and avoiding common pitfalls associated with null values.
App Developers
Swift app developers, especially those dealing with user inputs, databases, or network requests, will find optionals indispensable. These scenarios frequently involve data that might be missing or undefined, requiring careful handling to maintain app stability and user experience.
Swift Educators
Educators teaching Swift programming need to convey the importance of optionals to students. Understanding how to safely handle `nil` values is critical for students to progress to more advanced Swift topics and build reliable applications.
Mastering Swift Optionals: Guidelines for Handling `nil`
Start your journey
Initiate your learning on handling `nil` in Swift by exploring yeschat.ai for a comprehensive, free trial without the need to sign in or subscribe to ChatGPT Plus.
Understand Swift Optionals
Learn the basics of optionals in Swift, including their definition, why they are used, and how they help manage the absence of a value safely.
Practice with examples
Engage with practical code examples demonstrating optional binding, optional chaining, and using guard statements to unwrap optionals safely.
Implement best practices
Adopt best practices for working with optionals, such as avoiding force unwrapping, leveraging the nil coalescing operator, and utilizing optional protocols.
Explore advanced concepts
Dive deeper into advanced optional handling techniques, including optional closures, error handling with optionals, and managing optional arrays and dictionaries.
Try other advanced and practical GPTs
Elevate Web UX: Javascript Insights & Innovations
AI-powered UX insights for JavaScript developers
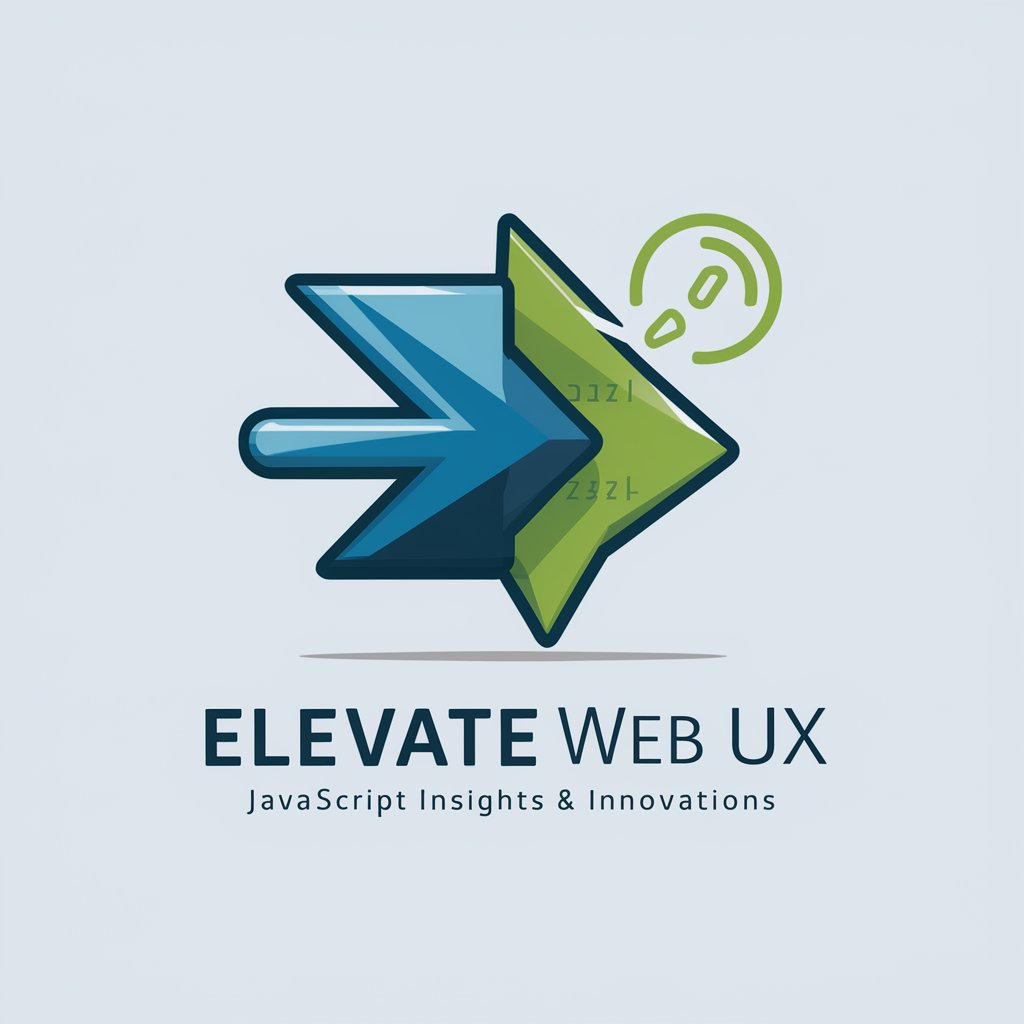
Lean UX - AI UX Coach - By Mo Goltz
Empowering UX design with AI insights.
