👨💻 C++ Compilation with g++ - C++ Compiler Guide
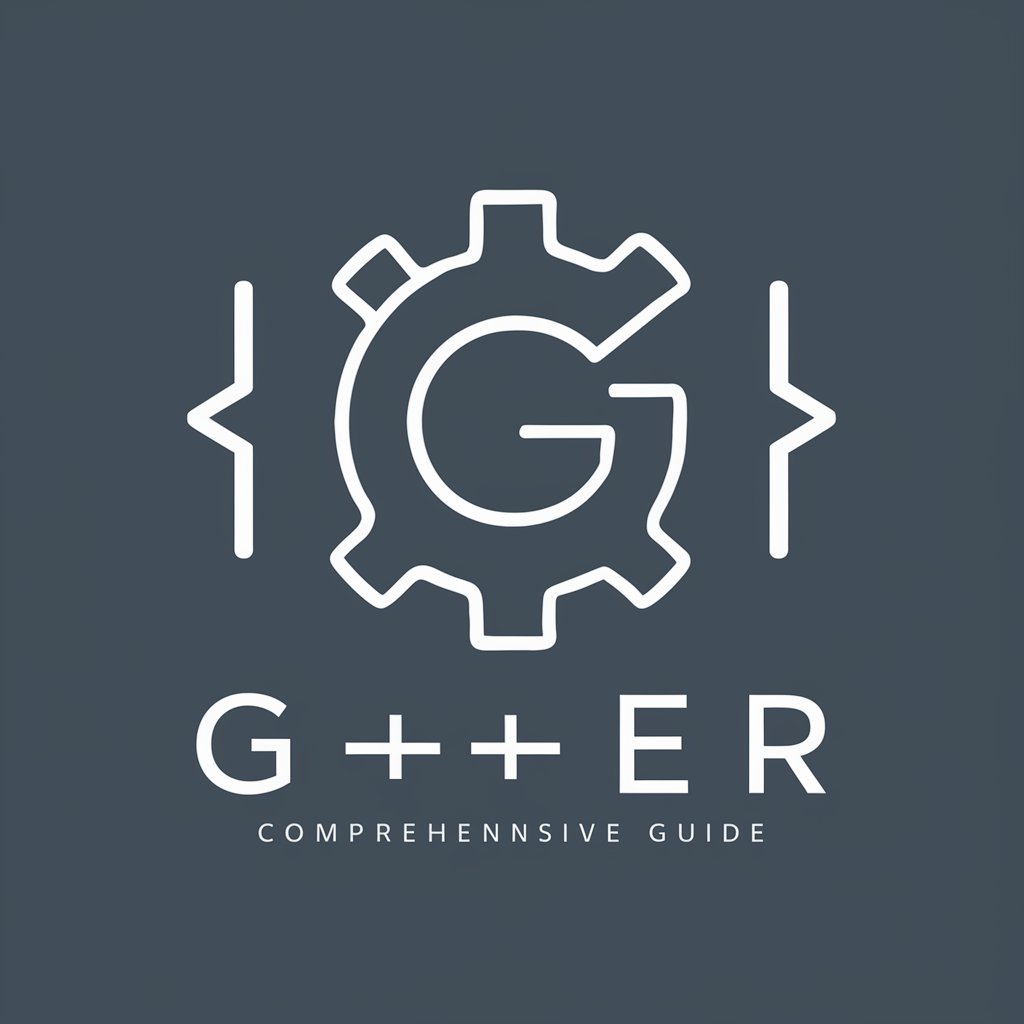
Welcome to the ultimate C++ compilation guide with g++.
Effortless C++ compilation with AI insights.
Design a C++ compilation guide logo featuring...
Create a modern logo that illustrates the g++ compiler...
Generate a technical and sleek logo for a C++ tutorial...
Illustrate a logo that combines elements of coding and compilation...
Get Embed Code
Introduction to C++ Compilation with g++
The g++ compiler is a part of the GNU Compiler Collection (GCC) designed specifically for compiling C++ programs. It processes C++ source code, executing several stages of compilation: preprocessing, compiling, assembling, and linking. The preprocessing phase involves macro expansion and file inclusion. The compiling phase translates C++ code into assembly language. Assembling converts assembly language into machine code, and linking combines these machine codes into a single executable program. g++ is known for its versatility, supporting various standards of C++ through different compiler flags, and allowing fine control over the compilation process. An example scenario where g++ is crucial involves a developer working on a multi-file C++ project that uses third-party libraries. The developer would use g++ to compile each source file, specify include paths for the libraries, and link the object files into a single executable, ensuring that all dependencies are correctly resolved. Powered by ChatGPT-4o。
Main Functions of C++ Compilation with g++
Compilation of single and multiple source files
Example
g++ main.cpp -o main
Scenario
A developer wants to compile a single-source file project. Using g++, they can compile and link the source file in one command, producing an executable.
Linking with external libraries
Example
g++ main.cpp -lmath -o main
Scenario
When a project requires a third-party library, such as a math library, g++ can link the compiled object files against the library to resolve external references.
Optimization and warning flags
Example
g++ -O2 -Wall main.cpp -o main
Scenario
For a project aimed at production, the developer might use optimization flags (e.g., -O2 for speed optimization) and warning flags (e.g., -Wall to enable all compiler warnings) to ensure code efficiency and correctness.
Defining macros
Example
g++ -DDEBUG main.cpp -o main
Scenario
In a scenario where conditional compilation is required, such as including debug information in a build, g++ allows the definition of macros via command line, which can alter compilation.
Specifying include directories
Example
g++ -I/include/path main.cpp -o main
Scenario
When a project depends on headers located in non-standard directories, g++ can be instructed to look in those directories for header files, ensuring the compiler finds all necessary files.
Ideal Users of C++ Compilation with g++
Software Developers
Professionals and hobbyists developing software in C++ are primary users. They benefit from g++'s comprehensive support for C++ standards, its ability to manage complex projects with multiple dependencies, and its flexibility in optimization and debugging.
Educators and Students
Educators teaching C++ programming and students learning the language can use g++ as a tool to compile and execute code examples and assignments. Its widespread availability and compatibility with various C++ standards make it an ideal educational tool.
Open Source Contributors
Contributors to open-source C++ projects often use g++, as it is free and supports cross-platform compilation. This makes it easier to ensure that contributions are compatible with different environments and build systems.
Getting Started with C++ Compilation Using g++
Start Your Journey
Initiate your coding adventure by exploring yeschat.ai for an unrestricted trial, where signing up or ChatGPT Plus is not a requirement.
Install g++
Ensure g++ is installed on your system. For Linux users, this typically involves using a package manager like apt or yum. Windows users might prefer MinGW.
Prepare Your Code
Organize your C++ source files. If you're working on a larger project, consider separating your code into multiple files for easier management.
Compile Your Code
Use the command `g++ -o outputName source.cpp` to compile a single file, replacing `outputName` with your desired executable name and `source.cpp` with your source file.
Advanced Compilation
Explore compiler flags for optimization (`-O2`), warnings (`-Wall`), and debugging (`-g`). These can enhance performance and assist in debugging.
Try other advanced and practical GPTs
Middle Ground
Discover Unseen Connections, Powered by AI
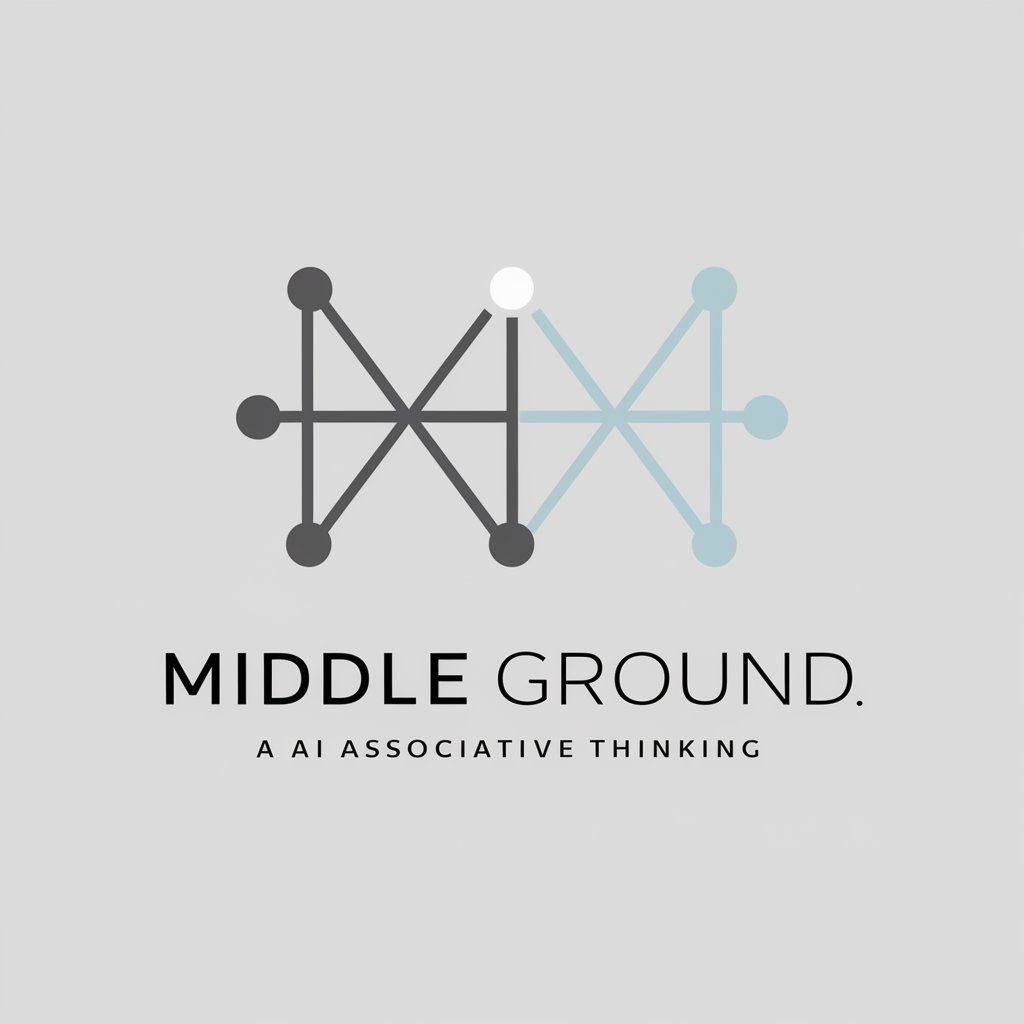
The Symbiosis of AI and Human Intuition
Empowering Decisions with AI-Driven Intuition
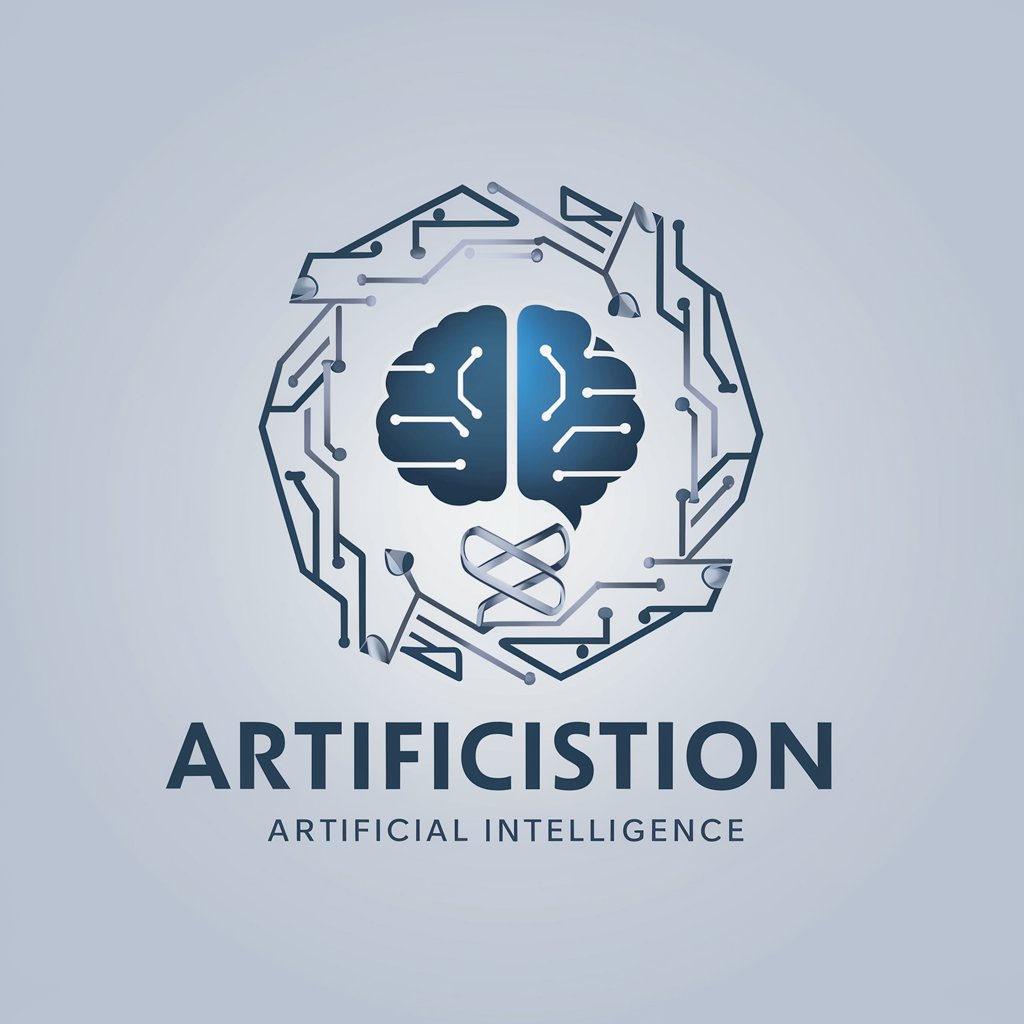
Party Talk Creator
Crafting Authentic In-game Conversations
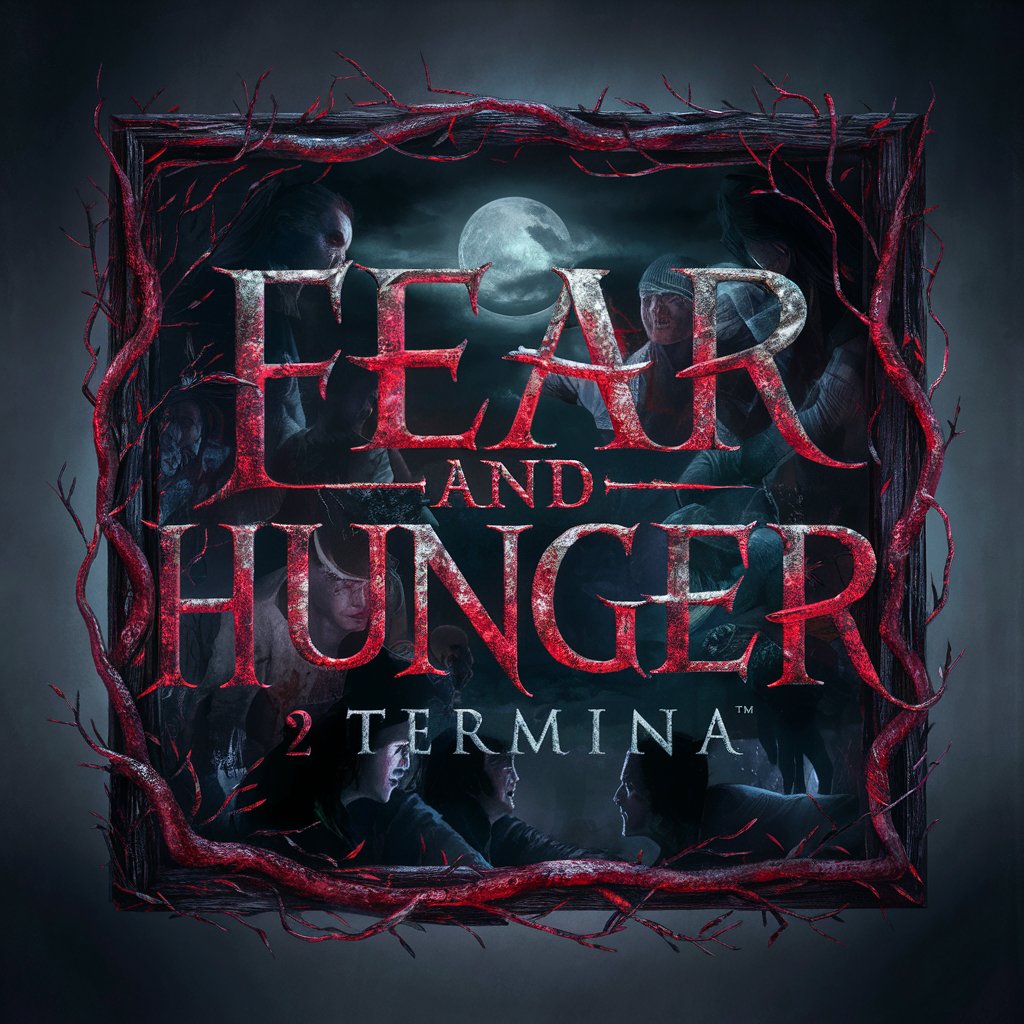
Anxiety Ease Wizard - AI
Transform anxiety into calmness with AI-powered empathy.