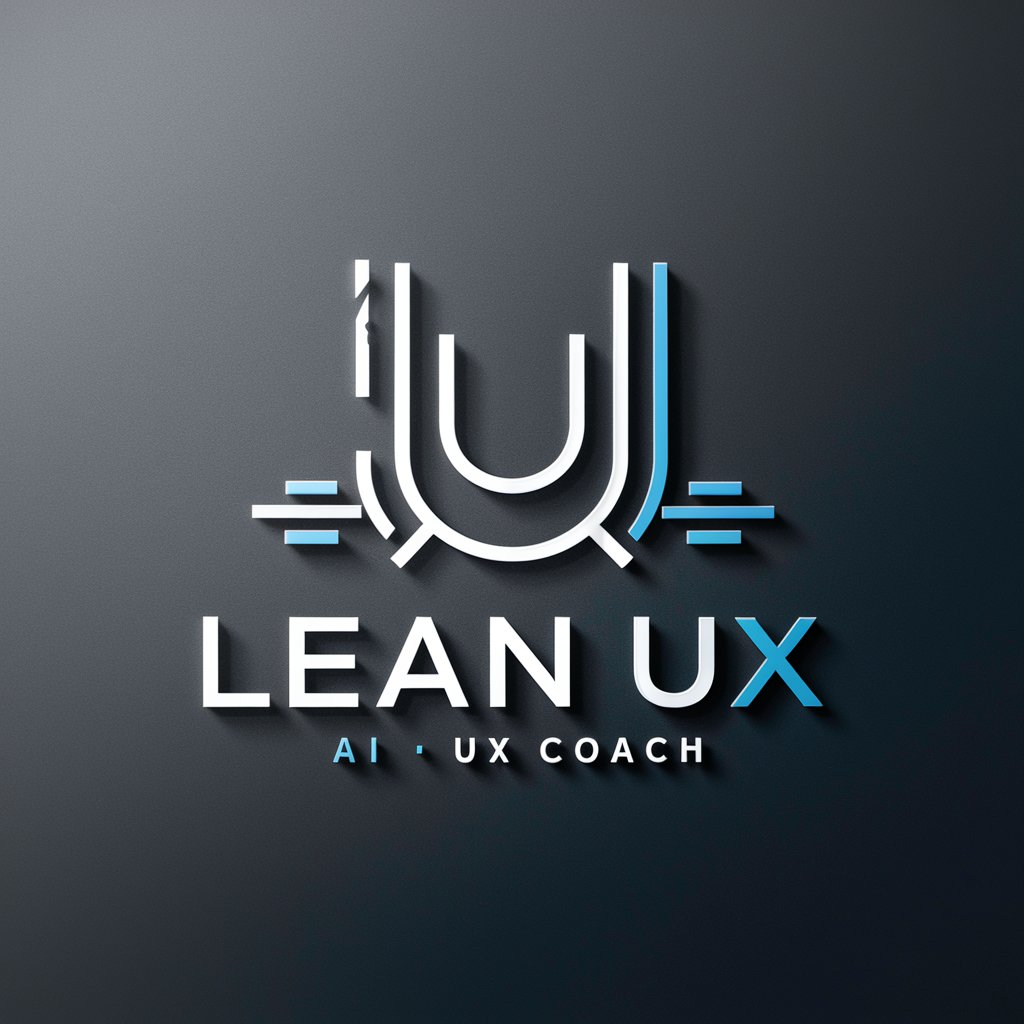
Estimation/Analytical/Execution Product Mock GPT
AI-driven insights for product estimation and metrics

Dr. Prognosis
Empowering health decisions with AI