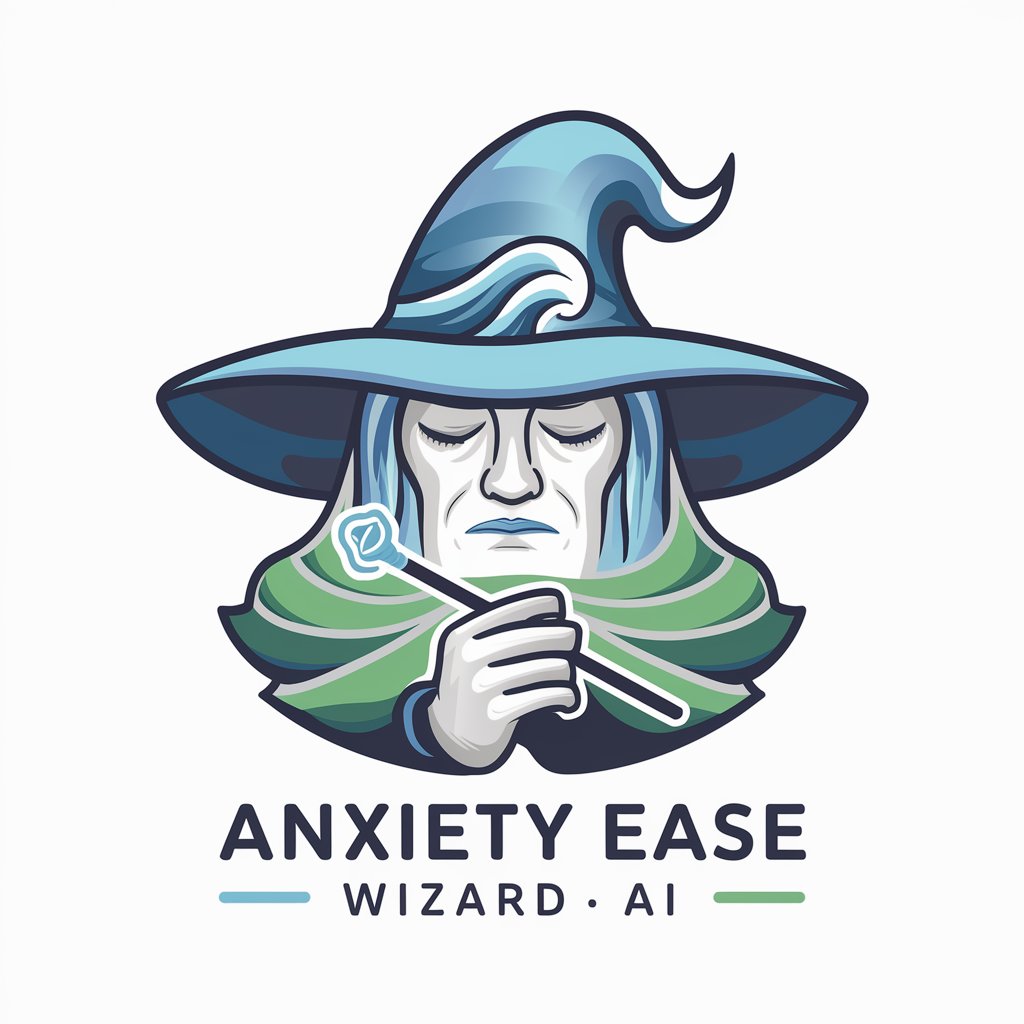
Culinary Creator Vege Friendly
Crafting Vegetarian Delights with AI
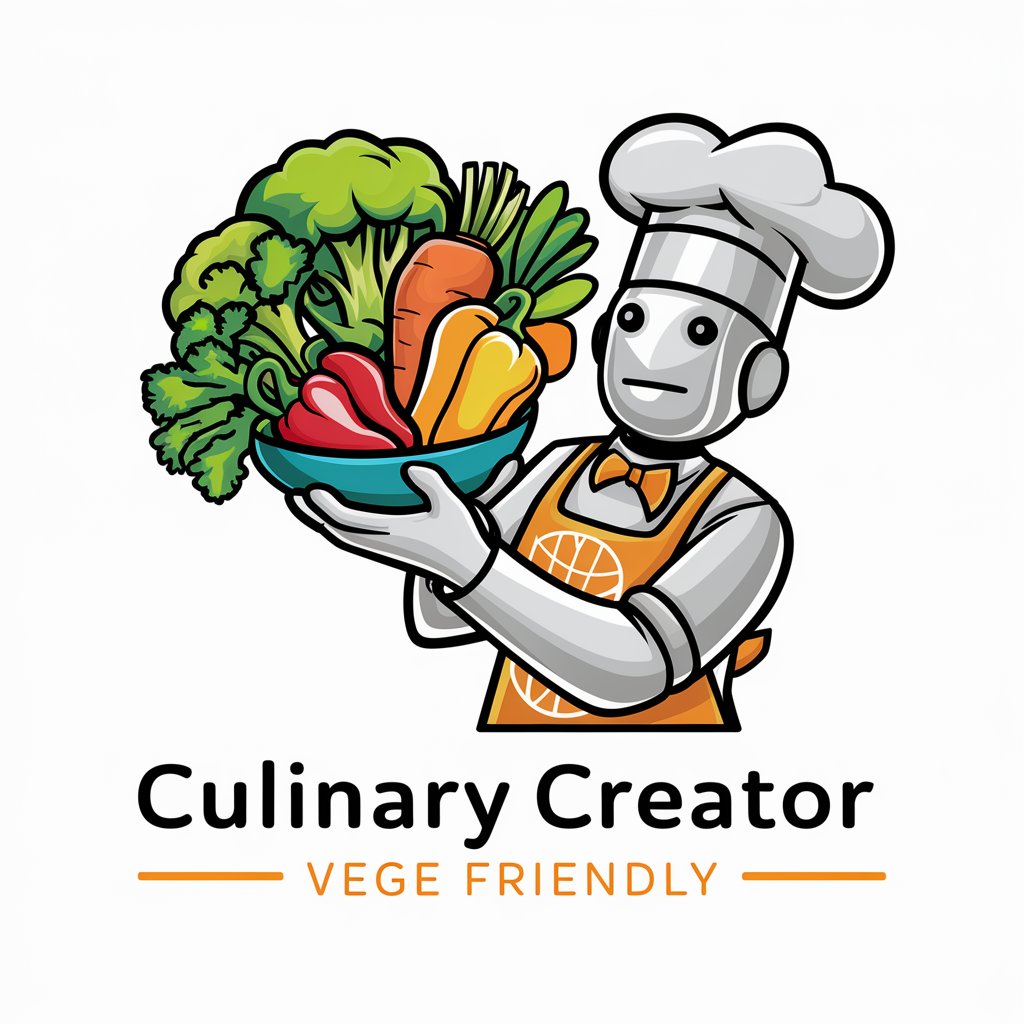
Go Context: Timeout & Cancellation Expertise
Streamline Go timeouts and cancellations.