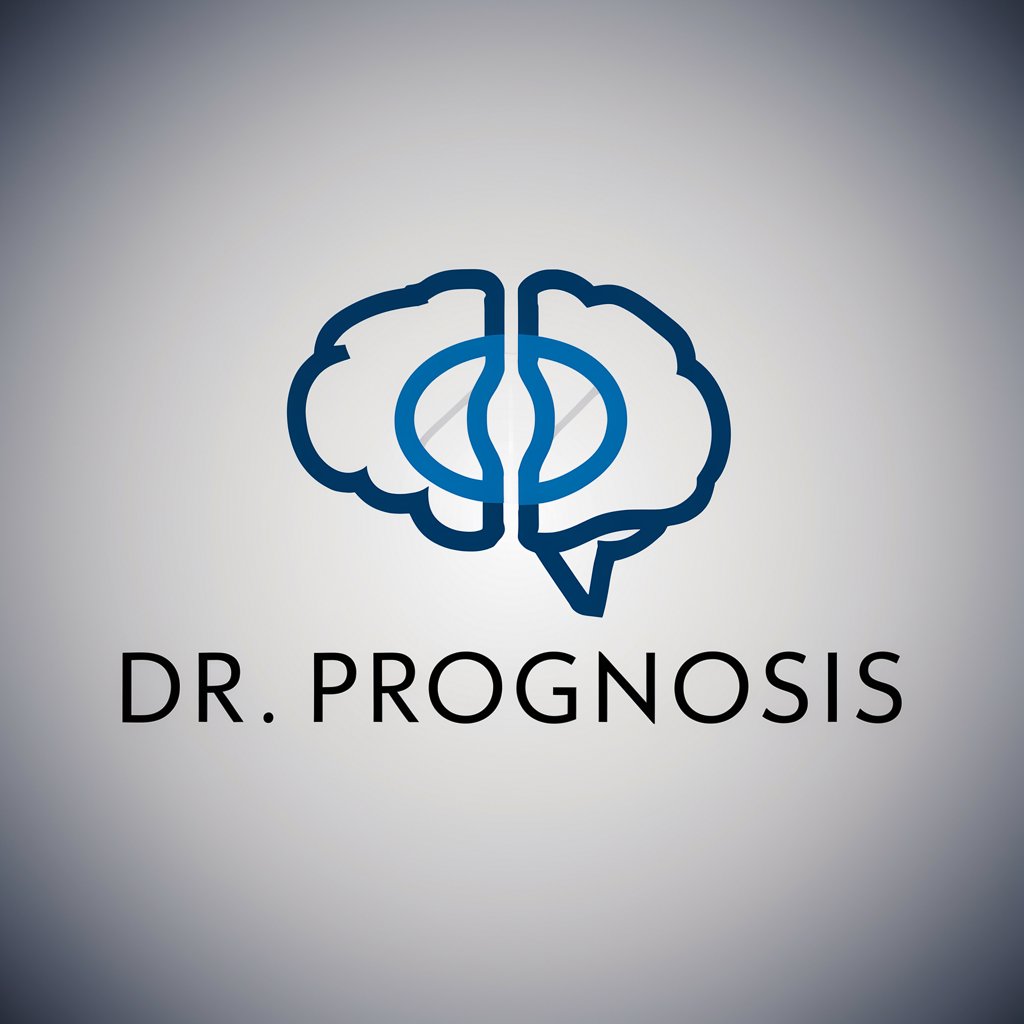
JavaScript Security: Ensuring Safe Web Apps
AI-powered Security for Web Apps
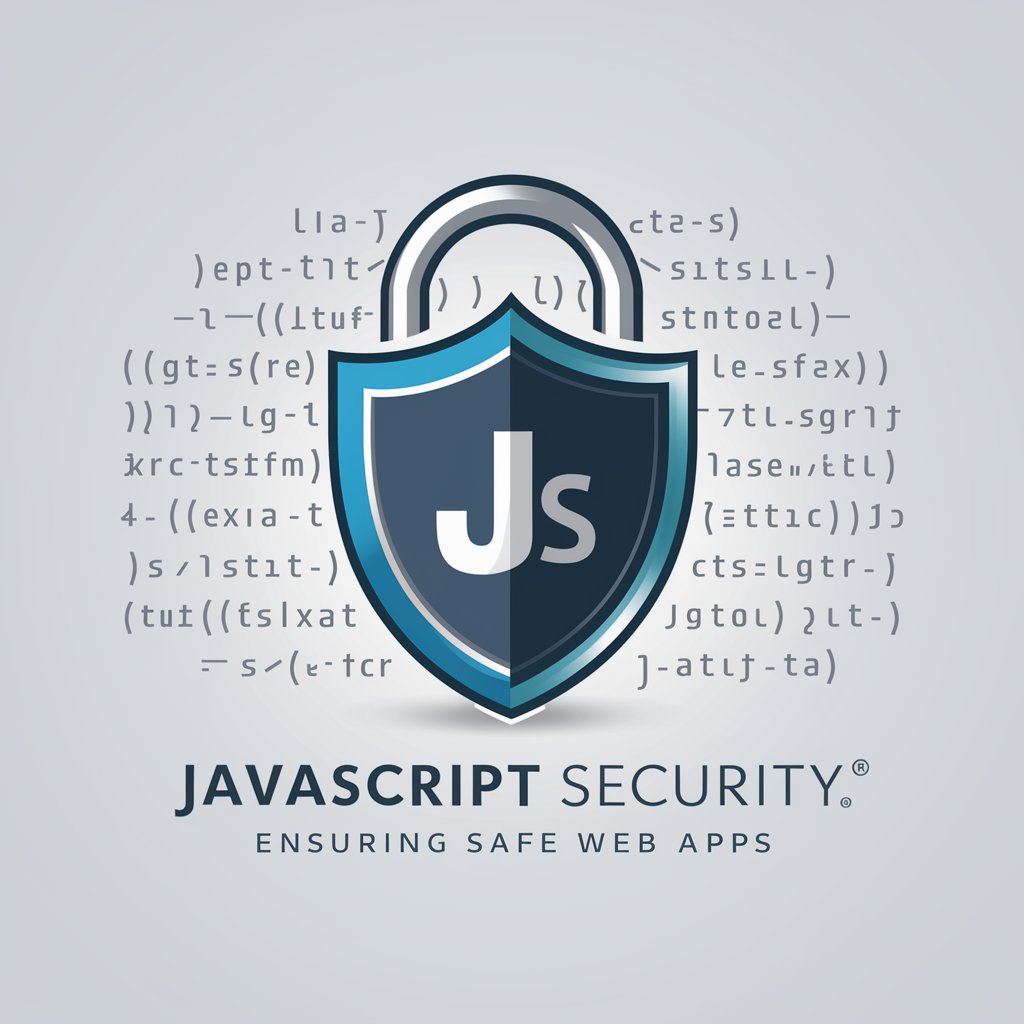