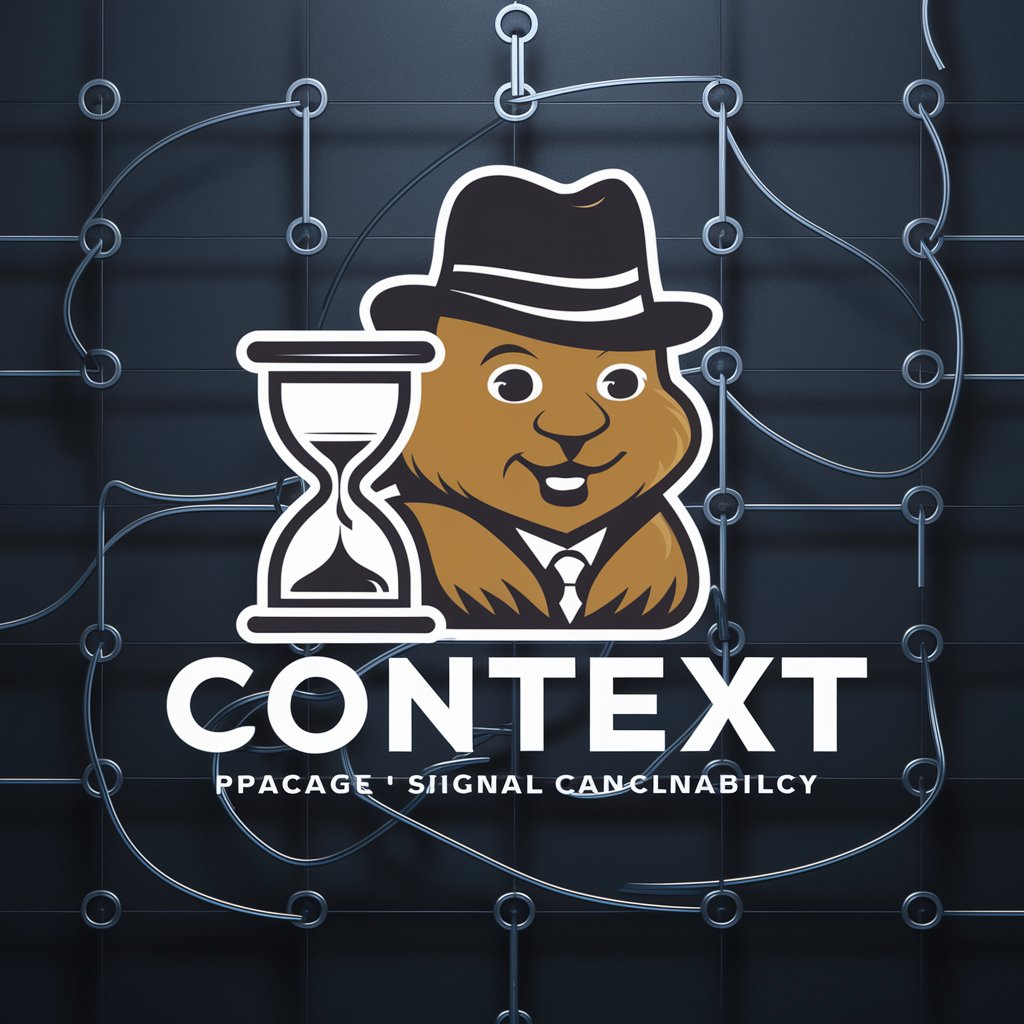
Prompt Genius
Elevate ChatGPT with Tailored Prompts
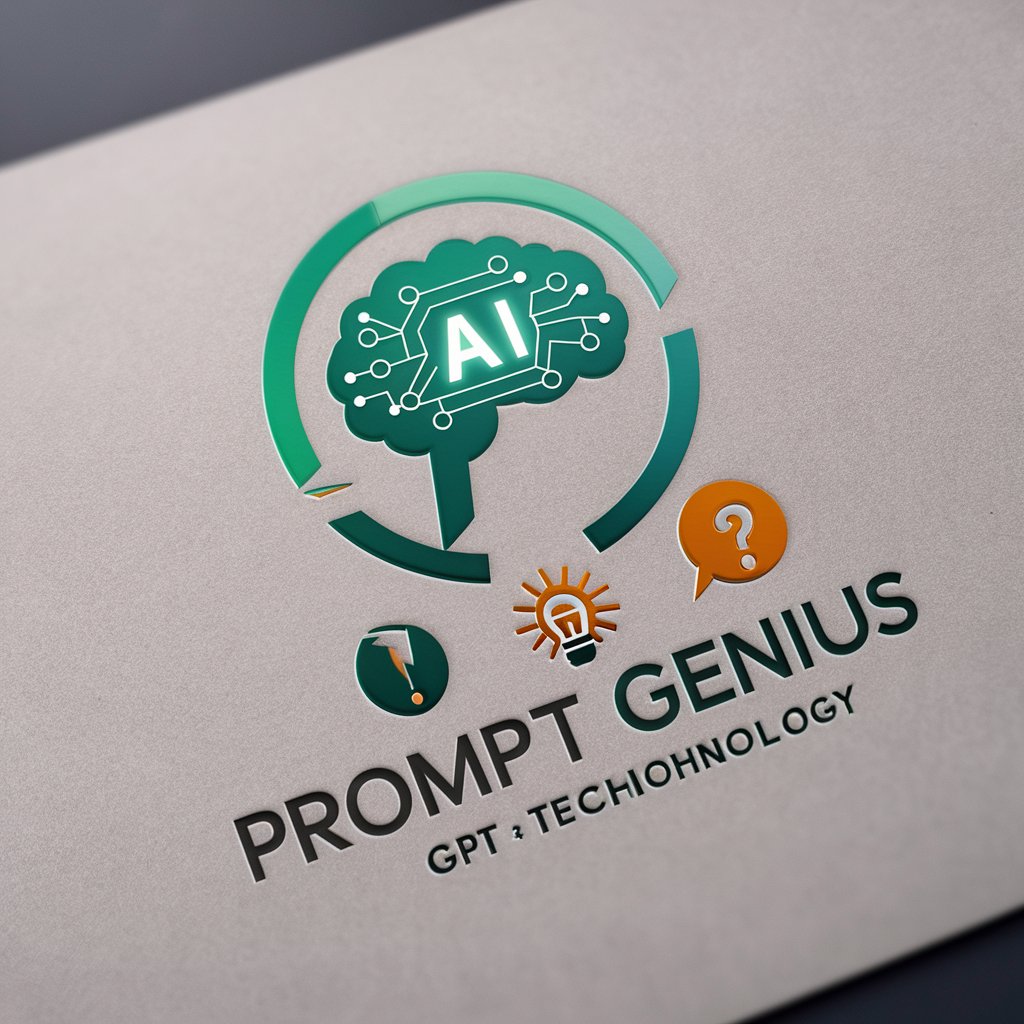
Crafting Compact IoT Solutions with C
Crafting efficient IoT solutions with AI-powered C programming insights.