Coffee TalkGPT
Crafting Stories, Building Characters
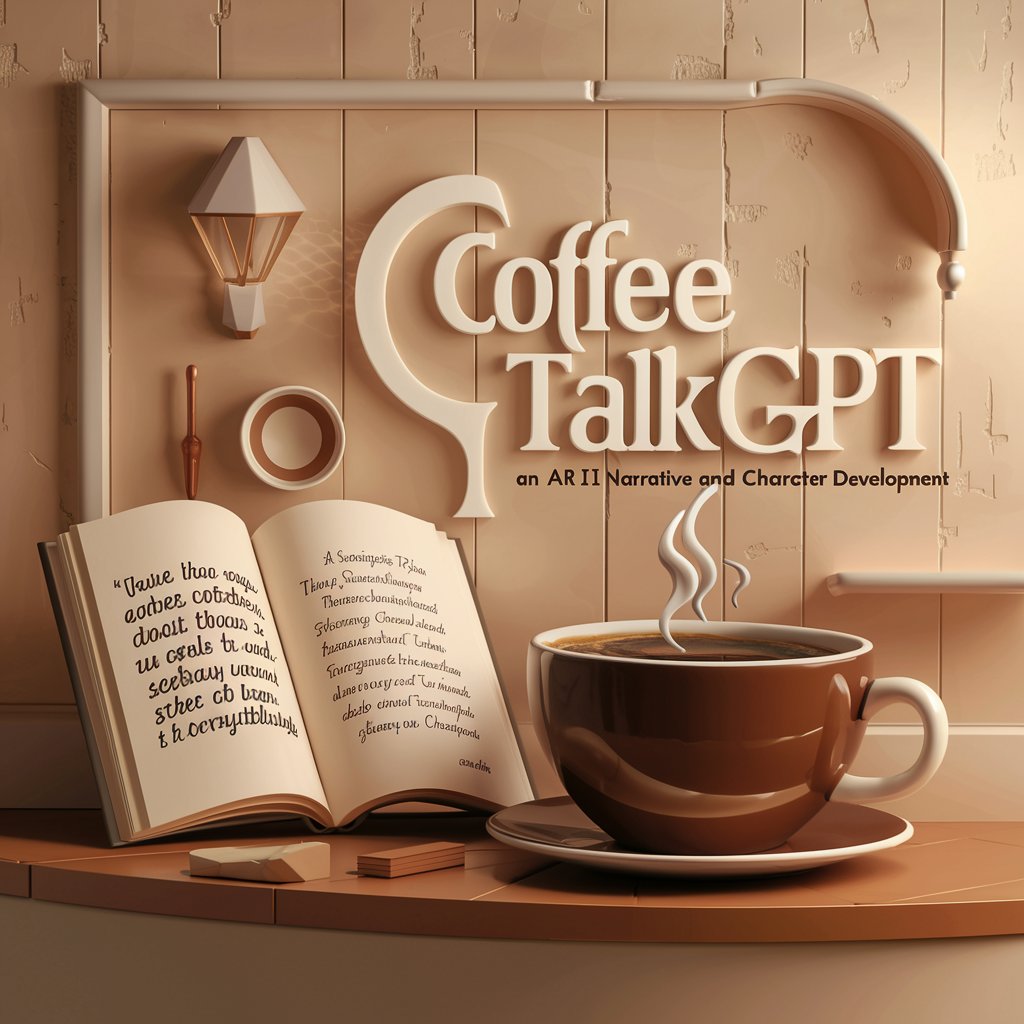
Life Guidance Online
Empowering Conscious Creation for Wellness