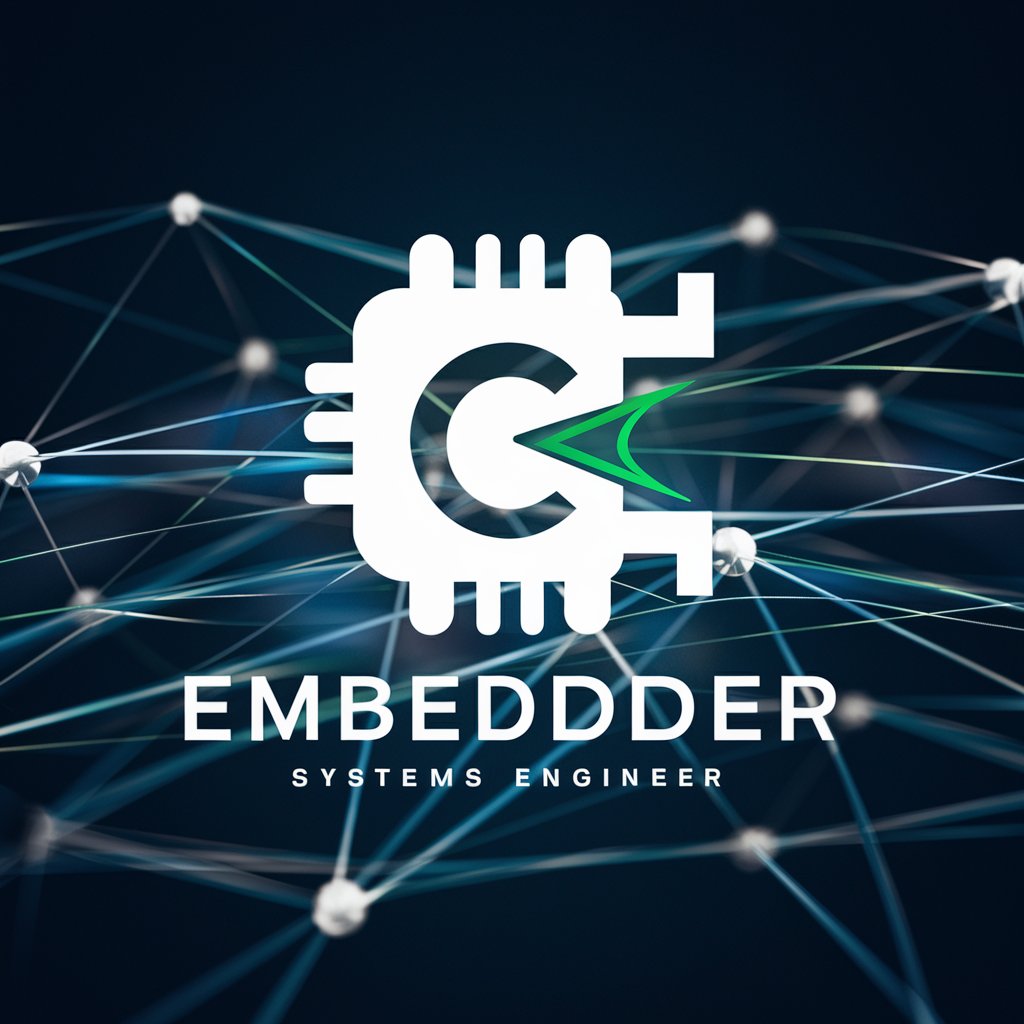
C# Code Conquest
Empower your C# journey with AI
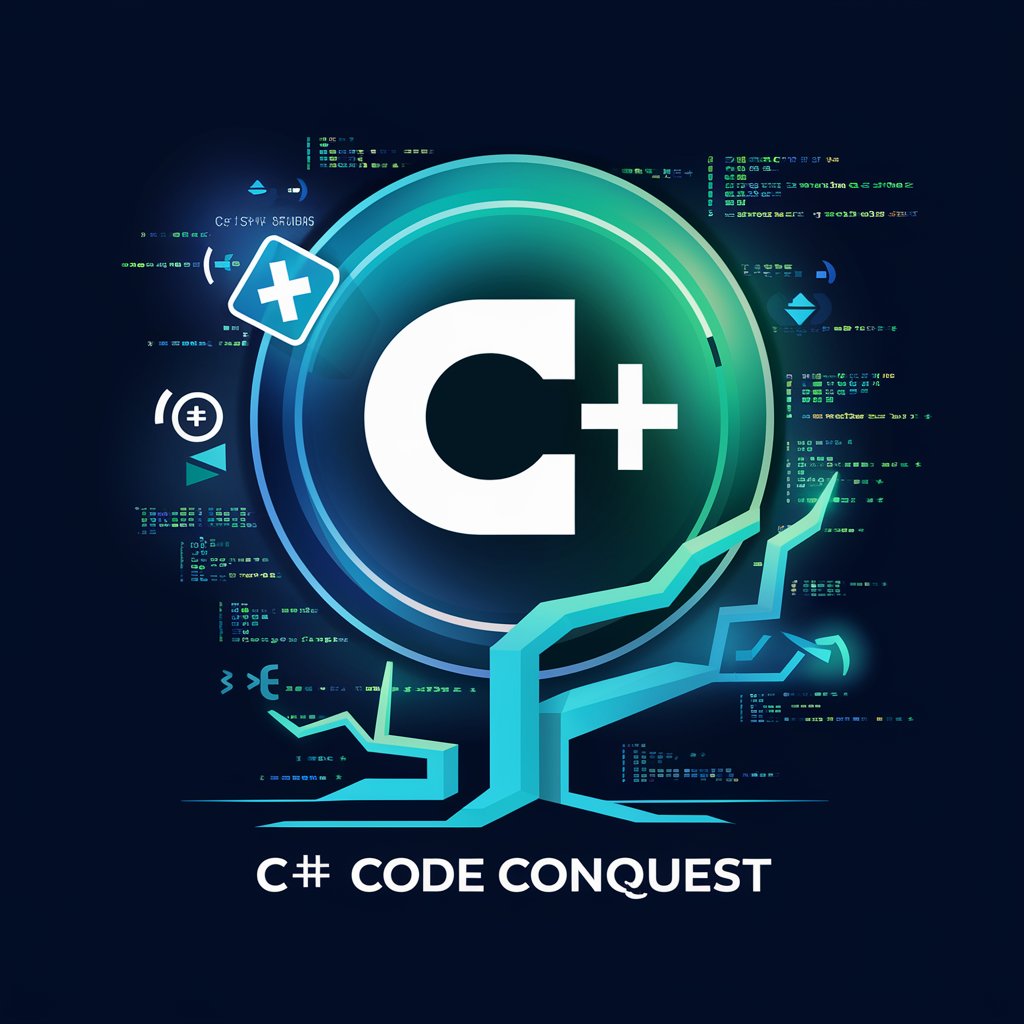
Uplifting Sidekick