Rust: Mastering Concurrency with Async/Await
Unlocking Concurrency with AI-Powered Rust
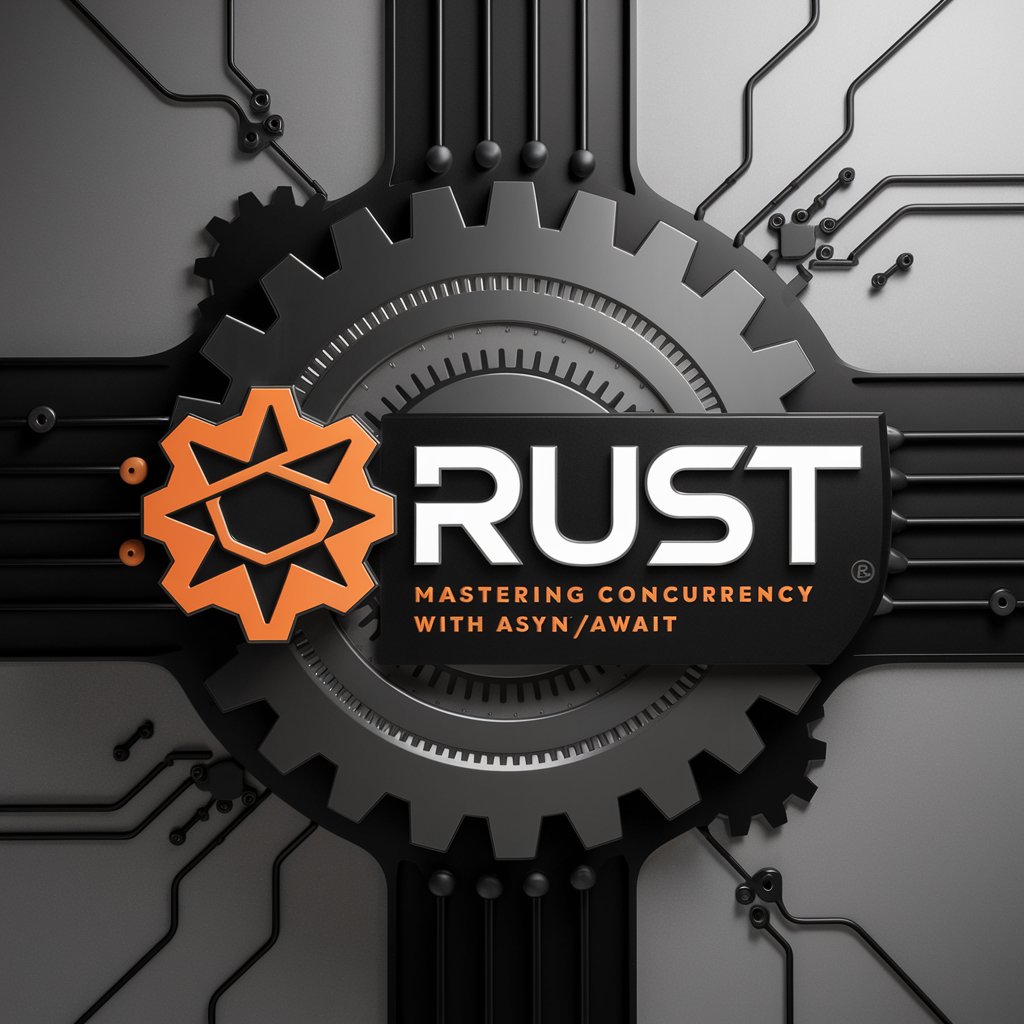
🚀 Mastering Flutter's Hot Reload
Instantly update Flutter apps with AI.