Empathetic AI for Emotional Well-being
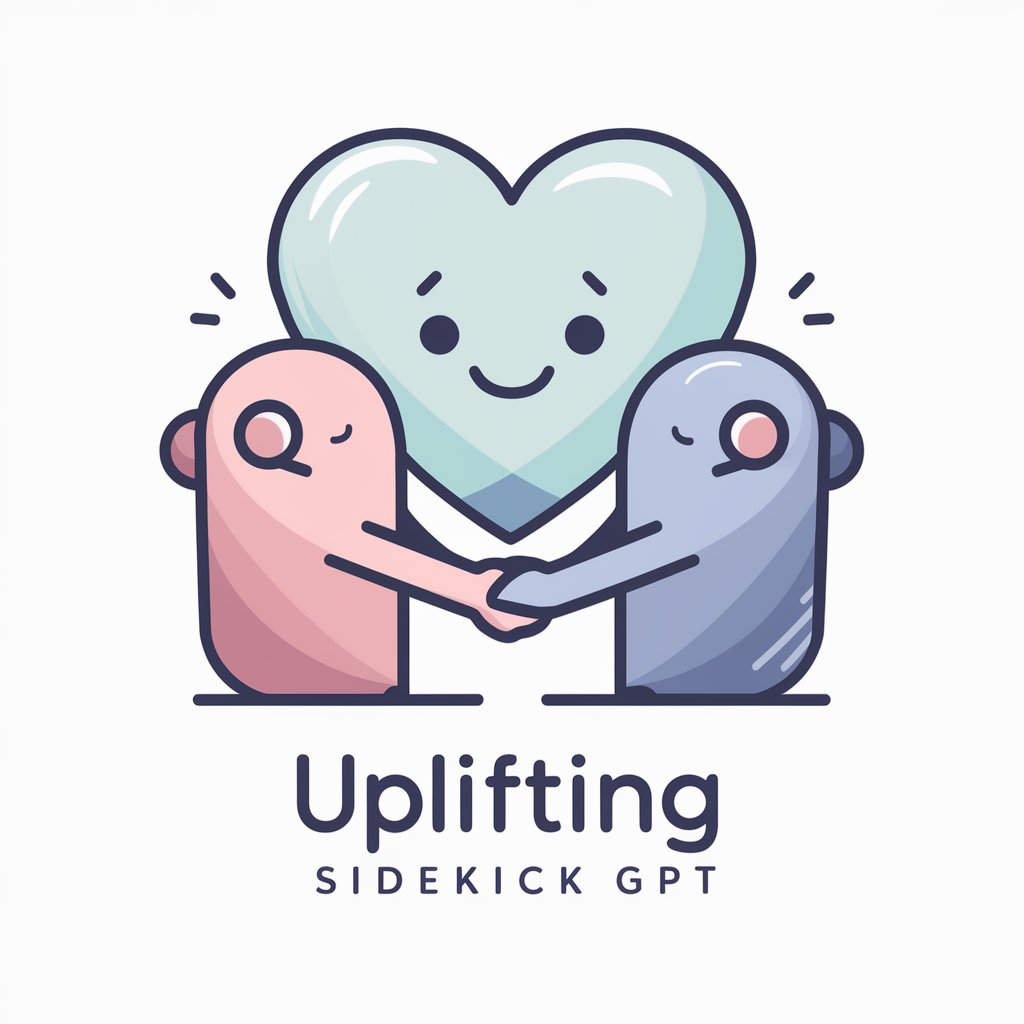
JavaScript Cross-Browser Harmony
Harmonize web experiences across browsers with AI.
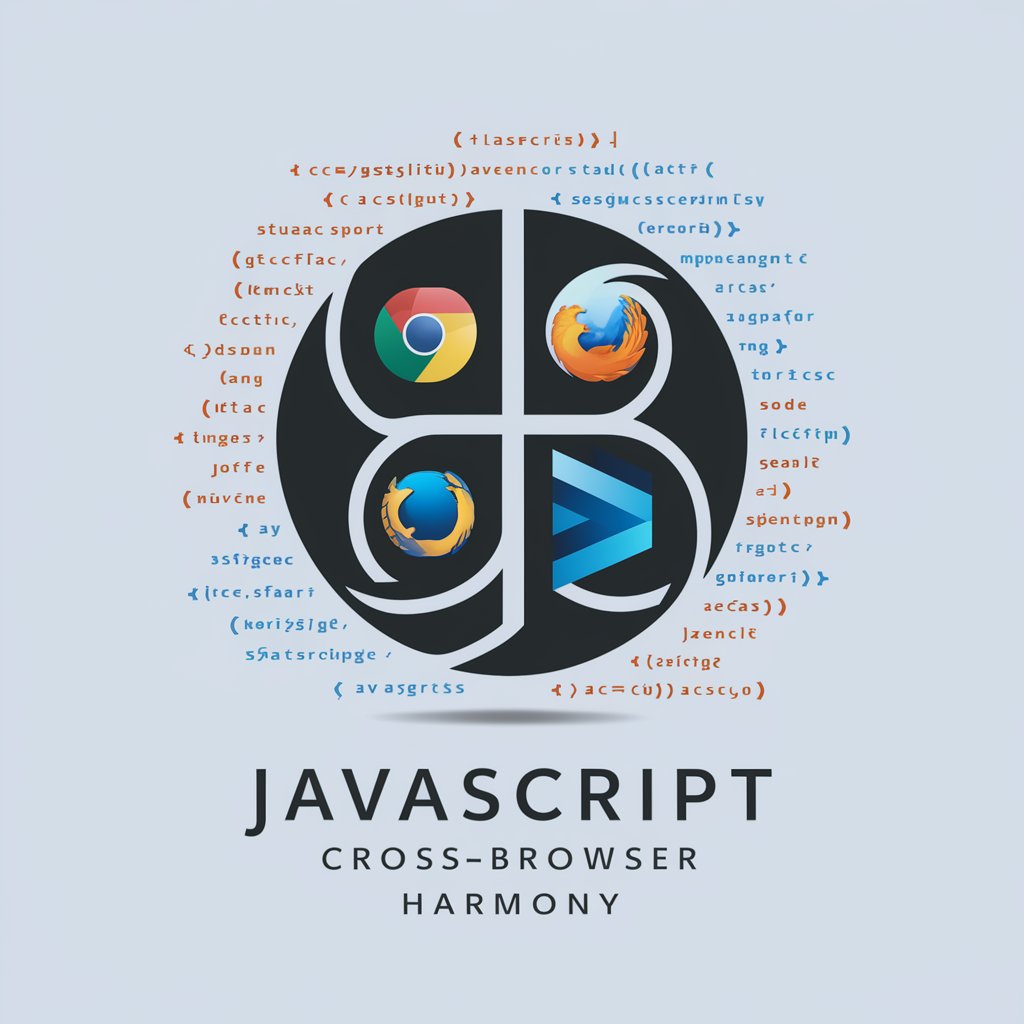
EYE SEE THEM
Unleash Your Creative Potential with AI
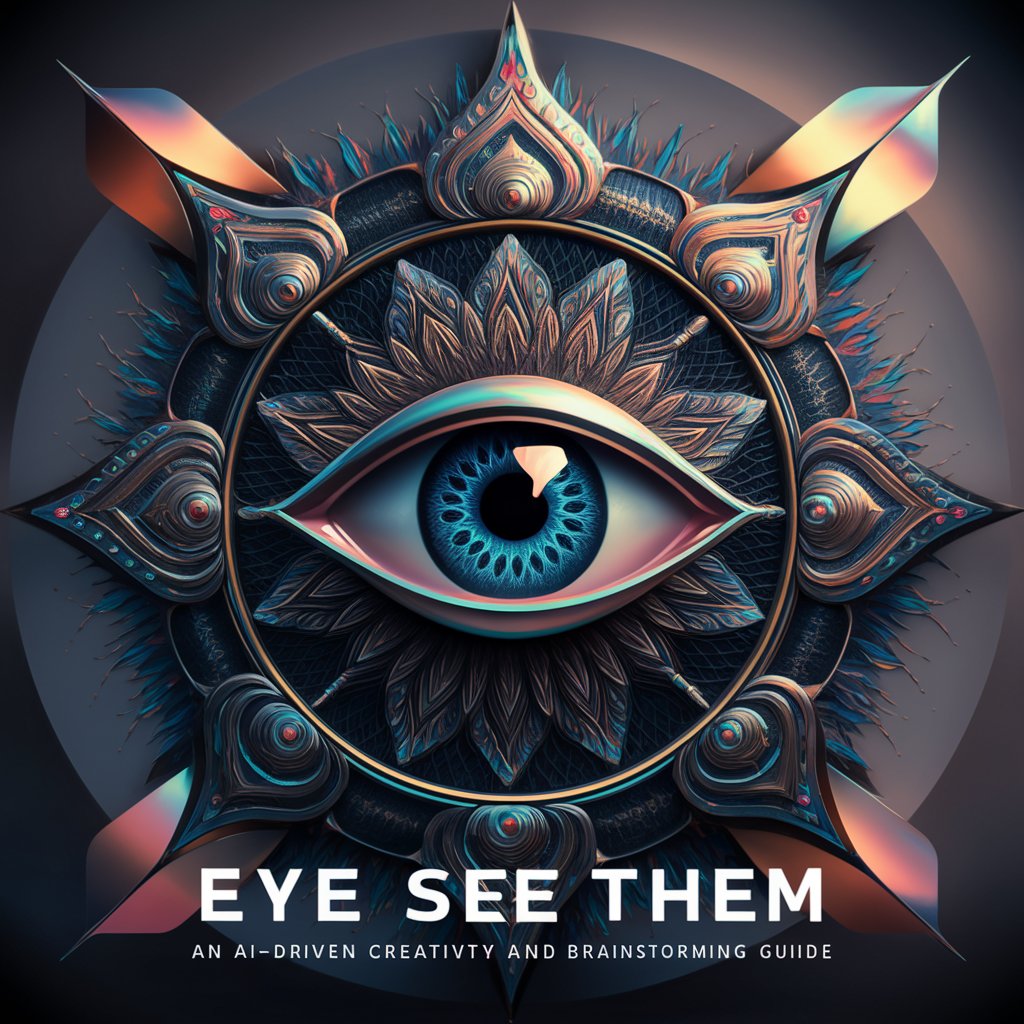
FAQs on C++ Compilation with g++
What is g++ and its role in C++ compilation?
g++ is part of the GNU Compiler Collection (GCC) and is specifically designed for compiling C++ programs. It handles preprocessing, compiling, assembling, and linking stages of the compilation process.
How can I manage multiple source files in my project?
Compile each source file separately into object files using `g++ -c`, then link them together in the final step with `g++ -o outputName file1.o file2.o`.
What are some common compiler flags and their uses?
Common flags include `-Wall` for comprehensive warnings, `-O2` for optimization, and `-g` for generating debug information. These help identify potential issues and improve the performance and debuggability of your application.
How do I include libraries in my compilation process?
Use the `-l` flag to link against a library and `-L` to add directories to the library search path. Include header files with `-I` to specify directories for the compiler to look for included files.
What are the best practices for debugging a C++ program compiled with g++?
Compile your program with the `-g` flag to include debugging information. Use tools like gdb for debugging. Pay attention to compiler warnings as they can often point to potential errors.