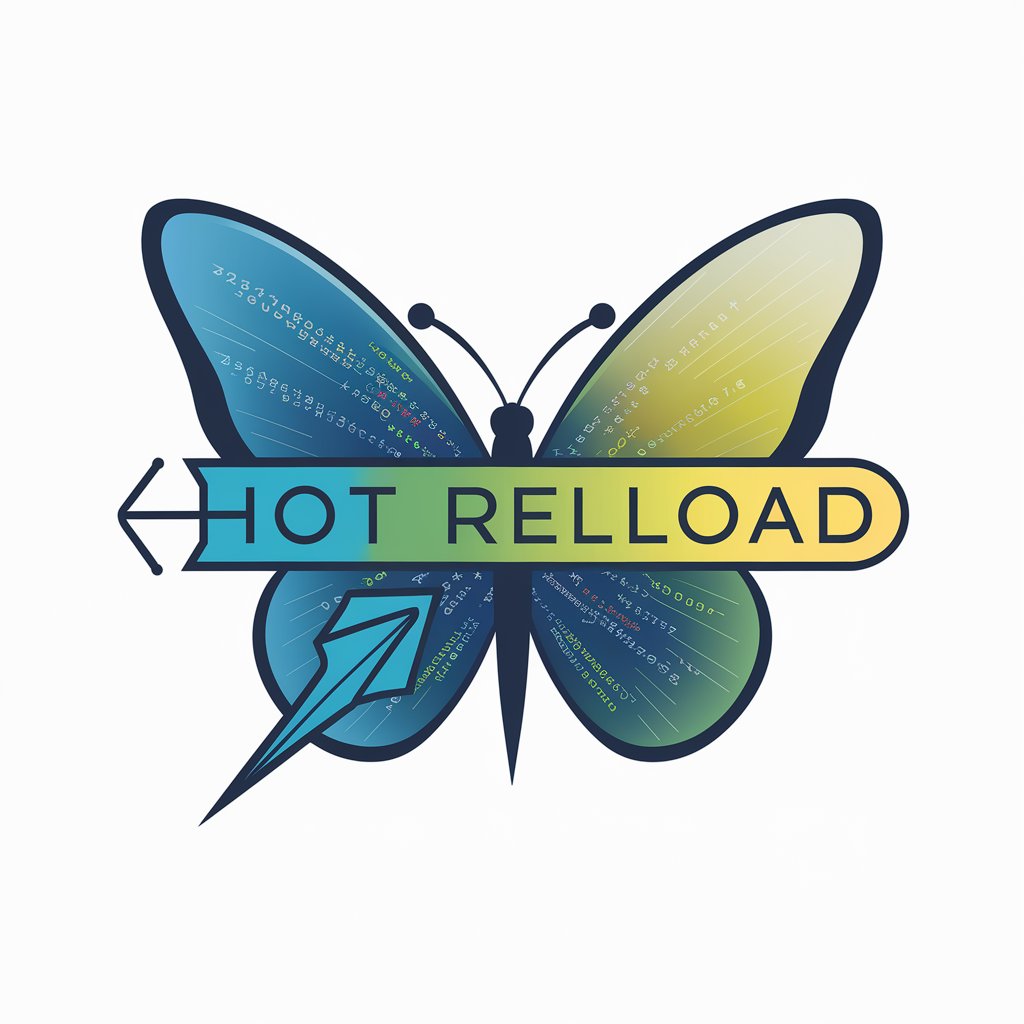
JavaScript Evolution: Refactor with ES6 Mastery
Transform your JavaScript with AI-powered ES6 Mastery
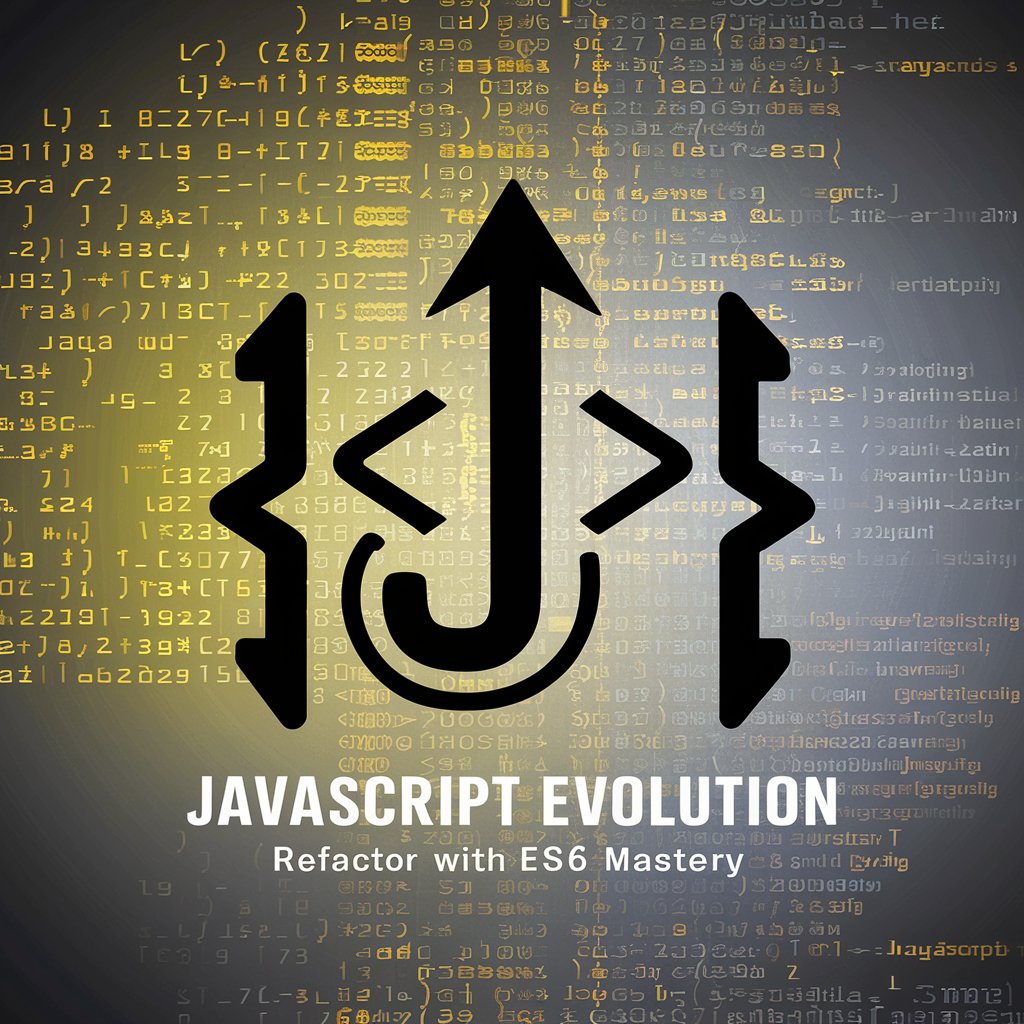
City Ranker
Empower Your City Choices with AI
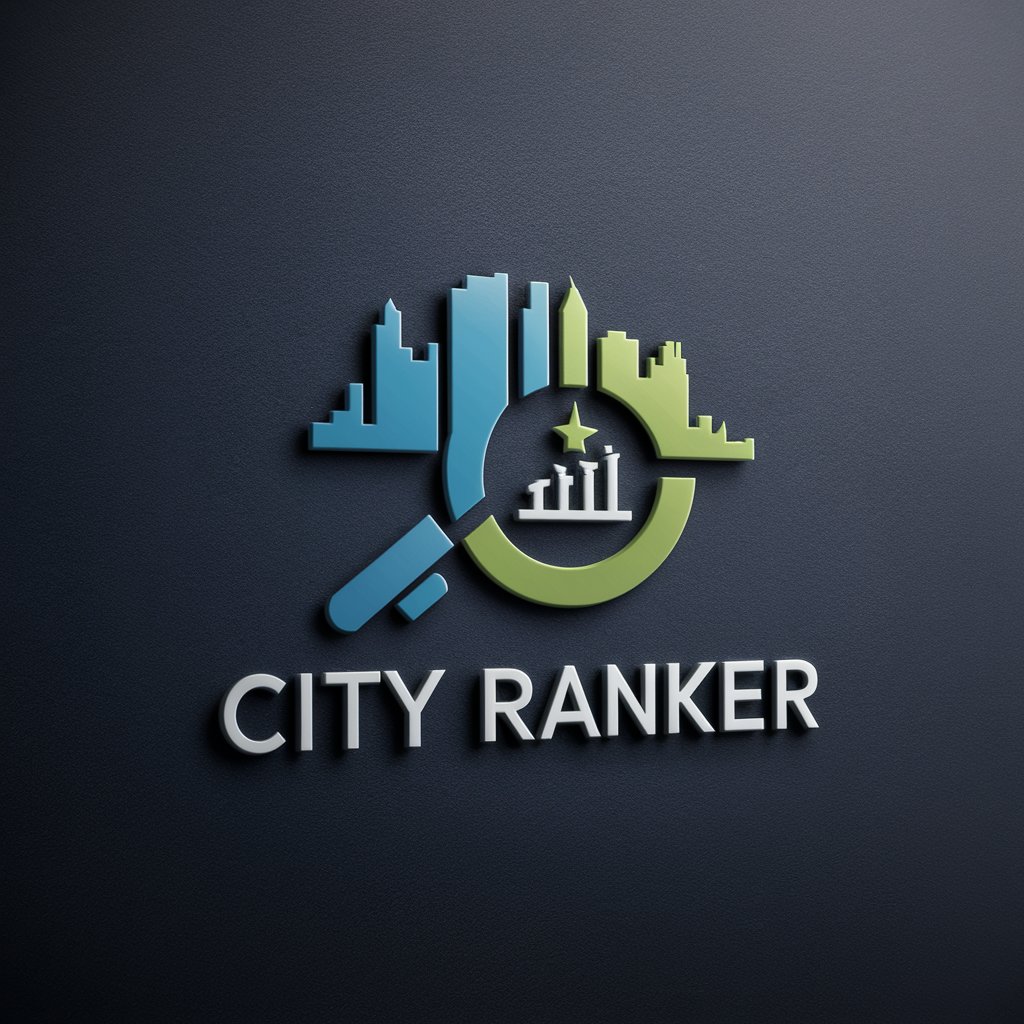
Personal Branding Writing Coach
Elevate Your Brand with AI-Powered Storytelling
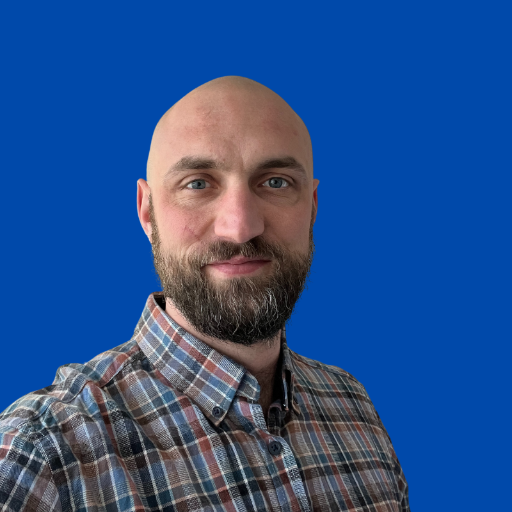
Swift Optionals Unpacked: Mastering `nil` Handling Q&A
What are Swift Optionals and why are they important?
Swift optionals are a type that can hold either a value or no value (`nil`). They are crucial for handling cases where data might be missing, allowing developers to write safer, more robust code by explicitly handling the absence of values.
How can I safely unwrap an optional in Swift?
You can safely unwrap an optional using optional binding (`if let` or `guard let`) to check for and extract a value only if one exists, or using optional chaining to call properties, methods, and subscripts on an optional that might currently be `nil`.
What is the nil coalescing operator and how do I use it?
The nil coalescing operator (`??`) provides a default value for an optional if it contains `nil`. It's used to simplify code when you need to unwrap an optional and provide a fallback value, like `let value = optional ?? defaultValue`.
Can you give an example of using a guard statement with optionals?
Yes, `guard let` is used to unwrap an optional and exit early if `nil` is encountered. For example, `guard let unwrappedValue = optionalValue else { return }` unwraps `optionalValue` or exits the function if it's `nil`.
How do I handle optional arrays or dictionaries?
Optional collections can be managed using the same techniques as other optionals. For example, you can use optional binding to safely access elements and optional chaining to call methods on the collection if it's not `nil`.